轻松的 React 动画:Framer 运动指南
TL;DR: 这篇博文提供了使用 React 动画库 Framer Motion 的全面指南。它涵盖了运动组件、变体和过渡等关键概念,并提供了创建淡入淡出按钮、滑入侧边栏、可拖动模式和卡片翻转动画的实际示例。
作为前端开发人员,我们的首要任务是创建让用户参与的 Web 应用程序。这可以通过创建交互式页面并提供更好的用户体验来实现。
动画使您的页面具有互动性;它们引导用户并使交互变得有趣。页面上的微小视觉动作,例如用户交互或事件或页面导航,给人一种活泼的感觉,就像我们正在与一个响应我们的动作的生物进行交互。
动画,简单来说,是一种通过在交互或某些事件上随着时间的推移更新元素的属性或尺寸来视觉上改变元素的方式。例如,显示您的操作正在进行中的加载指示器。
有两种方法可以为网页上的元素设置动画(两种更改元素属性的方法)。
- 通过 CSS,Animate.css 等库提供了一组可以添加到 HTML 元素的动画类。
- 通过 JavaScript,像 Framer Motion 这样的库可以在运行时通过代码操纵 DOM 元素的属性。
在本文中,我们将探索 Framer Motion,这是最流行的动画库之一。它提供简单性和灵活性,旨在与 React 等现代前端框架配合使用。
为什么选择 Framer Motion?
Framer Motion 是一个用于 React 的生产就绪动画库,它通过其声明性语法创建简单的动画(例如过渡)和复杂的基于手势的交互。它的特点是:
- 易于使用: Framer Motion 凭借其直观的 API 和方法,非常简单且易于使用。
- 灵活性:它可用于创建复杂的动画,如平移、拖动、捏合,或简单的动画,如淡入淡出、过渡。
- 性能:运动组件针对性能进行了优化,因为它们在 React 生命周期之外渲染,以平稳运行并确保无缝的用户体验。
- 社区和支持:广泛的文档、大量示例以及社区的广泛采用使入门变得更加容易。
Framer Motion 入门
使用 npm 或 yarn 包管理器将 Framer Motion 库添加到您的项目中。
npm install framer-motion
或者
npm install framer-motion
加载依赖项后,您可以将其包含在项目中以创建交互式动画。
yarn add framer-motion
基本概念
运动组件:
Framer Motion 附带一系列运动组件来创建 120fps 动画。它提供手势支持,其中包含所有可以使用的特殊 React 组件的 HTML 元素(如motion.div)和常见的 SVG 元素(如motion.square)。
// On Client side import { motion } from "motion/react" // On Server-side import * as motion from "motion/react-client"
道具和 API:
Framer Motion 提供了一系列 API 作为 props,例如定义动画行为的 initial、animate 和 exit。
<motion.div className="card" />
Initial 属性在组件挂载时触发,animate 在组件更新时触发,exit 属性在组件卸载时触发。有关更多详细信息,请参阅完整的 Framer Motion 动画指南。
运动组件独立于 React 生命周期或渲染周期,以提高性能。因此,我们应该依赖 React 状态来实现动画,而不是使用运动值来更新样式而不触发重新渲染。
<motion.button initial={{opacity: 0}} animate={{opacity: 1}} transition={{duration: 1}} exit={{opacity: 0}} > Click Me </motion.button>
变体包括:
- listVariants: 定义整个列表的动画行为,我们在将访问其触发时的属性的道具上传递变体值。 初始=“隐藏”和动画=“可见”。 staggerChildren 确保子元素按顺序动画。
- itemVariants: 定义列表项的动画行为。
- motion.ul 和 motion.li 组件继承变体以创建协调的动画。
自定义组件:任何 React 组件都可以通过将其传递给 motion.create() 函数来转换为运动组件。
import { motion, useMotionValue } from "framer-motion"; const MotionState = () => { const xPosition = useMotionValue(0); useEffect(() => { // It won’t trigger a re-render on the component const interval = setInterval(() => { xPosition.set(xPosition.get() + 100); }, 1000); return () => clearInterval(interval); }, []); return ( <motion.div > <p>In the previous example, the <strong>motion.div</strong> element will be translated by 100px on the x position (horizontally, translateX(100px)) at an interval of 1s.</p> <p><strong>Variants:</strong> framer-motion provides support for the variants, which allows the reuse of animation configurations across multiple elements.<br> </p> <pre class="brush:php;toolbar:false">const AnimatedList = () => { const listVariants = { hidden: { opacity: 0, y: 20 }, visible: { opacity: 1, y: 0, transition: { staggerChildren: 0.2, }, }, }; const itemVariants = { visible: { opacity: 1 }, hidden: { opacity: 0 }, }; return ( <motion.ul initial="hidden" animate="visible" variants={listVariants}> {[1, 2, 3].map((item) => ( <motion.li key={item} variants={itemVariants}> Item {item} </motion.li> ))} </motion.ul> ); };
默认情况下,所有运动道具在传递给 React 组件时都会被过滤掉。动画将应用于组件,但您无法访问 React 中的 props。
要访问运动道具,请在创建运动组件时传递标志 forwardMotionProps: true。
const ReactComponent = (props) => { return <button {...props}>ClickMe>/button>; }; const MotionComponent = motion.create(ReactComponent); const FadingButton2 = () => { return ( <MotionComponent initial={{ opacity: 0 }} animate={{ opacity: 1 }} exit={{ opacity: 0 }} transition={{ duration: 3 }} > Click Me </MotionComponent> ); };
motion.create() 函数还接受一个字符串,该字符串将创建自定义 DOM 元素的运动组件。
const MotionComponent = motion.create(ReactComponent, { forwardMotionProps: true, });
注意:避免在 React 生命周期方法中使用 motion.create(),因为这会每次触发生命周期方法时都会创建一个新组件。
现在您已经了解了 Framer Motion 的工作原理及其 API,让我们看一些如何将其用于常见动画的示例。
示例
褪色按钮
npm install framer-motion
- initial: 当元素不是视口时,设置按钮 opacity:0 的初始状态。
- animate: 当元素位于视口中时,将按钮的状态设置为 opacity:1。
- transition: 配置动画过渡;该按钮将需要一秒钟的时间从 opacity:0 变为 opacity:1
- exit: 设置元素离开视口时按钮的状态。
exit 属性仅在封装在 AnimatePresence 组件中时才生效。
yarn add framer-motion
AnimatePresence 影响直接子组件,这些子组件是从 React 组件树中删除的运动组件。
这可能是当组件根据生命周期更改(安装、更新、卸载)进行更新时
// On Client side import { motion } from "motion/react" // On Server-side import * as motion from "motion/react-client"
其键更改
<motion.div className="card" />
孩子被添加到列表中或从列表中删除。
<motion.button initial={{opacity: 0}} animate={{opacity: 1}} transition={{duration: 1}} exit={{opacity: 0}} > Click Me </motion.button>
滑入式侧边栏
import { motion, useMotionValue } from "framer-motion"; const MotionState = () => { const xPosition = useMotionValue(0); useEffect(() => { // It won’t trigger a re-render on the component const interval = setInterval(() => { xPosition.set(xPosition.get() + 100); }, 1000); return () => clearInterval(interval); }, []); return ( <motion.div > <p>In the previous example, the <strong>motion.div</strong> element will be translated by 100px on the x position (horizontally, translateX(100px)) at an interval of 1s.</p> <p><strong>Variants:</strong> framer-motion provides support for the variants, which allows the reuse of animation configurations across multiple elements.<br> </p> <pre class="brush:php;toolbar:false">const AnimatedList = () => { const listVariants = { hidden: { opacity: 0, y: 20 }, visible: { opacity: 1, y: 0, transition: { staggerChildren: 0.2, }, }, }; const itemVariants = { visible: { opacity: 1 }, hidden: { opacity: 0 }, }; return ( <motion.ul initial="hidden" animate="visible" variants={listVariants}> {[1, 2, 3].map((item) => ( <motion.li key={item} variants={itemVariants}> Item {item} </motion.li> ))} </motion.ul> ); };
过渡 道具在动画中起着至关重要的作用。它们控制动画随时间的进展方式。 Framer Motion 支持多种属性以实现流畅的动画。
- 持续时间: 动画的长度(以秒为单位)
- 延迟: 延迟动画的开始(以秒为单位)
- ease: 一组缓动函数,提倡动画如何进行(‘ease’、‘easeIn’、‘easeInOut’)
可拖动模态
Framer Motion 还支持悬停、点击和拖动等手势的交互式动画。
const ReactComponent = (props) => { return <button {...props}>ClickMe>/button>; }; const MotionComponent = motion.create(ReactComponent); const FadingButton2 = () => { return ( <MotionComponent initial={{ opacity: 0 }} animate={{ opacity: 1 }} exit={{ opacity: 0 }} transition={{ duration: 3 }} > Click Me </MotionComponent> ); };
- Page: 用运动动画包裹子组件。
- Initial, animate, & exit: 处理动画的出现和消失页面导航上的组件。
结论
感谢您的阅读! Framer Motion 是一个功能强大的动画库,可以更轻松地向 React 组件添加令人惊叹的动画。它可以帮助您创建简单的动画来处理复杂的基于手势的交互。 Framer Motion 为您的 React 应用程序添加交互有无限的可能性。
Essential Studio® 的新版本可在许可证和下载页面上供现有客户使用。如果您是新用户,请注册我们的 30 天免费试用版以探索我们的功能。
请随时通过我们的支持论坛、支持门户或反馈门户与我们联系。我们随时为您提供帮助!
相关博客
- 可实现流畅文档处理的前 5 个 React PDF 查看器
- 2025 年排名前 5 的 React 图表库
- Vite.js:构建更快的前端
- React 的 RxJS:解锁反应状态
以上是轻松的 React 动画:Framer 运动指南的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
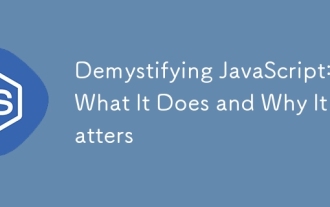
JavaScript是现代Web开发的基石,它的主要功能包括事件驱动编程、动态内容生成和异步编程。1)事件驱动编程允许网页根据用户操作动态变化。2)动态内容生成使得页面内容可以根据条件调整。3)异步编程确保用户界面不被阻塞。JavaScript广泛应用于网页交互、单页面应用和服务器端开发,极大地提升了用户体验和跨平台开发的灵活性。
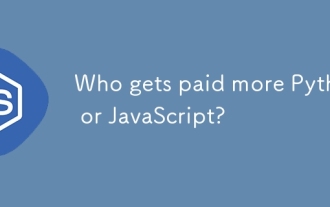
Python和JavaScript开发者的薪资没有绝对的高低,具体取决于技能和行业需求。1.Python在数据科学和机器学习领域可能薪资更高。2.JavaScript在前端和全栈开发中需求大,薪资也可观。3.影响因素包括经验、地理位置、公司规模和特定技能。
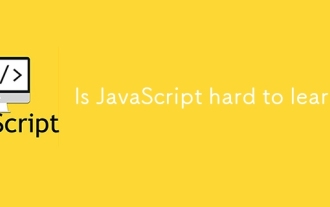
学习JavaScript不难,但有挑战。1)理解基础概念如变量、数据类型、函数等。2)掌握异步编程,通过事件循环实现。3)使用DOM操作和Promise处理异步请求。4)避免常见错误,使用调试技巧。5)优化性能,遵循最佳实践。
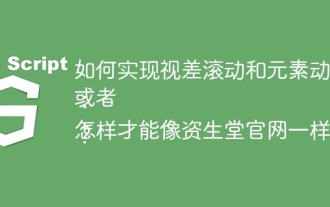
实现视差滚动和元素动画效果的探讨本文将探讨如何实现类似资生堂官网(https://www.shiseido.co.jp/sb/wonderland/)中�...
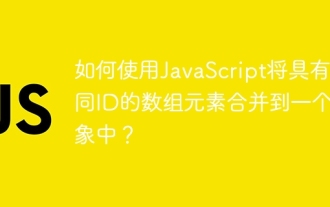
如何在JavaScript中将具有相同ID的数组元素合并到一个对象中?在处理数据时,我们常常会遇到需要将具有相同ID�...
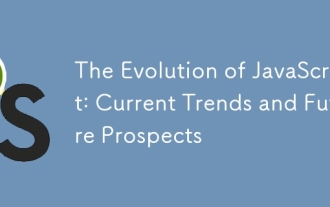
JavaScript的最新趋势包括TypeScript的崛起、现代框架和库的流行以及WebAssembly的应用。未来前景涵盖更强大的类型系统、服务器端JavaScript的发展、人工智能和机器学习的扩展以及物联网和边缘计算的潜力。
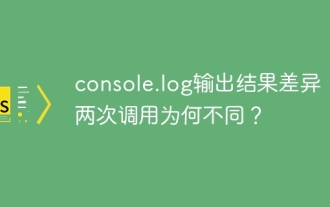
深入探讨console.log输出差异的根源本文将分析一段代码中console.log函数输出结果的差异,并解释其背后的原因。�...
