Python 中的 astype() 函数是什么
理解 Python 中的 astype()
astype() 函数是 Python 中的一个强大方法,主要用于 pandas 库,用于将 DataFrame 或 Series 中的列或数据集转换为特定数据类型。它也可在 NumPy 中用于将数组元素转换为不同类型。
astype() 的基本用法
astype() 函数用于将 pandas 对象(如 Series 或 DataFrame)或 NumPy 数组的数据类型转换为另一种类型。
pandas 的语法:
DataFrame.astype(dtype, copy=True, errors='raise')
NumPy 语法:
ndarray.astype(dtype, order='K', casting='unsafe', subok=True, copy=True)
关键参数
1.数据类型
要将数据转换为的目标数据类型。可以使用以下方式指定:
- 单一类型(例如 float、int、str)。
- 将列名映射到类型的字典(对于 pandas DataFrames)。
2.复制(pandas 和 NumPy)
- 默认:True
- 用途:是否返回原始数据的副本(如果为True)或就地修改(如果为False)。
3.错误(仅限熊猫)
-
选项:
- 'raise'(默认):如果转换失败则引发错误。
- 'ignore':默默地忽略错误。
4.顺序(仅限 NumPy)
- 控制输出数组的内存布局。选项:
- 'C':C-连续顺序。
- 'F':Fortran 连续顺序。
- 'A':如果输入是 Fortran 连续的,则使用 Fortran 顺序,否则使用 C 顺序。
- 'K':匹配输入数组的布局。
5.铸造(仅限 NumPy)
- 控制投射行为:
- 'no':不允许转换。
- 'equiv':仅允许字节顺序更改。
- “安全”:只允许保留值的强制转换。
- 'same_kind':仅允许安全强制转换或某种类型内的强制转换(例如,float -> int)。
- '不安全':允许任何数据转换。
6. subok(仅限 NumPy)
- 如果为 True,则传递子类;如果为 False,则返回的数组将是基类数组。
示例
1. pandas 的基本转换
import pandas as pd # Example DataFrame df = pd.DataFrame({'A': ['1', '2', '3'], 'B': [1.5, 2.5, 3.5]}) # Convert column 'A' to integer df['A'] = df['A'].astype(int) print(df.dtypes)
输出:
A int64 B float64 dtype: object
2.多列的字典映射
# Convert multiple columns df = df.astype({'A': float, 'B': int}) print(df.dtypes)
输出:
DataFrame.astype(dtype, copy=True, errors='raise')
3.使用错误='忽略'
ndarray.astype(dtype, order='K', casting='unsafe', subok=True, copy=True)
输出:
import pandas as pd # Example DataFrame df = pd.DataFrame({'A': ['1', '2', '3'], 'B': [1.5, 2.5, 3.5]}) # Convert column 'A' to integer df['A'] = df['A'].astype(int) print(df.dtypes)
- “two”的转换失败,但不会引发错误。
4.在 NumPy 中使用 astype()
A int64 B float64 dtype: object
输出:
# Convert multiple columns df = df.astype({'A': float, 'B': int}) print(df.dtypes)
5.在 NumPy 中使用casting='safe'进行铸造
A float64 B int64 dtype: object
输出:
df = pd.DataFrame({'A': ['1', 'two', '3'], 'B': [1.5, 2.5, 3.5]}) # Attempt conversion with errors='ignore' df['A'] = df['A'].astype(int, errors='ignore') print(df)
6.处理 pandas 中的非数字类型
A B 0 1 1.5 1 two 2.5 2 3 3.5
输出:
import numpy as np # Example array arr = np.array([1.1, 2.2, 3.3]) # Convert to integer arr_int = arr.astype(int) print(arr_int)
7.使用 astype() 进行内存优化
代码:
[1 2 3]
输出:
优化前(原始内存使用情况):
arr = np.array([1.1, 2.2, 3.3]) # Attempt an unsafe conversion try: arr_str = arr.astype(str, casting='safe') except TypeError as e: print(e)
优化后(优化内存使用):
Cannot cast array data from dtype('float64') to dtype('<U32') according to the rule 'safe'
说明:
-
原始内存使用情况:
- A 列作为 int64 使用 24 个字节(每个元素 8 个字节 × 3 个元素)。
- B 列作为 float64 使用 24 个字节(每个元素 8 个字节 × 3 个元素)。
-
优化内存使用:
- A 列作为 int8 使用 3 个字节(每个元素 1 个字节 × 3 个元素)。
- B 列作为 float32 使用 12 个字节(每个元素 4 个字节 × 3 个元素)。
使用较小的数据类型可以显着减少内存使用量,尤其是在处理大型数据集时。
常见陷阱
- 无效转换:转换不兼容的类型(例如,当存在非数字值时,将字符串转换为数字类型)。
df = pd.DataFrame({'A': ['2022-01-01', '2023-01-01'], 'B': ['True', 'False']}) # Convert to datetime and boolean df['A'] = pd.to_datetime(df['A']) df['B'] = df['B'].astype(bool) print(df.dtypes)
-
Errors='ignore'静默错误
:谨慎使用,因为它可能会静默地无法转换。 -
精度损失
:从高精度类型(例如 float64)转换为低精度类型(例如 float32)。
高级示例
1.复杂数据类型转换
A datetime64[ns] B bool dtype: object
输出:
import pandas as pd # Original DataFrame df = pd.DataFrame({'A': [1, 2, 3], 'B': [1.1, 2.2, 3.3]}) print("Original memory usage:") print(df.memory_usage()) # Downcast numerical types df['A'] = df['A'].astype('int8') df['B'] = df['B'].astype('float32') print("Optimized memory usage:") print(df.memory_usage())
2.在 NumPy 中使用 astype() 来处理结构化数组
Index 128 A 24 B 24 dtype: int64
输出:
DataFrame.astype(dtype, copy=True, errors='raise')
总结
astype() 函数是 pandas 和 NumPy 中数据类型转换的多功能工具。它允许对转换行为、内存优化和错误处理进行细粒度控制。正确使用其参数(例如 pandas 中的错误和 NumPy 中的转换)可确保稳健且高效的数据类型转换。
以上是Python 中的 astype() 函数是什么的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
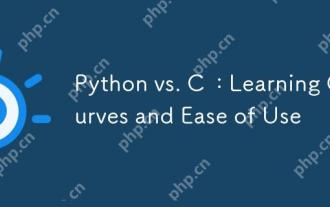
Python更易学且易用,C 则更强大但复杂。1.Python语法简洁,适合初学者,动态类型和自动内存管理使其易用,但可能导致运行时错误。2.C 提供低级控制和高级特性,适合高性能应用,但学习门槛高,需手动管理内存和类型安全。
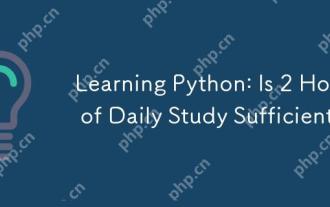
每天学习Python两个小时是否足够?这取决于你的目标和学习方法。1)制定清晰的学习计划,2)选择合适的学习资源和方法,3)动手实践和复习巩固,可以在这段时间内逐步掌握Python的基本知识和高级功能。
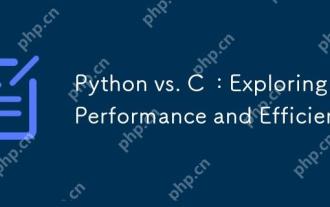
Python在开发效率上优于C ,但C 在执行性能上更高。1.Python的简洁语法和丰富库提高开发效率。2.C 的编译型特性和硬件控制提升执行性能。选择时需根据项目需求权衡开发速度与执行效率。
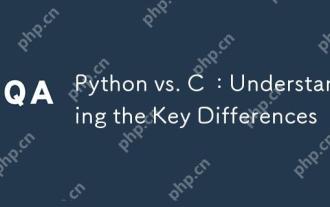
Python和C 各有优势,选择应基于项目需求。1)Python适合快速开发和数据处理,因其简洁语法和动态类型。2)C 适用于高性能和系统编程,因其静态类型和手动内存管理。
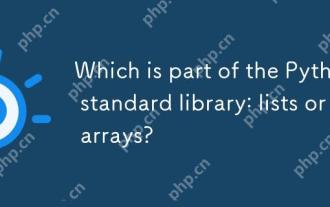
pythonlistsarepartofthestAndArdLibrary,herilearRaysarenot.listsarebuilt-In,多功能,和Rused ForStoringCollections,而EasaraySaraySaraySaraysaraySaraySaraysaraySaraysarrayModuleandleandleandlesscommonlyusedDduetolimitedFunctionalityFunctionalityFunctionality。
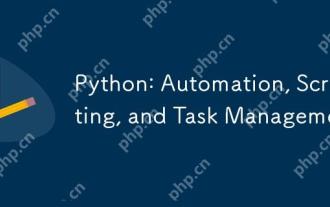
Python在自动化、脚本编写和任务管理中表现出色。1)自动化:通过标准库如os、shutil实现文件备份。2)脚本编写:使用psutil库监控系统资源。3)任务管理:利用schedule库调度任务。Python的易用性和丰富库支持使其在这些领域中成为首选工具。
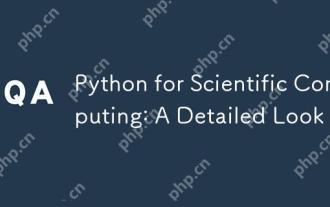
Python在科学计算中的应用包括数据分析、机器学习、数值模拟和可视化。1.Numpy提供高效的多维数组和数学函数。2.SciPy扩展Numpy功能,提供优化和线性代数工具。3.Pandas用于数据处理和分析。4.Matplotlib用于生成各种图表和可视化结果。
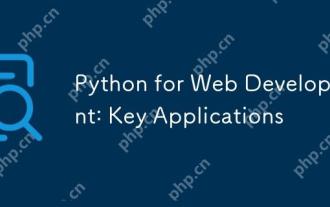
Python在Web开发中的关键应用包括使用Django和Flask框架、API开发、数据分析与可视化、机器学习与AI、以及性能优化。1.Django和Flask框架:Django适合快速开发复杂应用,Flask适用于小型或高度自定义项目。2.API开发:使用Flask或DjangoRESTFramework构建RESTfulAPI。3.数据分析与可视化:利用Python处理数据并通过Web界面展示。4.机器学习与AI:Python用于构建智能Web应用。5.性能优化:通过异步编程、缓存和代码优
