了解选择排序算法(附Java示例)
选择排序:分步指南
选择排序是一种简单的排序算法。 它反复从列表的未排序部分中查找最小元素并将其放在开头。这个过程一直持续到整个列表被排序为止。
选择排序的工作原理
让我们用一个例子来说明,按升序对这个数组进行排序:
迭代 1:
目标是将最小的元素放在开头。我们首先假设第一个元素是最小值。
我们将当前最小值与每个后续元素进行比较,如果找到更小的元素,则更新最小值。
这将持续到确定实际的最小值。
最后,我们将最小元素与第一个元素交换。
第一个元素现已排序。 后续迭代将仅考虑未排序的部分。
后续迭代:
对每个剩余的未排序元素重复此过程。
算法迭代 n-1 次(其中 n 是数组的长度)。 第五次迭代后(对于六元素数组),最后一个元素被隐式排序。
选择排序实现(Java):
import java.util.Arrays; public class SelectionSortTest { public static void main(String[] args) { int[] arr = {8, 2, 6, 4, 9, 1}; System.out.println("Unsorted array: " + Arrays.toString(arr)); selectionSort(arr); System.out.println("Sorted array: " + Arrays.toString(arr)); } public static void selectionSort(int[] arr) { int size = arr.length; // Iterate through the array size-1 times for (int i = 0; i < size - 1; i++) { int minIndex = i; // Find the minimum element in the unsorted part for (int j = i + 1; j < size; j++) { if (arr[j] < arr[minIndex]) { minIndex = j; } } // Swap the minimum element with the first unsorted element int temp = arr[minIndex]; arr[minIndex] = arr[i]; arr[i] = temp; } } }
输出:
未排序数组:[8,2,6,4,9,1] 排序数组:[1, 2, 4, 6, 8, 9]
复杂性分析:
- 时间复杂度: 所有情况下都是 O(n²)(最佳、平均、最差)。 无论输入顺序如何,嵌套循环始终执行固定次数。
- 空间复杂度: O(1)。 这是一种就地算法,需要恒定的额外空间。
结论:
选择排序的 O(n²) 时间复杂度使其对于大型数据集效率低下。 它最适合小型阵列或简单性优先于性能的情况。
以上是了解选择排序算法(附Java示例)的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
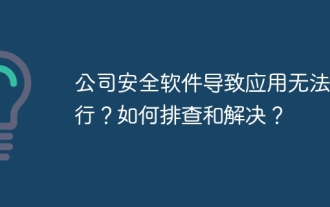
公司安全软件导致部分应用无法正常运行的排查与解决方法许多公司为了保障内部网络安全,会部署安全软件。...
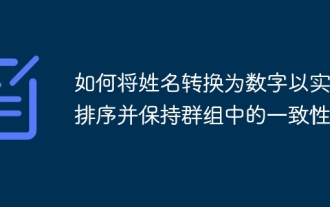
将姓名转换为数字以实现排序的解决方案在许多应用场景中,用户可能需要在群组中进行排序,尤其是在一个用...
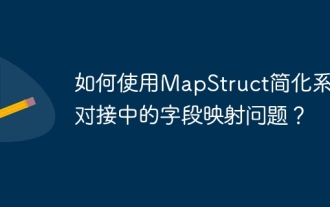
系统对接中的字段映射处理在进行系统对接时,常常会遇到一个棘手的问题:如何将A系统的接口字段有效地映�...
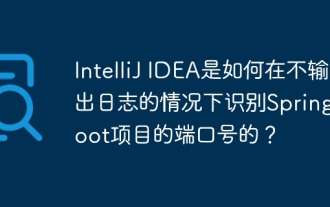
在使用IntelliJIDEAUltimate版本启动Spring...
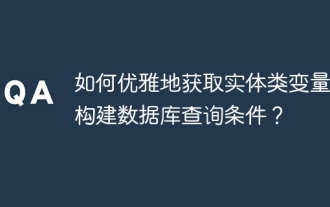
在使用MyBatis-Plus或其他ORM框架进行数据库操作时,经常需要根据实体类的属性名构造查询条件。如果每次都手动...
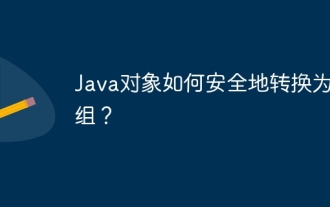
Java对象与数组的转换:深入探讨强制类型转换的风险与正确方法很多Java初学者会遇到将一个对象转换成数组的�...
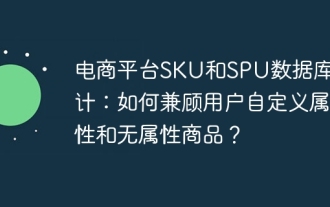
电商平台SKU和SPU表设计详解本文将探讨电商平台中SKU和SPU的数据库设计问题,特别是如何处理用户自定义销售属...
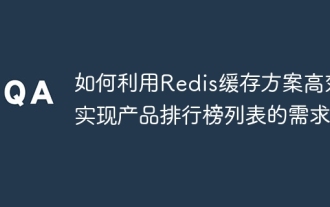
Redis缓存方案如何实现产品排行榜列表的需求?在开发过程中,我们常常需要处理排行榜的需求,例如展示一个�...
