C语言从0开始
踏上你的 C 编程之旅! 虽然最初令人畏惧,但通过正确的方法掌握 C 语言的基础知识是可以实现的。本指南提供了结构化的介绍,从基本概念到更高级的主题。
目录
- C 基础知识和数据类型
- 用户输入和输出
- 条件语句(包括快捷方式)
- Switch 语句
- 数组:一维和二维
- 嵌套循环
- 函数:结构和用法
- 结构 (
structs
) - 指针
C 基础知识和数据类型
C 程序遵循标准结构并利用各种数据类型作为变量。 一个简单的例子:
#include <stdio.h> int main() { printf("Hello, World!\n"); return 0; }
关键概念:
- 数据类型:
int
:整数(例如,int x = 10;
)float
和double
:浮点数(小数)(例如float pi = 3.14;
)char
:单个字符或 ASCII 代码(例如char letter = 'A';
)bool
:布尔值(真/假)(需要<stdbool.h>
)
// Data Type Examples: int a = 40; // Integer (4 bytes) short int b = 32767; // Short Integer (2 bytes) unsigned int c = 4294967295; // Unsigned Integer (4 bytes) float d = 9.81; // Float (4 bytes) double e = 3.14159; // Double (8 bytes) bool f = true; // Boolean (1 byte) char g = 'e'; // Character (1 byte) char h = 100; // Character (1 byte) char name[] = "Example"; // String (array of characters) // Variable declaration and initialization int age; // Declaration age = 5; // Initialization char letter = 'C'; // Declaration and initialization // Displaying variables printf("You are %d years old\n", age); // Integer printf("Hello %s\n", name); // String printf("Learning %c\n", letter); // Character // Format specifiers: %d (int), %s (string), %c (char), %f (float), %.2f (float to 2 decimal places)
- 运算符:
-
、*
、/
、%
(模)、--
(递减)。 请记住类型转换以获得准确的结果(例如,float z = 5 / (float)2;
)。
用户输入和输出
对于 VS Code 中的用户输入,请使用“终端”选项卡。
int age; char name[25]; // Integer Input printf("Enter your age: "); scanf("%d", &age); printf("You are %d years old\n", age); // String Input (using `fgets` for safer input) printf("Enter your name: "); fgets(name, sizeof(name), stdin); // fgets handles spaces name[strcspn(name, "\n")] = 0; // Remove trailing newline from fgets printf("Hello, %s!\n", name);
C 中区分大小写很重要。使用 toupper()
中的 <ctype.h>
等函数进行不区分大小写的比较。
条件快捷键(三元运算符)
三元运算符提供了一种简洁的方式来编写if-else
语句:
int max = (a > b) ? a : b; // Equivalent to an if-else statement
Switch 语句
高效处理多种情况:
char grade = 'A'; switch (grade) { case 'A': printf("Excellent!\n"); break; case 'B': printf("Good!\n"); break; default: printf("Try again!\n"); }
始终包含一个default
案例。
数组
数组存储相同类型变量的集合:
int numbers[5] = {10, 20, 30, 40, 50}; printf("%d\n", numbers[0]); // Accesses the first element (10) // 2D Array int matrix[2][3] = {{1, 2, 3}, {4, 5, 6}}; // Array of Strings char cars[][10] = {"BMW", "Tesla", "Toyota"};
嵌套循环
循环中的循环,对于处理多维数据很有用:(为简洁起见,省略示例,但使用嵌套 for
循环可以轻松构建)。
功能
函数促进代码可重用性:
void greet(char name[]) { printf("Hello, %s!\n", name); } int main() { greet("Alice"); return 0; }
结构 (structs
)
对相关变量进行分组:
struct Player { char name[50]; int score; }; struct Player player1 = {"Bob", 150}; printf("Name: %s, Score: %d\n", player1.name, player1.score);
指针
存储内存地址的变量:
#include <stdio.h> int main() { printf("Hello, World!\n"); return 0; }
指针对于动态内存分配至关重要。 本指南提供了坚实的基础。 坚持练习是掌握 C 编程的关键。
以上是C语言从0开始的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
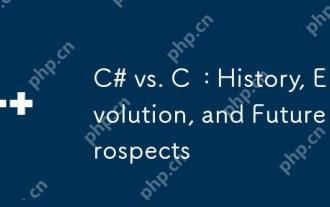
C#和C 的历史与演变各有特色,未来前景也不同。1.C 由BjarneStroustrup在1983年发明,旨在将面向对象编程引入C语言,其演变历程包括多次标准化,如C 11引入auto关键字和lambda表达式,C 20引入概念和协程,未来将专注于性能和系统级编程。2.C#由微软在2000年发布,结合C 和Java的优点,其演变注重简洁性和生产力,如C#2.0引入泛型,C#5.0引入异步编程,未来将专注于开发者的生产力和云计算。
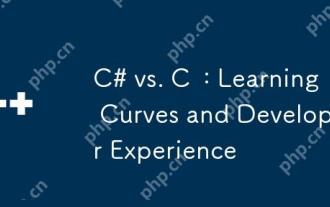
C#和C 的学习曲线和开发者体验有显着差异。 1)C#的学习曲线较平缓,适合快速开发和企业级应用。 2)C 的学习曲线较陡峭,适用于高性能和低级控制的场景。
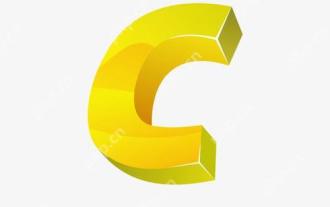
静态分析在C 中的应用主要包括发现内存管理问题、检查代码逻辑错误和提高代码安全性。1)静态分析可以识别内存泄漏、双重释放和未初始化指针等问题。2)它能检测未使用变量、死代码和逻辑矛盾。3)静态分析工具如Coverity能发现缓冲区溢出、整数溢出和不安全API调用,提升代码安全性。
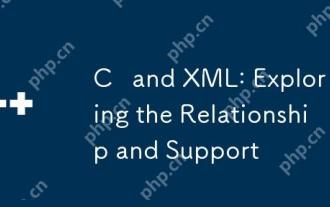
C 通过第三方库(如TinyXML、Pugixml、Xerces-C )与XML交互。1)使用库解析XML文件,将其转换为C 可处理的数据结构。2)生成XML时,将C 数据结构转换为XML格式。3)在实际应用中,XML常用于配置文件和数据交换,提升开发效率。
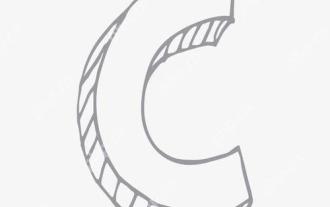
使用C 中的chrono库可以让你更加精确地控制时间和时间间隔,让我们来探讨一下这个库的魅力所在吧。C 的chrono库是标准库的一部分,它提供了一种现代化的方式来处理时间和时间间隔。对于那些曾经饱受time.h和ctime折磨的程序员来说,chrono无疑是一个福音。它不仅提高了代码的可读性和可维护性,还提供了更高的精度和灵活性。让我们从基础开始,chrono库主要包括以下几个关键组件:std::chrono::system_clock:表示系统时钟,用于获取当前时间。std::chron
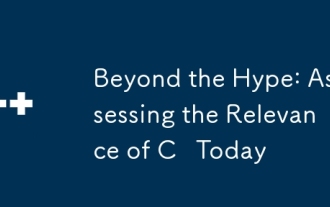
C 在现代编程中仍然具有重要相关性。1)高性能和硬件直接操作能力使其在游戏开发、嵌入式系统和高性能计算等领域占据首选地位。2)丰富的编程范式和现代特性如智能指针和模板编程增强了其灵活性和效率,尽管学习曲线陡峭,但其强大功能使其在今天的编程生态中依然重要。
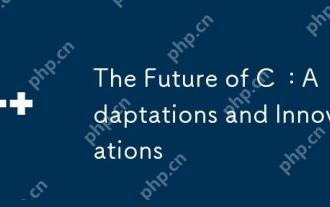
C 的未来将专注于并行计算、安全性、模块化和AI/机器学习领域:1)并行计算将通过协程等特性得到增强;2)安全性将通过更严格的类型检查和内存管理机制提升;3)模块化将简化代码组织和编译;4)AI和机器学习将促使C 适应新需求,如数值计算和GPU编程支持。
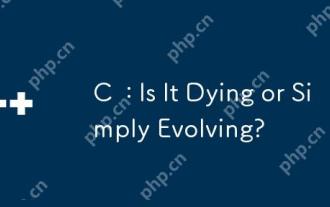
1)c relevantduetoItsAverity and效率和效果临界。2)theLanguageIsconTinuellyUped,withc 20introducingFeaturesFeaturesLikeTuresLikeSlikeModeLeslikeMeSandIntIneStoImproutiMimproutimprouteverusabilityandperformance.3)
