JavaScript 开发人员的基本设计模式:提高您的编码掌握程度
JavaScript 开发人员的 7 个基本设计模式:提高您的编码能力
在软件开发的动态世界中,理解设计模式对于创建可扩展、可维护和高效的代码至关重要。无论您是在处理小型项目还是构建复杂的应用程序,设计模式都可以为常见挑战提供经过时间考验的解决方案。这篇文章深入探讨了每个 JavaScript 开发人员都应该知道的七个关键设计模式,并通过实际示例来增强您的编码专业知识。
— -
1。单例模式:确保单个实例
单例模式确保一个类只有一个实例,同时提供全局访问点。它非常适合管理单个配置对象或集中状态等场景。
class Singleton { constructor() { if (Singleton.instance) { return Singleton.instance; } Singleton.instance = this; this.data = {}; } setData(key, value) { this.data[key] = value; } getData(key) { return this.data[key]; } } // Example Usage const instance1 = new Singleton(); instance1.setData("theme", "dark"); const instance2 = new Singleton(); console.log(instance2.getData("theme")); // Output: dark
— -
2。工厂模式:简化对象创建
工厂模式提供了一种创建对象的方法,而无需指定其确切的类。这种模式对于构建具有多种对象类型的应用程序非常有价值。
class Car { constructor(model) { this.type = "Car"; this.model = model; } } class Bike { constructor(model) { this.type = "Bike"; this.model = model; } } class VehicleFactory { static createVehicle(type, model) { if (type === "car") return new Car(model); if (type === "bike") return new Bike(model); throw new Error("Unknown vehicle type"); } } // Example Usage const tesla = VehicleFactory.createVehicle("car", "Tesla"); console.log(tesla); // Output: Car { type: 'Car', model: 'Tesla' }
— -
3。观察者模式:对变化做出反应
在观察者模式中,多个对象(观察者)观察单个主题。当主体的状态发生变化时,所有观察者都会收到通知。这种模式在 GUI 等事件驱动系统中特别有用。
class Subject { constructor() { this.observers = []; } subscribe(observer) { this.observers.push(observer); } notify(data) { this.observers.forEach(observer => observer.update(data)); } } class Observer { constructor(name) { this.name = name; } update(data) { console.log(`${this.name} received: ${data}`); } } // Example Usage const newsChannel = new Subject(); const subscriber1 = new Observer("Alice"); const subscriber2 = new Observer("Bob"); newsChannel.subscribe(subscriber1); newsChannel.subscribe(subscriber2); newsChannel.notify("Breaking News!");
— -
4。装饰器模式:增强功能
装饰器模式允许您动态地向对象添加行为,而无需修改其结构。这对于以模块化方式扩展功能特别有用。
function withTimestamp(func) { return function(message) { func(`[${new Date().toISOString()}] ${message}`); }; } // Example Usage const log = console.log; const logWithTimestamp = withTimestamp(log); logWithTimestamp("Hello, World!"); // Output: [2024–12–14T12:00:00.000Z] Hello, World!
— -
5。策略模式:动态算法切换
策略模式定义了一系列算法,封装它们,并使它们可以互换。这非常适合需要基于用户输入或上下文的多种行为的应用程序。
class PaymentStrategy { pay(amount) { throw new Error("pay() must be implemented."); } } class CreditCardPayment extends PaymentStrategy { pay(amount) { console.log(`Paid $${amount} with Credit Card.`); } } class PayPalPayment extends PaymentStrategy { pay(amount) { console.log(`Paid $${amount} with PayPal.`); } } // Example Usage const paymentMethod = new PayPalPayment(); paymentMethod.pay(100); // Output: Paid 0 with PayPal.
— -
6。原型模式:对象克隆变得简单
此模式允许通过复制原型来创建对象。它通常用于对象组合和避免重复实例化。
const vehiclePrototype = { start() { console.log(`${this.model} is starting.`); }, }; function createVehicle(model) { const vehicle = Object.create(vehiclePrototype); vehicle.model = model; return vehicle; } // Example Usage const car = createVehicle("Toyota"); car.start(); // Output: Toyota is starting.
— -
7。命令模式:封装请求
命令模式将请求封装为对象,实现灵活的操作调度和撤消功能。
class Light { on() { console.log("Light is ON"); } off() { console.log("Light is OFF"); } } class LightOnCommand { constructor(light) { this.light = light; } execute() { this.light.on(); } } // Example Usage const light = new Light(); const lightOnCommand = new LightOnCommand(light); lightOnCommand.execute(); // Output: Light is ON
— -
最后的想法
掌握这些设计模式将为您提供强大的工具来编写更好的代码。通过了解它们的实际应用,您可以有效地应对挑战并构建强大的软件。您最喜欢哪种设计模式?在下面的评论中分享你的想法!
以上是JavaScript 开发人员的基本设计模式:提高您的编码掌握程度的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
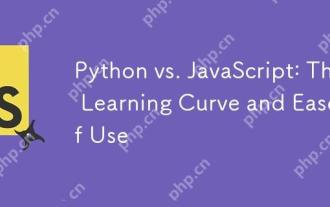
Python更适合初学者,学习曲线平缓,语法简洁;JavaScript适合前端开发,学习曲线较陡,语法灵活。1.Python语法直观,适用于数据科学和后端开发。2.JavaScript灵活,广泛用于前端和服务器端编程。
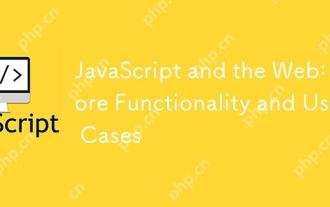
JavaScript在Web开发中的主要用途包括客户端交互、表单验证和异步通信。1)通过DOM操作实现动态内容更新和用户交互;2)在用户提交数据前进行客户端验证,提高用户体验;3)通过AJAX技术实现与服务器的无刷新通信。
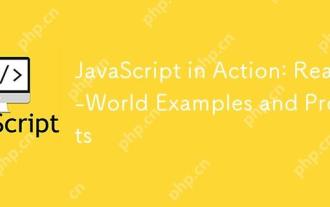
JavaScript在现实世界中的应用包括前端和后端开发。1)通过构建TODO列表应用展示前端应用,涉及DOM操作和事件处理。2)通过Node.js和Express构建RESTfulAPI展示后端应用。
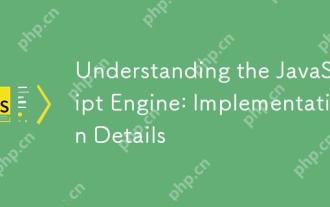
理解JavaScript引擎内部工作原理对开发者重要,因为它能帮助编写更高效的代码并理解性能瓶颈和优化策略。1)引擎的工作流程包括解析、编译和执行三个阶段;2)执行过程中,引擎会进行动态优化,如内联缓存和隐藏类;3)最佳实践包括避免全局变量、优化循环、使用const和let,以及避免过度使用闭包。
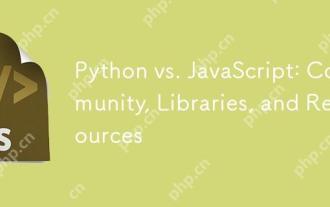
Python和JavaScript在社区、库和资源方面的对比各有优劣。1)Python社区友好,适合初学者,但前端开发资源不如JavaScript丰富。2)Python在数据科学和机器学习库方面强大,JavaScript则在前端开发库和框架上更胜一筹。3)两者的学习资源都丰富,但Python适合从官方文档开始,JavaScript则以MDNWebDocs为佳。选择应基于项目需求和个人兴趣。
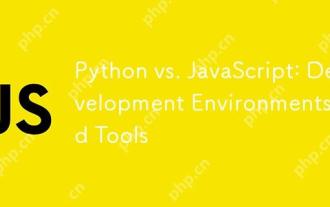
Python和JavaScript在开发环境上的选择都很重要。1)Python的开发环境包括PyCharm、JupyterNotebook和Anaconda,适合数据科学和快速原型开发。2)JavaScript的开发环境包括Node.js、VSCode和Webpack,适用于前端和后端开发。根据项目需求选择合适的工具可以提高开发效率和项目成功率。
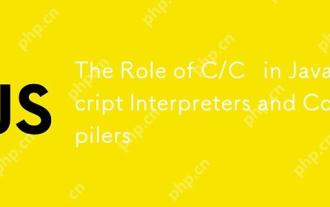
C和C 在JavaScript引擎中扮演了至关重要的角色,主要用于实现解释器和JIT编译器。 1)C 用于解析JavaScript源码并生成抽象语法树。 2)C 负责生成和执行字节码。 3)C 实现JIT编译器,在运行时优化和编译热点代码,显着提高JavaScript的执行效率。

Python更适合数据科学和自动化,JavaScript更适合前端和全栈开发。1.Python在数据科学和机器学习中表现出色,使用NumPy、Pandas等库进行数据处理和建模。2.Python在自动化和脚本编写方面简洁高效。3.JavaScript在前端开发中不可或缺,用于构建动态网页和单页面应用。4.JavaScript通过Node.js在后端开发中发挥作用,支持全栈开发。
