如何动态编译和执行C#代码片段?
C#代码片段的动态编译与执行
本文介绍如何动态编译和执行来自文本文件或输入流的C#代码片段。推荐使用C#和.NET语言中的代码文档对象模型(CodeDom)来实现此功能。
CodeDom允许您将代码作为对象进行操作,并利用编译器动态编译代码。以下代码片段展示了这种方法:
using System.CodeDom.Compiler; using Microsoft.CSharp; using System.Reflection; using System.Collections.Generic; using System.Linq; class Program { static void Main(string[] args) { var csc = new CSharpCodeProvider(new Dictionary<string, string>() { { "CompilerVersion", "v3.5" } }); var parameters = new CompilerParameters(new[] { "mscorlib.dll", "System.Core.dll" }, "foo.exe", true); parameters.GenerateExecutable = true; CompilerResults results = csc.CompileAssemblyFromSource(parameters, @"using System.Linq; class Program { public static void Main(string[] args) { var q = from i in Enumerable.Range(1,100) where i % 2 == 0 select i; foreach(var item in q) { Console.WriteLine(item); } } }"); // 检查错误 results.Errors.Cast<CompilerError>().ToList().ForEach(error => Console.WriteLine(error.ErrorText)); // 动态加载并执行已编译的程序集 if (results.Errors.Count == 0) { Assembly assembly = results.CompiledAssembly; var type = assembly.GetTypes()[0]; var method = type.GetMethod("Main"); method.Invoke(null, new object[] { new string[] { } }); } } }
在这个例子中,CompilationParameters
和CSharpCodeProvider
用于动态编译代码。如果没有编译错误,则动态加载已编译的程序集并调用其Main
方法。 为了更完整地展示结果,已将示例代码修改为打印偶数结果。
这种方法为动态编译和执行C#代码片段提供了一种灵活且通用的解决方案,允许您实现各种场景,例如脚本执行或动态代码生成。
以上是如何动态编译和执行C#代码片段?的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
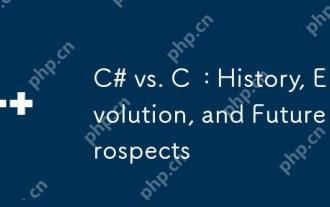
C#和C 的历史与演变各有特色,未来前景也不同。1.C 由BjarneStroustrup在1983年发明,旨在将面向对象编程引入C语言,其演变历程包括多次标准化,如C 11引入auto关键字和lambda表达式,C 20引入概念和协程,未来将专注于性能和系统级编程。2.C#由微软在2000年发布,结合C 和Java的优点,其演变注重简洁性和生产力,如C#2.0引入泛型,C#5.0引入异步编程,未来将专注于开发者的生产力和云计算。
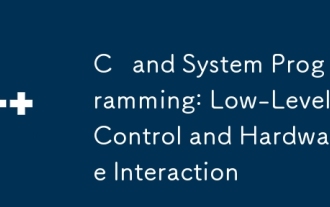
C 适合系统编程和硬件交互,因为它提供了接近硬件的控制能力和面向对象编程的强大特性。1)C 通过指针、内存管理和位操作等低级特性,实现高效的系统级操作。2)硬件交互通过设备驱动程序实现,C 可以编写这些驱动程序,处理与硬件设备的通信。
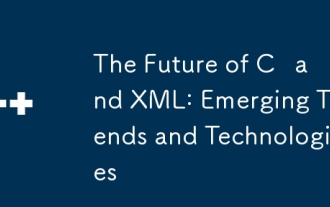
C 和XML的未来发展趋势分别为:1)C 将通过C 20和C 23标准引入模块、概念和协程等新特性,提升编程效率和安全性;2)XML将继续在数据交换和配置文件中占据重要地位,但会面临JSON和YAML的挑战,并朝着更简洁和易解析的方向发展,如XMLSchema1.1和XPath3.1的改进。
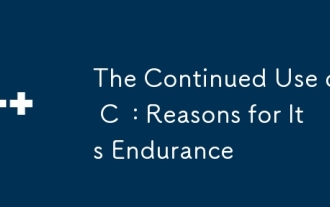
C 持续使用的理由包括其高性能、广泛应用和不断演进的特性。1)高效性能:通过直接操作内存和硬件,C 在系统编程和高性能计算中表现出色。2)广泛应用:在游戏开发、嵌入式系统等领域大放异彩。3)不断演进:自1983年发布以来,C 持续增加新特性,保持其竞争力。
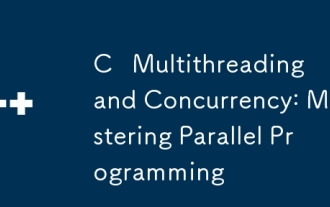
C 多线程和并发编程的核心概念包括线程的创建与管理、同步与互斥、条件变量、线程池、异步编程、常见错误与调试技巧以及性能优化与最佳实践。1)创建线程使用std::thread类,示例展示了如何创建并等待线程完成。2)同步与互斥使用std::mutex和std::lock_guard保护共享资源,避免数据竞争。3)条件变量通过std::condition_variable实现线程间的通信和同步。4)线程池示例展示了如何使用ThreadPool类并行处理任务,提高效率。5)异步编程使用std::as
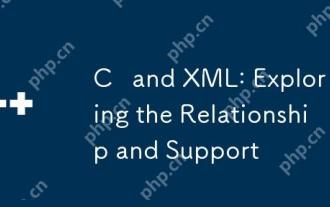
C 通过第三方库(如TinyXML、Pugixml、Xerces-C )与XML交互。1)使用库解析XML文件,将其转换为C 可处理的数据结构。2)生成XML时,将C 数据结构转换为XML格式。3)在实际应用中,XML常用于配置文件和数据交换,提升开发效率。
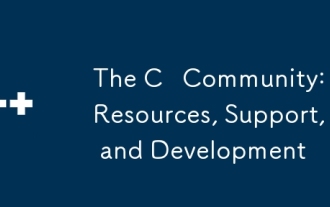
C 学习者和开发者可以从StackOverflow、Reddit的r/cpp社区、Coursera和edX的课程、GitHub上的开源项目、专业咨询服务以及CppCon等会议中获得资源和支持。1.StackOverflow提供技术问题的解答;2.Reddit的r/cpp社区分享最新资讯;3.Coursera和edX提供正式的C 课程;4.GitHub上的开源项目如LLVM和Boost提升技能;5.专业咨询服务如JetBrains和Perforce提供技术支持;6.CppCon等会议有助于职业
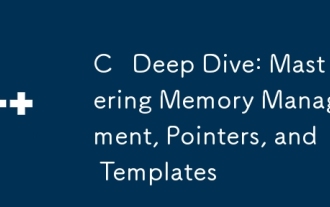
C 的内存管理、指针和模板是核心特性。1.内存管理通过new和delete手动分配和释放内存,需注意堆和栈的区别。2.指针允许直接操作内存地址,使用需谨慎,智能指针可简化管理。3.模板实现泛型编程,提高代码重用性和灵活性,需理解类型推导和特化。
