单点登录 (SSO) 变得简单
什么是单点登录 (SSO)?
前端单点登录 (SSO) 是一种用户身份验证和授权方法,使用户能够使用一组登录凭据访问多个应用程序或网站,从而消除重复登录和注册。 这改善了用户体验,降低了维护成本,并增强了安全性。
实施单点登录解决方案
前端 SSO 实现有几种关键方法:
基于 Cookie 的 SSO
这种广泛使用的方法利用了浏览器的 cookie 机制。 首次登录中央身份验证页面(例如页面 A)时,会创建一个包含用户数据和过期时间的加密 cookie。 Cookie 的域设置为顶级域(例如 example.com),从而允许在该域内的应用程序之间共享(a.example.com、b.example.com 等)。随后访问其他应用程序会检查此 cookie;如果存在,用户将自动登录;否则,重定向至认证页面。 虽然简单,但这种方法仅限于同域应用程序,面临跨域挑战,并且对 cookie 大小和数量有限制。
示例:设置和检索 cookie。
设置cookie(页面A):
// Generate an encrypted cookie value const encryptedValue = encrypt(userinfo); // Set the cookie document.cookie = `sso_token=${encryptedValue};domain=.example.com;path=/;max-age=86400;`;
检索和使用 cookie(页面 B):
// Retrieve the cookie const cookieValue = document.cookie .split(';') .find((cookie) => cookie.trim().startsWith('sso_token=')) .split('=')[1]; // Decrypt the cookie const userinfo = decrypt(cookieValue); // Log in directly login(userinfo);
基于令牌的 SSO
这种无状态方法涉及在认证中心成功登录后生成加密令牌(包含用户信息和过期时间)。该令牌存储在客户端(localStorage 或 sessionStorage)。 后续应用访问验证token;有效的令牌授予直接访问权限,而无效的令牌则重定向到身份验证中心。 基于令牌的 SSO 支持跨域功能并避免 cookie 限制,但需要额外的存储和网络开销,并且如果令牌被泄露,则会带来安全风险。
示例:存储和验证令牌。
存储令牌(页面 A):
// Generate the token value const token = generateToken(userinfo); // Store the token localStorage.setItem('sso_token', token);
检索和使用令牌(其他页面):
// Retrieve the token const token = localStorage.getItem('sso_token'); // Validate the token const userinfo = verifyToken(token); // Log in directly login(userinfo);
基于 OAuth 2.0 的 SSO
此方法利用 OAuth 2.0 的授权代码流程。 首次登录会触发对认证中心的请求,认证中心返回授权码并重定向到应用程序的回调 URL。 应用程序将此代码交换为存储在客户端的访问和刷新令牌(包含用户数据和过期时间)。 后续应用程序访问会检查有效的访问令牌,如果找到则自动登录,否则重定向到身份验证中心。 虽然遵守 OAuth 2.0 标准并支持各种客户端类型(Web、移动、桌面),但它更加复杂,需要多个请求和重定向。
示例:授权代码流程。
发送授权请求(页面 A):
// Generate an encrypted cookie value const encryptedValue = encrypt(userinfo); // Set the cookie document.cookie = `sso_token=${encryptedValue};domain=.example.com;path=/;max-age=86400;`;
处理回调(页面A):
// Retrieve the cookie const cookieValue = document.cookie .split(';') .find((cookie) => cookie.trim().startsWith('sso_token=')) .split('=')[1]; // Decrypt the cookie const userinfo = decrypt(cookieValue); // Log in directly login(userinfo);
Leapcell:您的首要 Node.js 托管解决方案
Leapcell 是一个用于 Web 托管、异步任务和 Redis 的尖端无服务器平台,提供:
- 多语言支持: Node.js、Python、Go 和 Rust。
- 免费无限项目: 只需支付使用费用。
- 性价比:按需付费,无闲置费用。
- 简化的开发人员体验:直观的 UI、自动化 CI/CD、实时指标。
- 可扩展且高性能:自动扩展,零运营开销。
探索文档并尝试一下!
在 X 上关注我们:@LeapcellHQ
在我们的博客上阅读更多内容
以上是单点登录 (SSO) 变得简单的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
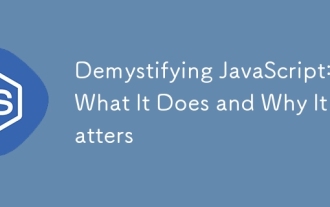
JavaScript是现代Web开发的基石,它的主要功能包括事件驱动编程、动态内容生成和异步编程。1)事件驱动编程允许网页根据用户操作动态变化。2)动态内容生成使得页面内容可以根据条件调整。3)异步编程确保用户界面不被阻塞。JavaScript广泛应用于网页交互、单页面应用和服务器端开发,极大地提升了用户体验和跨平台开发的灵活性。
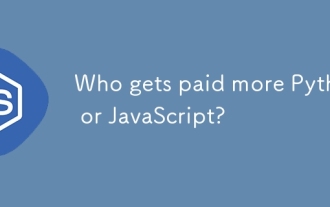
Python和JavaScript开发者的薪资没有绝对的高低,具体取决于技能和行业需求。1.Python在数据科学和机器学习领域可能薪资更高。2.JavaScript在前端和全栈开发中需求大,薪资也可观。3.影响因素包括经验、地理位置、公司规模和特定技能。
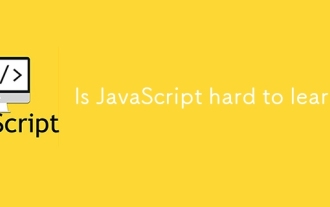
学习JavaScript不难,但有挑战。1)理解基础概念如变量、数据类型、函数等。2)掌握异步编程,通过事件循环实现。3)使用DOM操作和Promise处理异步请求。4)避免常见错误,使用调试技巧。5)优化性能,遵循最佳实践。
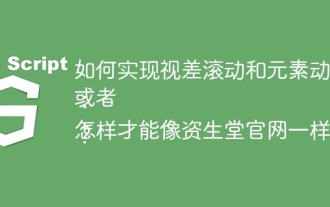
实现视差滚动和元素动画效果的探讨本文将探讨如何实现类似资生堂官网(https://www.shiseido.co.jp/sb/wonderland/)中�...
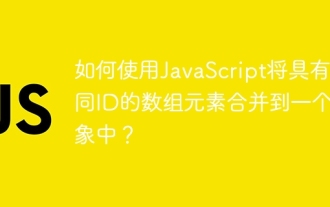
如何在JavaScript中将具有相同ID的数组元素合并到一个对象中?在处理数据时,我们常常会遇到需要将具有相同ID�...
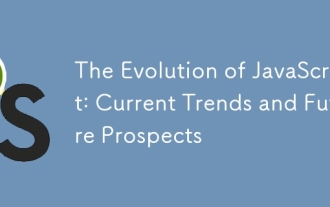
JavaScript的最新趋势包括TypeScript的崛起、现代框架和库的流行以及WebAssembly的应用。未来前景涵盖更强大的类型系统、服务器端JavaScript的发展、人工智能和机器学习的扩展以及物联网和边缘计算的潜力。
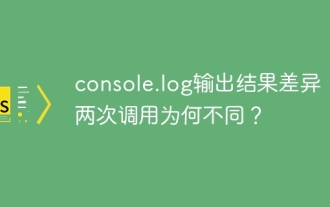
深入探讨console.log输出差异的根源本文将分析一段代码中console.log函数输出结果的差异,并解释其背后的原因。�...
