如何在 WinForms 应用程序中有效地循环访问多个面板上的文本框?
在 Winforms 应用程序中循环遍历文本框
在 Winforms 应用程序中,您可能会遇到需要遍历文本框集合的情况在屏幕上。每个文本框都可以按顺序编号,例如:
DateTextBox0 DateTextBox1 ... DateTextBox37
要为这些文本框分配值,您可以考虑以下方法:
int month = MonthYearPicker.Value.Month; int year = MonthYearPicker.Value.Year; int numberOfDays = DateTime.DaysInMonth(year, month); m_MonthStartDate = new DateTime(year, month, 1); m_MonthEndDate = new DateTime(year, month, numberOfDays); DayOfWeek monthStartDayOfWeek = m_MonthStartDate.DayOfWeek; int daysOffset = Math.Abs(DayOfWeek.Sunday - monthStartDayOfWeek); for (int i = 0; i <= (numberOfDays - 1); i++) { // Here is where you want to loop through the textboxes and assign values based on the 'i' value // DateTextBox(daysOffset + i) = m_MonthStartDate.AddDays(i).Day.ToString(); }
但是,您的应用程序引入了一个由于这些文本框位于单独的面板上,因此增加了额外的复杂性。要有效地循环这些控件,您可以使用扩展方法:
public static IEnumerable<TControl> GetChildControls<TControl>(this Control control) where TControl : Control { var children = (control.Controls != null) ? control.Controls.OfType<TControl>() : Enumerable.Empty<TControl>(); return children.SelectMany(c => GetChildControls<TControl>(c)).Concat(children); }
使用此扩展方法,您可以递归检索指定类型的所有控件和子控件。使用示例:
var allTextBoxes = this.GetChildControls<TextBox>(); foreach (TextBox tb in allTextBoxes) { tb.Text = ...; }
通过使用此扩展方法,您可以有效地循环遍历单独面板上的所有文本框,并根据您想要的逻辑为其赋值。
以上是如何在 WinForms 应用程序中有效地循环访问多个面板上的文本框?的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
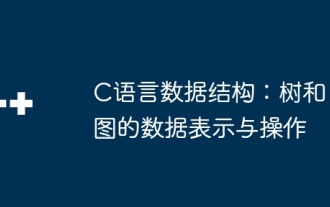
C语言数据结构:树和图的数据表示与操作树是一个层次结构的数据结构由节点组成,每个节点包含一个数据元素和指向其子节点的指针二叉树是一种特殊类型的树,其中每个节点最多有两个子节点数据表示structTreeNode{intdata;structTreeNode*left;structTreeNode*right;};操作创建树遍历树(先序、中序、后序)搜索树插入节点删除节点图是一个集合的数据结构,其中的元素是顶点,它们通过边连接在一起边可以是带权或无权的数据表示邻
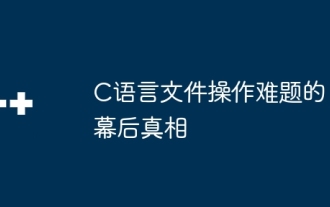
文件操作难题的真相:文件打开失败:权限不足、路径错误、文件被占用。数据写入失败:缓冲区已满、文件不可写、磁盘空间不足。其他常见问题:文件遍历缓慢、文本文件编码不正确、二进制文件读取错误。
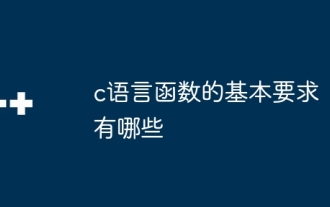
C语言函数是代码模块化和程序搭建的基础。它们由声明(函数头)和定义(函数体)组成。C语言默认使用值传递参数,但也可使用地址传递修改外部变量。函数可以有返回值或无返回值,返回值类型必须与声明一致。函数命名应清晰易懂,使用驼峰或下划线命名法。遵循单一职责原则,保持函数简洁性,以提高可维护性和可读性。
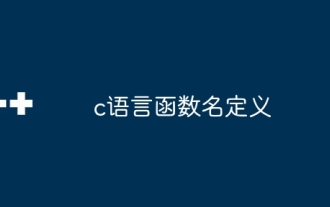
C语言函数名定义包括:返回值类型、函数名、参数列表和函数体。函数名应清晰、简洁、统一风格,避免与关键字冲突。函数名具有作用域,可在声明后使用。函数指针允许将函数作为参数传递或赋值。常见错误包括命名冲突、参数类型不匹配和未声明的函数。性能优化重点在函数设计和实现上,而清晰、易读的代码至关重要。
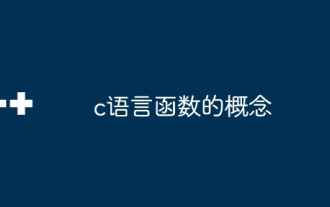
C语言函数是可重复利用的代码块,它接收输入,执行操作,返回结果,可将代码模块化提高可复用性,降低复杂度。函数内部机制包含参数传递、函数执行、返回值,整个过程涉及优化如函数内联。编写好的函数遵循单一职责原则、参数数量少、命名规范、错误处理。指针与函数结合能实现更强大的功能,如修改外部变量值。函数指针将函数作为参数传递或存储地址,用于实现动态调用函数。理解函数特性和技巧是编写高效、可维护、易理解的C语言程序的关键。
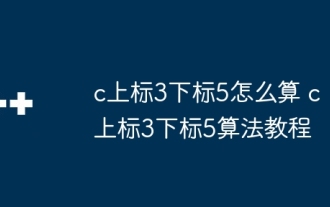
C35 的计算本质上是组合数学,代表从 5 个元素中选择 3 个的组合数,其计算公式为 C53 = 5! / (3! * 2!),可通过循环避免直接计算阶乘以提高效率和避免溢出。另外,理解组合的本质和掌握高效的计算方法对于解决概率统计、密码学、算法设计等领域的许多问题至关重要。
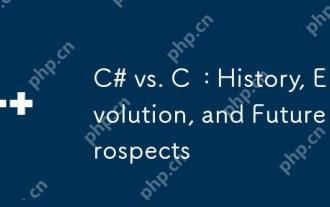
C#和C 的历史与演变各有特色,未来前景也不同。1.C 由BjarneStroustrup在1983年发明,旨在将面向对象编程引入C语言,其演变历程包括多次标准化,如C 11引入auto关键字和lambda表达式,C 20引入概念和协程,未来将专注于性能和系统级编程。2.C#由微软在2000年发布,结合C 和Java的优点,其演变注重简洁性和生产力,如C#2.0引入泛型,C#5.0引入异步编程,未来将专注于开发者的生产力和云计算。
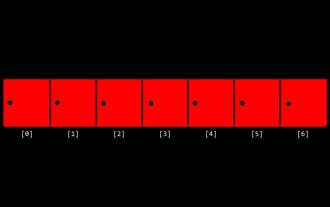
算法是解决问题的指令集,其执行速度和内存占用各不相同。编程中,许多算法都基于数据搜索和排序。本文将介绍几种数据检索和排序算法。线性搜索假设有一个数组[20,500,10,5,100,1,50],需要查找数字50。线性搜索算法会逐个检查数组中的每个元素,直到找到目标值或遍历完整个数组。算法流程图如下:线性搜索的伪代码如下:检查每个元素:如果找到目标值:返回true返回falseC语言实现:#include#includeintmain(void){i
