如何使用 C# 识别 2D 点云中的凸孔并将其多边形化?
此代码演示了一种在一组 2d 点中查找凸孔的方法。该方法包括创建点云位图、计算位图中每个单元的数据密度、创建未使用区域的列表(map[][] = 0 或 map[][]
这是该算法的 C# 实现提供:
using System; using System.Collections.Generic; using System.Linq; using System.Drawing; namespace HoleFinder { class Program { // Define a cell structure for the bitmap public struct Cell { public double x0, x1, y0, y1; // Bounding box of points inside the cell public int count; // Number of points inside the cell } // Define a line structure for representing hole boundaries public struct Line { public double x0, y0, x1, y1; // Line edge points public int id; // Id of the hole to which the line belongs for segmentation/polygonization public int i0, i1, j0, j1; // Index in map[][] } // Compute the bounding box of the point cloud public static (double x0, double x1, double y0, double y1) ComputeBoundingBox(List<Point> points) { double x0 = points[0].X; double x1 = points[0].X; double y0 = points[0].Y; double y1 = points[0].Y; foreach (var point in points) { if (point.X < x0) x0 = point.X; if (point.X > x1) x1 = point.X; if (point.Y < y0) y0 = point.Y; if (point.Y > y1) y1 = point.Y; } return (x0, x1, y0, y1); } // Create a bitmap of the point cloud public static int[,] CreateBitmap(List<Point> points, (double x0, double x1, double y0, double y1) boundingBox, int N) { // Create a 2D array to represent the bitmap int[,] bitmap = new int[N, N]; // Compute the scale factors for converting point coordinates to bitmap indices double mx = N / (boundingBox.x1 - boundingBox.x0); double my = N / (boundingBox.y1 - boundingBox.y0); // Iterate over the points and increment the corresponding cells in the bitmap foreach (var point in points) { int i = (int)Math.Round((point.X - boundingBox.x0) * mx); int j = (int)Math.Round((point.Y - boundingBox.y0) * my); if (i >= 0 && i < N && j >= 0 && j < N) bitmap[i, j]++; } return bitmap; } // Compute the data density for each cell in the bitmap public static void ComputeDataDensity(int[,] bitmap, Cell[] map) { for (int i = 0; i < map.Length; i++) { map[i].count = 0; } for (int i = 0; i < bitmap.GetLength(0); i++) { for (int j = 0; j < bitmap.GetLength(1); j++) { map[i * bitmap.GetLength(1) + j].count += bitmap[i, j]; } } } // Create a list of unused areas (map[][] = 0 or map[][] <= treshold) public static List<(int i0, int i1, int j0, int j1)> FindUnusedAreasHorizontalVertical(Cell[] map, int N, int treshold = 0) { List<(int i0, int i1, int j0, int j1)> unusedAreas = new List<(int, int, int, int)>(); // Scan horizontally for (int j = 0; j < N; j++) { int i0 = -1; int i1 = -1; for (int i = 0; i < N; i++) { if (map[i * N + j].count == 0 || map[i * N + j].count <= treshold) { if (i0 < 0) i0 = i; } else { if (i0 >= 0) { unusedAreas.Add((i0, i1, j, j)); i0 = -1; i1 = -1; } } } if (i0 >= 0) unusedAreas.Add((i0, i1, j, j)); } // Scan vertically for (int i = 0; i < N; i++) { int j0 = -1; int j1 = -1; for (int j = 0; j < N; j++) { if (map[i * N + j].count == 0 || map[i * N + j].count <= treshold) { if (j0 < 0) j0 = j; } else { if (j0 >= 0) { unusedAreas.Add((i, i, j0, j1)); j0 = -1; j1 = -1; } } } if (j0 >= 0) unusedAreas.Add((i, i, j0, j1)); } return unusedAreas; } // Segment the list of unused areas into groups of connected components public static List<List<(int i0, int i1, int j0, int j1)>> SegmentUnusedAreas(List<(int i0, int i1, int j0, int j1)> unusedAreas) { // Initialize each unused area as a separate group List<List<(int i0, int i1, int j0, int j1)>> segments = new List<List<(int i0, int i1, int j0, int j1)>>(); foreach (var unusedArea in unusedAreas) { segments.Add(new List<(int i0, int i1, int j0, int j1)> { unusedArea }); } // Iterate until no more segments can be joined bool joined = true; while (joined) { joined = false; // Check if any two segments intersect or are adjacent for (int i = 0; i < segments.Count; i++) { for (int j = i + 1; j < segments.Count; j++) { // Check for intersection bool intersects = false; foreach (var unusedArea1 in segments[i]) { foreach (var unusedArea2 in segments[j]) { if (unusedArea1.i0 <= unusedArea2.i1 && unusedArea1.i1 >= unusedArea2.i0 && unusedArea1.j0 <= unusedArea2.j1 && unusedArea1.j1 >= unusedArea2.j0) { intersects = true; break; } } if (intersects) break; } // Check for adjacency bool adjacent = false; if (!intersects) { foreach (var unusedArea1 in segments[i]) { foreach (var unusedArea2 in segments[j]) { if (unusedArea1.i0 == unusedArea2.i0 && unusedArea1.i1 == unusedArea2.i1 && ((unusedArea1.j1 == unusedArea2.j0 && Math.Abs(unusedArea1.j0 - unusedArea2.j1) == 1) || (unusedArea1.j0 == unusedArea2.j1 && Math.Abs(unusedArea1.j1 - unusedArea2.j0) == 1))) { adjacent = true; break; } if (unusedArea1.j0 == unusedArea2.j0 && unusedArea1.j1 == unusedArea2.j1
以上是如何使用 C# 识别 2D 点云中的凸孔并将其多边形化?的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
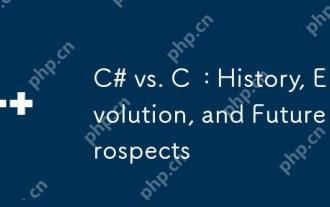
C#和C 的历史与演变各有特色,未来前景也不同。1.C 由BjarneStroustrup在1983年发明,旨在将面向对象编程引入C语言,其演变历程包括多次标准化,如C 11引入auto关键字和lambda表达式,C 20引入概念和协程,未来将专注于性能和系统级编程。2.C#由微软在2000年发布,结合C 和Java的优点,其演变注重简洁性和生产力,如C#2.0引入泛型,C#5.0引入异步编程,未来将专注于开发者的生产力和云计算。
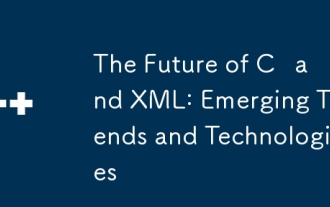
C 和XML的未来发展趋势分别为:1)C 将通过C 20和C 23标准引入模块、概念和协程等新特性,提升编程效率和安全性;2)XML将继续在数据交换和配置文件中占据重要地位,但会面临JSON和YAML的挑战,并朝着更简洁和易解析的方向发展,如XMLSchema1.1和XPath3.1的改进。
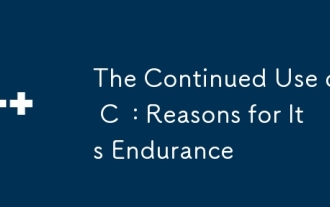
C 持续使用的理由包括其高性能、广泛应用和不断演进的特性。1)高效性能:通过直接操作内存和硬件,C 在系统编程和高性能计算中表现出色。2)广泛应用:在游戏开发、嵌入式系统等领域大放异彩。3)不断演进:自1983年发布以来,C 持续增加新特性,保持其竞争力。
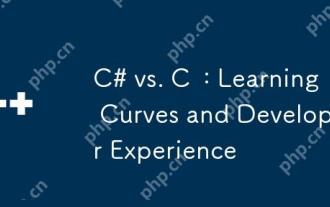
C#和C 的学习曲线和开发者体验有显着差异。 1)C#的学习曲线较平缓,适合快速开发和企业级应用。 2)C 的学习曲线较陡峭,适用于高性能和低级控制的场景。
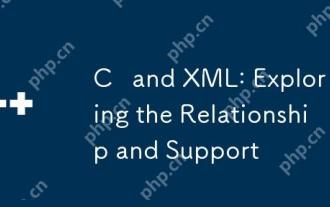
C 通过第三方库(如TinyXML、Pugixml、Xerces-C )与XML交互。1)使用库解析XML文件,将其转换为C 可处理的数据结构。2)生成XML时,将C 数据结构转换为XML格式。3)在实际应用中,XML常用于配置文件和数据交换,提升开发效率。
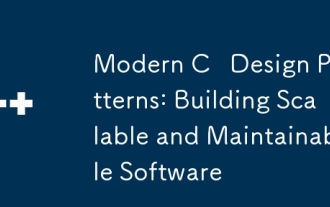
现代C 设计模式利用C 11及以后的新特性实现,帮助构建更灵活、高效的软件。1)使用lambda表达式和std::function简化观察者模式。2)通过移动语义和完美转发优化性能。3)智能指针确保类型安全和资源管理。
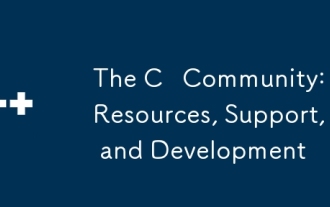
C 学习者和开发者可以从StackOverflow、Reddit的r/cpp社区、Coursera和edX的课程、GitHub上的开源项目、专业咨询服务以及CppCon等会议中获得资源和支持。1.StackOverflow提供技术问题的解答;2.Reddit的r/cpp社区分享最新资讯;3.Coursera和edX提供正式的C 课程;4.GitHub上的开源项目如LLVM和Boost提升技能;5.专业咨询服务如JetBrains和Perforce提供技术支持;6.CppCon等会议有助于职业
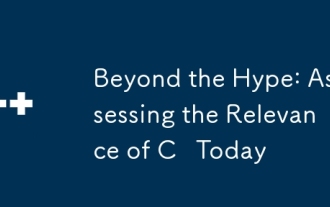
C 在现代编程中仍然具有重要相关性。1)高性能和硬件直接操作能力使其在游戏开发、嵌入式系统和高性能计算等领域占据首选地位。2)丰富的编程范式和现代特性如智能指针和模板编程增强了其灵活性和效率,尽管学习曲线陡峭,但其强大功能使其在今天的编程生态中依然重要。
