如何在不同的应用程序中可靠地复制和粘贴透明图像?
在不同应用程序中可靠复制粘贴透明图像
您可能遇到过这样的问题:在复制粘贴图像时,透明度无法在不同程序间保持一致。尽管Chrome可以保留透明度,但使用系统剪贴板对象设置图像时,透明度可能会丢失。
剪贴板限制
Windows剪贴板本身并不原生支持图像透明度。然而,您可以利用多种数据类型来提高跨应用程序的兼容性。
PNG流:最佳兼容性选择
PNG流是保持剪贴板图像透明度的最可靠方法。将PNG图像粘贴到GIMP或较新的MS Office程序中,透明度将被保留。
多种格式,更广兼容性
为了确保与更多应用程序兼容,建议在剪贴板上包含多种支持的图像格式,例如PNG、设备无关位图(DIB)和标准位图。
示例代码
public static void SetClipboardImage(Bitmap image, Bitmap imageNoTr, DataObject data) { Clipboard.Clear(); if (data == null) data = new DataObject(); if (imageNoTr == null) imageNoTr = image; using (MemoryStream pngMemStream = new MemoryStream()) using (MemoryStream dibMemStream = new MemoryStream()) { data.SetData(DataFormats.Bitmap, true, imageNoTr); // 设置标准位图(无透明度) image.Save(pngMemStream, ImageFormat.Png); data.SetData("PNG", false, pngMemStream); // 设置PNG流(GIMP和较新MS Office兼容) Byte[] dibData = ConvertToDib(image); dibMemStream.Write(dibData, 0, dibData.Length); data.SetData(DataFormats.Dib, false, dibMemStream); // 设置DIB格式(处理错误解释透明度的应用) Clipboard.SetDataObject(data, true); } } public static Byte[] ConvertToDib(Image image) { using (Bitmap bm32b = new Bitmap(image.Width, image.Height, PixelFormat.Format32bppArgb)) { using (Graphics gr = Graphics.FromImage(bm32b)) gr.DrawImage(image, new Rectangle(0, 0, bm32b.Width, bm32b.Height)); bm32b.RotateFlip(RotateFlipType.Rotate180FlipX); Int32 stride; Byte[] bm32bData = ImageUtils.GetImageData(bm32b, out stride); Int32 hdrSize = 0x28; Byte[] fullImage = new Byte[hdrSize + 12 + bm32bData.Length]; ArrayUtils.WriteIntToByteArray(fullImage, 0x00, 4, true, (UInt32)hdrSize); ArrayUtils.WriteIntToByteArray(fullImage, 0x04, 4, true, (UInt32)image.Width); ArrayUtils.WriteIntToByteArray(fullImage, 0x08, 4, true, (UInt32)image.Height); ArrayUtils.WriteIntToByteArray(fullImage, 0x0C, 2, true, 1); ArrayUtils.WriteIntToByteArray(fullImage, 0x0E, 2, true, 32); ArrayUtils.WriteIntToByteArray(fullImage, 0x10, 4, true, 3); ArrayUtils.WriteIntToByteArray(fullImage, 0x14, 4, true, (UInt32)bm32bData.Length); ArrayUtils.WriteIntToByteArray(fullImage, hdrSize + 0, 4, true, 0x00FF0000); ArrayUtils.WriteIntToByteArray(fullImage, hdrSize + 4, 4, true, 0x0000FF00); ArrayUtils.WriteIntToByteArray(fullImage, hdrSize + 8, 4, true, 0x000000FF); Array.Copy(bm32bData, 0, fullImage, hdrSize + 12, bm32bData.Length); return fullImage; } }
从剪贴板提取图像
要从剪贴板提取图像,需要检查各种支持的格式,优先使用PNG以确保最大可靠性:
public static Bitmap GetClipboardImage(DataObject retrievedData) { if (retrievedData.GetDataPresent("PNG", false)) { MemoryStream png_stream = retrievedData.GetData("PNG", false) as MemoryStream; if (png_stream != null) using (Bitmap bm = new Bitmap(png_stream)) return ImageUtils.CloneImage(bm); } else if (retrievedData.GetDataPresent(DataFormats.Dib, false)) { MemoryStream dib = retrievedData.GetData(DataFormats.Dib, false) as MemoryStream; if (dib != null) return ImageFromClipboardDib(dib.ToArray()); } else if (retrievedData.GetDataPresent(DataFormats.Bitmap)) return new Bitmap(retrievedData.GetData(DataFormats.Bitmap) as Image); else if (retrievedData.GetDataPresent(typeof(Image))) return new Bitmap(retrievedData.GetData(typeof(Image)) as Image); return null; } public static Bitmap ImageFromClipboardDib(Byte[] dibBytes) { if (dibBytes == null || dibBytes.Length < 4 || dibBytes.Length < (Int32)ArrayUtils.ReadIntFromByteArray(dibBytes, 0, 4, true)) return null; Int32 width = (Int32)ArrayUtils.ReadIntFromByteArray(dibBytes, 0x04, 4, true); Int32 height = (Int32)ArrayUtils.ReadIntFromByteArray(dibBytes, 0x08, 4, true); Int16 planes = (Int16)ArrayUtils.ReadIntFromByteArray(dibBytes, 0x0C, 2, true); Int16 bitCount = (Int16)ArrayUtils.ReadIntFromByteArray(dibBytes, 0x0E, 2, true); Int32 compression = (Int32)ArrayUtils.ReadIntFromByteArray(dibBytes, 0x10, 4, true); if (planes != 1 || (compression != 0 && compression != 3)) return null; PixelFormat fmt; switch (bitCount) { case 32: fmt = PixelFormat.Format32bppRgb; break; case 24: fmt = PixelFormat.Format24bppRgb; break; case 16: fmt = PixelFormat.Format16bppRgb555; break; default: return null; } Int32 stride = (((((bitCount * width) + 7) / 8) + 3) / 4) * 4; Int32 imageIndex = 40 + (compression == 3 ? 12 : 0); if (dibBytes.Length < imageIndex) return null; Byte[] image = new Byte[dibBytes.Length - imageIndex]; Array.Copy(dibBytes, imageIndex, image, 0, image.Length); Bitmap bitmap = ImageUtils.BuildImage(image, width, height, stride, fmt, null, null); // ... (ImageFromClipboardDib function continues, requires ImageUtils.BuildImage implementation) ... }
(注意:上述代码片段中ImageUtils.GetImageData
、ImageUtils.CloneImage
和 ImageUtils.BuildImage
需要根据实际情况自行实现。) 这些函数负责图像数据的处理和位图的创建。 这部分实现取决于你使用的图像处理库和具体的平台环境。
以上是如何在不同的应用程序中可靠地复制和粘贴透明图像?的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
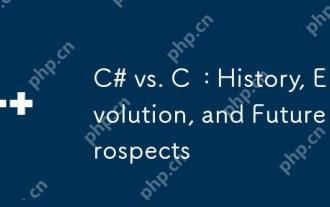
C#和C 的历史与演变各有特色,未来前景也不同。1.C 由BjarneStroustrup在1983年发明,旨在将面向对象编程引入C语言,其演变历程包括多次标准化,如C 11引入auto关键字和lambda表达式,C 20引入概念和协程,未来将专注于性能和系统级编程。2.C#由微软在2000年发布,结合C 和Java的优点,其演变注重简洁性和生产力,如C#2.0引入泛型,C#5.0引入异步编程,未来将专注于开发者的生产力和云计算。
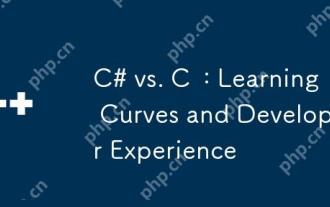
C#和C 的学习曲线和开发者体验有显着差异。 1)C#的学习曲线较平缓,适合快速开发和企业级应用。 2)C 的学习曲线较陡峭,适用于高性能和低级控制的场景。
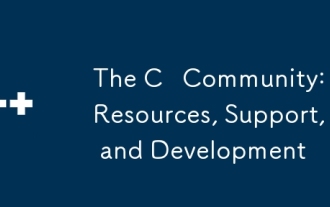
C 学习者和开发者可以从StackOverflow、Reddit的r/cpp社区、Coursera和edX的课程、GitHub上的开源项目、专业咨询服务以及CppCon等会议中获得资源和支持。1.StackOverflow提供技术问题的解答;2.Reddit的r/cpp社区分享最新资讯;3.Coursera和edX提供正式的C 课程;4.GitHub上的开源项目如LLVM和Boost提升技能;5.专业咨询服务如JetBrains和Perforce提供技术支持;6.CppCon等会议有助于职业
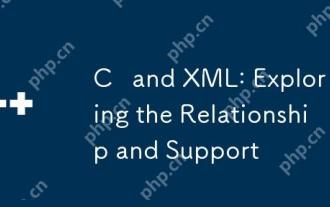
C 通过第三方库(如TinyXML、Pugixml、Xerces-C )与XML交互。1)使用库解析XML文件,将其转换为C 可处理的数据结构。2)生成XML时,将C 数据结构转换为XML格式。3)在实际应用中,XML常用于配置文件和数据交换,提升开发效率。
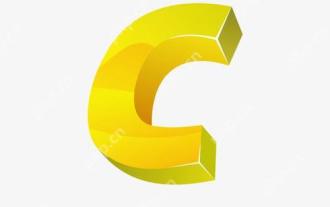
静态分析在C 中的应用主要包括发现内存管理问题、检查代码逻辑错误和提高代码安全性。1)静态分析可以识别内存泄漏、双重释放和未初始化指针等问题。2)它能检测未使用变量、死代码和逻辑矛盾。3)静态分析工具如Coverity能发现缓冲区溢出、整数溢出和不安全API调用,提升代码安全性。
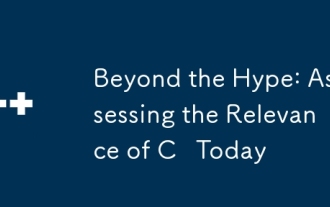
C 在现代编程中仍然具有重要相关性。1)高性能和硬件直接操作能力使其在游戏开发、嵌入式系统和高性能计算等领域占据首选地位。2)丰富的编程范式和现代特性如智能指针和模板编程增强了其灵活性和效率,尽管学习曲线陡峭,但其强大功能使其在今天的编程生态中依然重要。
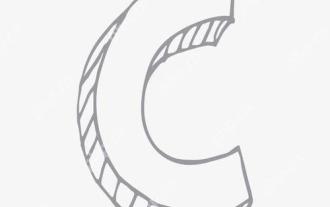
使用C 中的chrono库可以让你更加精确地控制时间和时间间隔,让我们来探讨一下这个库的魅力所在吧。C 的chrono库是标准库的一部分,它提供了一种现代化的方式来处理时间和时间间隔。对于那些曾经饱受time.h和ctime折磨的程序员来说,chrono无疑是一个福音。它不仅提高了代码的可读性和可维护性,还提供了更高的精度和灵活性。让我们从基础开始,chrono库主要包括以下几个关键组件:std::chrono::system_clock:表示系统时钟,用于获取当前时间。std::chron
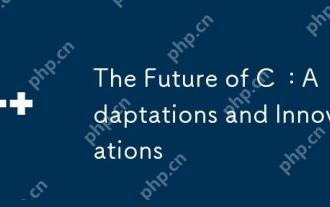
C 的未来将专注于并行计算、安全性、模块化和AI/机器学习领域:1)并行计算将通过协程等特性得到增强;2)安全性将通过更严格的类型检查和内存管理机制提升;3)模块化将简化代码组织和编译;4)AI和机器学习将促使C 适应新需求,如数值计算和GPU编程支持。
