WebAssembly (WASM) 简介
WebAssembly (WASM) 是一种基于堆栈的虚拟机的二进制指令格式,设计为高性能应用程序的便携式目标。在本文中,我们将探讨如何将简单的 C 程序编译为 WebAssembly,将其加载到 Web 浏览器中,并使用 JavaScript 与其交互。我们还将探索一些在开发容器环境之外使用 WASM 的有用工具和命令。
设置开发环境
为您的 WebAssembly 项目创建必要的文件夹结构和文件。
- 创建项目文件夹: 首先为您的项目创建一个新目录。在此文件夹中,您将添加必要的文件和配置。
mkdir wasm-web-example cd wasm-web-example
- 设置开发容器: 在 wasm-web-example 目录中,创建 .devcontainer 文件夹来存储 dev 容器配置文件。这些文件将设置一个安装了 Emscripten 的容器,用于将 C 代码编译成 WebAssembly。
在 .devcontainer 文件夹中,创建以下文件:
-
devcontainer.json
此文件将 VSCode 配置为使用具有必要扩展和环境设置的 Docker 容器。
{ "name": "Emscripten DevContainer", "build": { "dockerfile": "Dockerfile" }, "customizations": { "vscode": { "settings": { "terminal.integrated.shell.linux": "/bin/bash", "C_Cpp.default.configurationProvider": "ms-vscode.cmake-tools", "C_Cpp.default.intelliSenseMode": "gcc-x64" }, "extensions": [ "ms-vscode.cpptools", "ms-vscode.cmake-tools" ] } }, "postCreateCommand": "emcc --version" }
登录后复制登录后复制 -
Dockerfile
Dockerfile 将设置 Emscripten 环境。这是该文件的内容:
# Use the official Emscripten image FROM emscripten/emsdk:3.1.74 # Set the working directory WORKDIR /workspace # Copy the source code into the container COPY . . # Install any additional packages if necessary (optional) # Ensure to clean up cache to minimize image size RUN apt-get update && \ apt-get install -y build-essential && \ apt-get clean && \ rm -rf /var/lib/apt/lists/*
登录后复制登录后复制
- 创建 VSCode 设置: 在项目的根目录中,创建一个包含以下文件的 .vscode 文件夹:
-
c_cpp_properties.json
此文件配置 C IntelliSense 并包含项目的路径。
{ "configurations": [ { "name": "Linux", "includePath": [ "${workspaceFolder}/**", "/emsdk/upstream/emscripten/system/include" ], "defines": [], "compilerPath": "/usr/bin/gcc", "cStandard": "c17", "cppStandard": "gnu++17", "configurationProvider": "ms-vscode.cmake-tools" } ], "version": 4 }
登录后复制登录后复制 -
settings.json
此文件包含语言关联的特定 VSCode 设置。
{ "files.associations": { "emscripten.h": "c" }, "[javascript]": { "editor.defaultFormatter": "vscode.typescript-language-features" }, "[typescript]": { "editor.defaultFormatter": "vscode.typescript-language-features" }, "[jsonc]": { "editor.defaultFormatter": "vscode.json-language-features" }, "[json]": { "editor.defaultFormatter": "vscode.json-language-features" }, "[html]": { "editor.defaultFormatter": "vscode.html-language-features" } }
登录后复制
- 创建 C、JavaScript 和 HTML 文件: 现在,为您的项目创建以下文件:
-
test.c
这个 C 文件包含将被编译为 WebAssembly 的简单函数。
// test.c int add(int lhs, int rhs) { return lhs + rhs; }
登录后复制 -
test.html
此 HTML 文件将使用 JavaScript 加载 WebAssembly 模块。
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>WebAssembly Example</title> </head> <body> <h1>WebAssembly Example</h1> <div> </li> <li> <p><strong>test.js</strong><br><br> This JavaScript file will fetch the WebAssembly module and call the exported function.<br> </p> <pre class="brush:php;toolbar:false"> // test.js const wasmFile = 'test.wasm'; fetch(wasmFile) .then(response => response.arrayBuffer()) .then(bytes => WebAssembly.instantiate(bytes)) .then(({ instance }) => { const result = instance.exports.add(5, 3); // Call the WebAssembly function document.getElementById('output').textContent = `Result from WebAssembly: ${result}`; }) .catch(error => console.error('Error loading WebAssembly module:', error));
登录后复制
现在您已经设置了所有必要的文件和配置,您可以继续编译 WebAssembly 并与 WebAssembly 交互。
使用 Emscripten 将 C 代码编译为 WebAssembly
基础 C 程序:
文件 test.c 包含一个简单的函数 add,用于将两个整数相加。我们将使用 Emscripten 将此 C 函数编译为 WebAssembly。Emscripten 命令:
在 dev 容器内,打开终端(在 VSCode 中使用 cmd j)并运行以下 Emscripten 命令将 C 代码编译为 WebAssembly:
mkdir wasm-web-example cd wasm-web-example
此命令将生成 test.wasm(WebAssembly 二进制文件),并确保导出 add 函数以在 JavaScript 中使用。
在浏览器中加载 WebAssembly 并与之交互
HTML 设置:
文件 test.html 包含一个简单的 HTML 页面,该页面使用 JavaScript 加载 WebAssembly 二进制文件。JavaScript 设置:
JavaScript 文件 test.js 加载 test.wasm 文件并调用导出的 add 函数:
在 macOS 上使用外部工具
在开发容器之外,您可以运行几个有用的命令来在 Mac 上使用 WebAssembly。
- 安装wabt: wabt(WebAssembly 二进制工具包)提供了用于使用 WebAssembly 的实用程序,包括将 .wasm 文件转换为人类可读的 WAT(WebAssembly 文本)格式。通过 Homebrew 安装:
{ "name": "Emscripten DevContainer", "build": { "dockerfile": "Dockerfile" }, "customizations": { "vscode": { "settings": { "terminal.integrated.shell.linux": "/bin/bash", "C_Cpp.default.configurationProvider": "ms-vscode.cmake-tools", "C_Cpp.default.intelliSenseMode": "gcc-x64" }, "extensions": [ "ms-vscode.cpptools", "ms-vscode.cmake-tools" ] } }, "postCreateCommand": "emcc --version" }
- 将 WASM 转换为 WAT: 安装 wabt 后,您可以使用 wasm2wat 工具将 WebAssembly 二进制文件 (test.wasm) 转换为 WAT 格式:
# Use the official Emscripten image FROM emscripten/emsdk:3.1.74 # Set the working directory WORKDIR /workspace # Copy the source code into the container COPY . . # Install any additional packages if necessary (optional) # Ensure to clean up cache to minimize image size RUN apt-get update && \ apt-get install -y build-essential && \ apt-get clean && \ rm -rf /var/lib/apt/lists/*
- 提供 HTML 页面: 要查看与 WebAssembly 模块交互的 HTML 页面,可以使用 Python 的简单 HTTP 服务器:
{ "configurations": [ { "name": "Linux", "includePath": [ "${workspaceFolder}/**", "/emsdk/upstream/emscripten/system/include" ], "defines": [], "compilerPath": "/usr/bin/gcc", "cStandard": "c17", "cppStandard": "gnu++17", "configurationProvider": "ms-vscode.cmake-tools" } ], "version": 4 }
结论
通过执行以下步骤,您可以设置一个开发环境,将 C 代码编译为 WebAssembly,使用 JavaScript 与其交互,并转换生成的二进制文件以供检查。使用 wabt 和 Python 的 HTTP 服务器等外部工具简化了 macOS 系统上的 WebAssembly 模块的管理和探索。
以上是WebAssembly (WASM) 简介的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
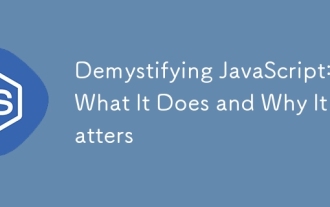
JavaScript是现代Web开发的基石,它的主要功能包括事件驱动编程、动态内容生成和异步编程。1)事件驱动编程允许网页根据用户操作动态变化。2)动态内容生成使得页面内容可以根据条件调整。3)异步编程确保用户界面不被阻塞。JavaScript广泛应用于网页交互、单页面应用和服务器端开发,极大地提升了用户体验和跨平台开发的灵活性。
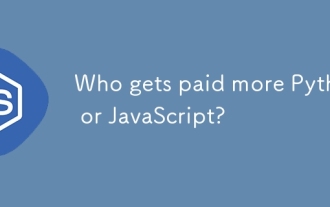
Python和JavaScript开发者的薪资没有绝对的高低,具体取决于技能和行业需求。1.Python在数据科学和机器学习领域可能薪资更高。2.JavaScript在前端和全栈开发中需求大,薪资也可观。3.影响因素包括经验、地理位置、公司规模和特定技能。
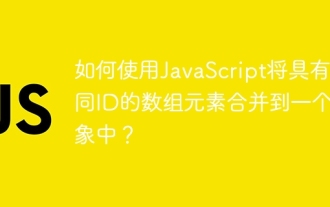
如何在JavaScript中将具有相同ID的数组元素合并到一个对象中?在处理数据时,我们常常会遇到需要将具有相同ID�...
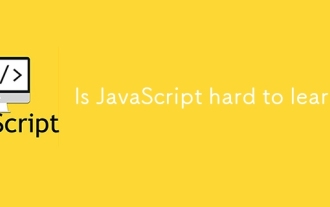
学习JavaScript不难,但有挑战。1)理解基础概念如变量、数据类型、函数等。2)掌握异步编程,通过事件循环实现。3)使用DOM操作和Promise处理异步请求。4)避免常见错误,使用调试技巧。5)优化性能,遵循最佳实践。
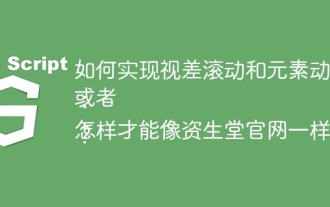
实现视差滚动和元素动画效果的探讨本文将探讨如何实现类似资生堂官网(https://www.shiseido.co.jp/sb/wonderland/)中�...
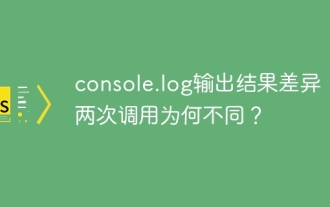
深入探讨console.log输出差异的根源本文将分析一段代码中console.log函数输出结果的差异,并解释其背后的原因。�...
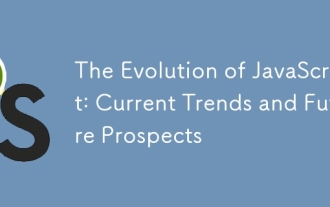
JavaScript的最新趋势包括TypeScript的崛起、现代框架和库的流行以及WebAssembly的应用。未来前景涵盖更强大的类型系统、服务器端JavaScript的发展、人工智能和机器学习的扩展以及物联网和边缘计算的潜力。
