如何使用 jQuery AJAX 将对象数组传递到 MVC 控制器?
使用 jQuery AJAX 将对象数组发送到 MVC 控制器
使用 jQuery 的 ajax()
方法将对象数组发送到 MVC 控制器时,一个常见问题是在控制器中接收数组的 null
值。 即使控制器参数正确定义为 List<T>
.
解决方案涉及两个关键调整:
-
指定 JSON 数据类型:
ajax()
调用必须显式将contentType
和dataType
设置为'application/json; charset=utf-8'
。 -
使用 JSON.stringify() 序列化数据: JavaScript 对象数组需要使用
JSON.stringify()
转换为 JSON 字符串。 至关重要的是,这个字符串应该将数组封装在属性中(例如“things”)。
这是实现这些更改的更正代码:
$(document).ready(function () { const things = [ { id: 1, color: 'yellow' }, { id: 2, color: 'blue' }, { id: 3, color: 'red' } ]; const data = JSON.stringify({ things: things }); $.ajax({ contentType: 'application/json; charset=utf-8', dataType: 'json', type: 'POST', url: '/Home/PassThings', data: data, success: function () { $('#result').html('"PassThings()" successfully called.'); }, error: function (response) { $('#result').html(response.responseText); // Display error details } }); });
以及对应的C#控制器方法:
public void PassThings(List<Thing> things) { // Access the 'things' array here } public class Thing { public int Id { get; set; } public string Color { get; set; } }
通过执行这些步骤,您将通过 jQuery AJAX 成功将对象数组传输到 MVC 控制器方法。 请注意 AJAX 调用中从 failure
到 error
的更改,以便更好地处理错误,显示响应文本以进行调试。
以上是如何使用 jQuery AJAX 将对象数组传递到 MVC 控制器?的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
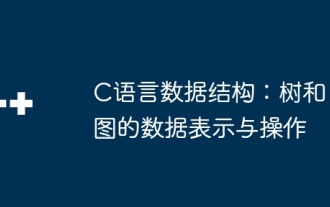
C语言数据结构:树和图的数据表示与操作树是一个层次结构的数据结构由节点组成,每个节点包含一个数据元素和指向其子节点的指针二叉树是一种特殊类型的树,其中每个节点最多有两个子节点数据表示structTreeNode{intdata;structTreeNode*left;structTreeNode*right;};操作创建树遍历树(先序、中序、后序)搜索树插入节点删除节点图是一个集合的数据结构,其中的元素是顶点,它们通过边连接在一起边可以是带权或无权的数据表示邻
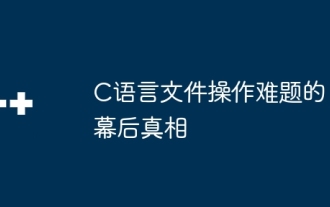
文件操作难题的真相:文件打开失败:权限不足、路径错误、文件被占用。数据写入失败:缓冲区已满、文件不可写、磁盘空间不足。其他常见问题:文件遍历缓慢、文本文件编码不正确、二进制文件读取错误。
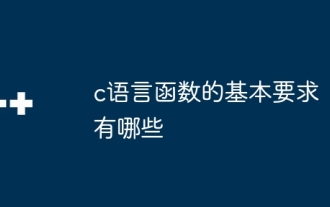
C语言函数是代码模块化和程序搭建的基础。它们由声明(函数头)和定义(函数体)组成。C语言默认使用值传递参数,但也可使用地址传递修改外部变量。函数可以有返回值或无返回值,返回值类型必须与声明一致。函数命名应清晰易懂,使用驼峰或下划线命名法。遵循单一职责原则,保持函数简洁性,以提高可维护性和可读性。
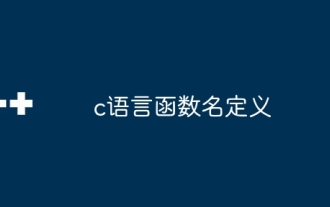
C语言函数名定义包括:返回值类型、函数名、参数列表和函数体。函数名应清晰、简洁、统一风格,避免与关键字冲突。函数名具有作用域,可在声明后使用。函数指针允许将函数作为参数传递或赋值。常见错误包括命名冲突、参数类型不匹配和未声明的函数。性能优化重点在函数设计和实现上,而清晰、易读的代码至关重要。
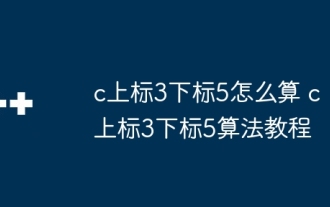
C35 的计算本质上是组合数学,代表从 5 个元素中选择 3 个的组合数,其计算公式为 C53 = 5! / (3! * 2!),可通过循环避免直接计算阶乘以提高效率和避免溢出。另外,理解组合的本质和掌握高效的计算方法对于解决概率统计、密码学、算法设计等领域的许多问题至关重要。
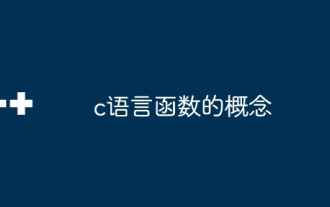
C语言函数是可重复利用的代码块,它接收输入,执行操作,返回结果,可将代码模块化提高可复用性,降低复杂度。函数内部机制包含参数传递、函数执行、返回值,整个过程涉及优化如函数内联。编写好的函数遵循单一职责原则、参数数量少、命名规范、错误处理。指针与函数结合能实现更强大的功能,如修改外部变量值。函数指针将函数作为参数传递或存储地址,用于实现动态调用函数。理解函数特性和技巧是编写高效、可维护、易理解的C语言程序的关键。
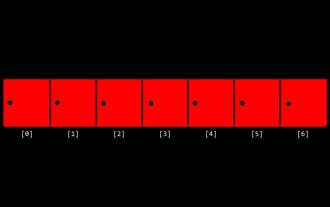
算法是解决问题的指令集,其执行速度和内存占用各不相同。编程中,许多算法都基于数据搜索和排序。本文将介绍几种数据检索和排序算法。线性搜索假设有一个数组[20,500,10,5,100,1,50],需要查找数字50。线性搜索算法会逐个检查数组中的每个元素,直到找到目标值或遍历完整个数组。算法流程图如下:线性搜索的伪代码如下:检查每个元素:如果找到目标值:返回true返回falseC语言实现:#include#includeintmain(void){i
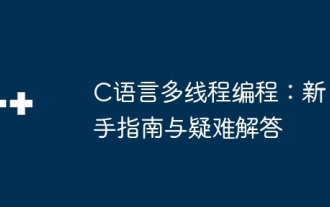
C语言多线程编程指南:创建线程:使用pthread_create()函数,指定线程ID、属性和线程函数。线程同步:通过互斥锁、信号量和条件变量防止数据竞争。实战案例:使用多线程计算斐波那契数,将任务分配给多个线程并同步结果。疑难解答:解决程序崩溃、线程停止响应和性能瓶颈等问题。
