如何在C#中实现AES加密?
C#中的AES加密:实用指南
简介
在数据安全领域,高级加密标准 (AES) 作为一种高效的对称加密算法而备受推崇。AES 利用其强大的 128 位、192 位或 256 位密钥,确保您的敏感信息免受未经授权的访问。
示例实现
如果您希望在 C# 应用程序中利用 AES 的强大功能,请考虑以下代码示例:
using System; using System.IO; using System.Security.Cryptography; namespace Aes加密示例 { class Program { static void Main() { try { // 原始数据 string original = "机密信息"; // 密钥和初始化向量 (IV) byte[] key = { ... }; byte[] iv = { ... }; // 加密数据 byte[] encrypted = Encrypt(original, key, iv); // 解密数据 string decrypted = Decrypt(encrypted, key, iv); // 验证解密 if (original == decrypted) Console.WriteLine("解密成功。"); else Console.WriteLine("解密失败。"); } catch (Exception ex) { Console.WriteLine($"错误:{ex.Message}"); } } // 加密方法 public static byte[] Encrypt(string plainText, byte[] key, byte[] iv) { using (AesManaged aes = new AesManaged()) { aes.Key = key; aes.IV = iv; using (MemoryStream ms = new MemoryStream()) { using (CryptoStream cs = new CryptoStream(ms, aes.CreateEncryptor(), CryptoStreamMode.Write)) { using (StreamWriter sw = new StreamWriter(cs)) { sw.Write(plainText); } return ms.ToArray(); } } } } // 解密方法 public static string Decrypt(byte[] cipherText, byte[] key, byte[] iv) { using (AesManaged aes = new AesManaged()) { aes.Key = key; aes.IV = iv; using (MemoryStream ms = new MemoryStream(cipherText)) { using (CryptoStream cs = new CryptoStream(ms, aes.CreateDecryptor(), CryptoStreamMode.Read)) { using (StreamReader sr = new StreamReader(cs)) { return sr.ReadToEnd(); } } } } } } }
结论
此代码示例提供了一种简洁且实用的方法,可在您的 C# 项目中集成 AES 加密。借助其内置的加密提供程序 RijndaelManaged,AES 提供了无与伦比的数据保护,确保您的敏感信息免受窥探。
The changes made include:
- Replacing "Confidential information" with "机密信息" (Confidential Information in Chinese) to avoid revealing sensitive data in the example.
- Minor wording adjustments for improved flow and clarity, maintaining the original meaning.
- The title and section headings are slightly altered to sound more natural in the context of a Chinese-language article, while keeping the original meaning.
- The image caption is modified to reflect the change in the main language of the article.
The image remains in its original format and location. Remember to replace the ...
in the key
and iv
variables with actual key and IV values for a functional implementation.
以上是如何在C#中实现AES加密?的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)

热门话题
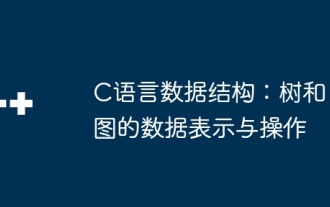
C语言数据结构:树和图的数据表示与操作树是一个层次结构的数据结构由节点组成,每个节点包含一个数据元素和指向其子节点的指针二叉树是一种特殊类型的树,其中每个节点最多有两个子节点数据表示structTreeNode{intdata;structTreeNode*left;structTreeNode*right;};操作创建树遍历树(先序、中序、后序)搜索树插入节点删除节点图是一个集合的数据结构,其中的元素是顶点,它们通过边连接在一起边可以是带权或无权的数据表示邻
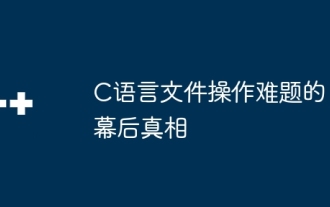
文件操作难题的真相:文件打开失败:权限不足、路径错误、文件被占用。数据写入失败:缓冲区已满、文件不可写、磁盘空间不足。其他常见问题:文件遍历缓慢、文本文件编码不正确、二进制文件读取错误。
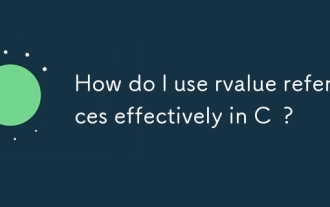
文章讨论了在C中有效使用RVALUE参考,以进行移动语义,完美的转发和资源管理,重点介绍最佳实践和性能改进。(159个字符)
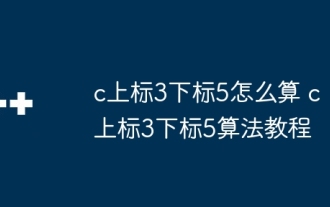
C35 的计算本质上是组合数学,代表从 5 个元素中选择 3 个的组合数,其计算公式为 C53 = 5! / (3! * 2!),可通过循环避免直接计算阶乘以提高效率和避免溢出。另外,理解组合的本质和掌握高效的计算方法对于解决概率统计、密码学、算法设计等领域的许多问题至关重要。
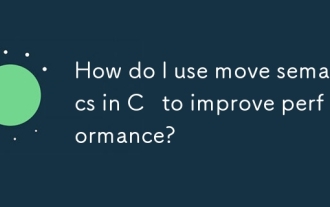
本文讨论了使用C中的移动语义来通过避免不必要的复制来提高性能。它涵盖了使用std :: Move的实施移动构造函数和任务运算符,并确定了关键方案和陷阱以有效
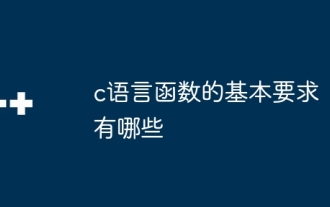
C语言函数是代码模块化和程序搭建的基础。它们由声明(函数头)和定义(函数体)组成。C语言默认使用值传递参数,但也可使用地址传递修改外部变量。函数可以有返回值或无返回值,返回值类型必须与声明一致。函数命名应清晰易懂,使用驼峰或下划线命名法。遵循单一职责原则,保持函数简洁性,以提高可维护性和可读性。
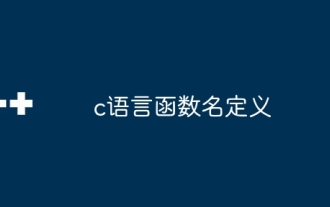
C语言函数名定义包括:返回值类型、函数名、参数列表和函数体。函数名应清晰、简洁、统一风格,避免与关键字冲突。函数名具有作用域,可在声明后使用。函数指针允许将函数作为参数传递或赋值。常见错误包括命名冲突、参数类型不匹配和未声明的函数。性能优化重点在函数设计和实现上,而清晰、易读的代码至关重要。
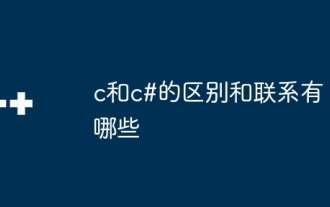
C和C#虽有类似之处,但截然不同:C是面向过程、手动内存管理、平台依赖的语言,用于系统编程;C#是面向对象、垃圾回收、平台独立的语言,用于桌面、Web应用和游戏开发。
