从邮政请求中读取jax-rs客户端中的响应主体
>从邮政请求中读取响应主体,
>本节详细介绍了如何从jax-rs客户端中的邮政请求中读取响应主体。 核心方法涉及使用ClientResponse
方法返回的对象。 该对象提供对响应状态代码和实体(响应主体)的访问。 特定方法取决于响应主体的内容类型。 对于常见的JSON响应,您需要使用JSON处理库(例如Jackson或Gson。 您还需要将杰克逊依赖性包括在项目中。invoke()
WebTarget
有效地解析JSON响应主体
之类的注释将JSON密钥准确地映射到Java字段。
import javax.ws.rs.client.Client; import javax.ws.rs.client.ClientBuilder; import javax.ws.rs.client.Entity; import javax.ws.rs.client.WebTarget; import javax.ws.rs.core.MediaType; import javax.ws.rs.core.Response; import com.fasterxml.jackson.databind.ObjectMapper; //Jackson library public class ReadPostResponseBody { public static void main(String[] args) { Client client = ClientBuilder.newClient(); WebTarget target = client.target("http://your-api-endpoint.com/your-resource"); // Create a sample request entity (replace with your actual data) String requestBody = "{\"key1\":\"value1\", \"key2\":\"value2\"}"; Response response = target.request(MediaType.APPLICATION_JSON).post(Entity.json(requestBody)); if (response.getStatus() == 200) { //Check for successful response try { ObjectMapper objectMapper = new ObjectMapper(); YourResponseObject responseObject = objectMapper.readValue(response.readEntity(String.class), YourResponseObject.class); //Process responseObject System.out.println("Response: " + responseObject); } catch (Exception e) { e.printStackTrace(); } } else { System.err.println("Request failed with status code: " + response.getStatus()); } response.close(); client.close(); } //Define your response object structure public static class YourResponseObject { // Your class fields here } }
"http://your-api-endpoint.com/your-resource"
强大错误处理的最佳实践至关重要。 始终检查服务器返回的HTTP状态代码。 2XX状态代码表示成功,而其他代码表示错误。 您的代码应以不同的方式处理这些。 例如:YourResponseObject
处理响应主体正常。
objectMapper.readValue()
@JsonProperty
这些错误通常表明请求的问题(例如,输入不良)。记录错误并可能提供用户友好的反馈。
- 5xx(服务器错误):
- 这些指示服务器端上的问题。 登录错误并考虑在延迟后重试该请求,或提醒管理员。 >第一部分中的示例演示了200个状态代码的基本检查。 您应该将其扩展以根据您的API规范处理更广泛的状态代码。 使用
- >语句或一系列语句来优雅地管理不同的状态代码。处理潜在的异常
- >块中以处理潜在问题,例如:>
IOException
:网络问题,连接超时。JSONException
:在JSON解析过程中出现错误(如果使用抛出此抛出的库)。ProcessingException
:自定义例外,您可能会定义为特定的错误场景。处理确保您的应用程序不会因意外错误而崩溃,并允许您优雅处理不同的故障方案。 请记住向用户提供信息性错误消息或日志详细信息以进行调试。import javax.ws.rs.client.Client; import javax.ws.rs.client.ClientBuilder; import javax.ws.rs.client.Entity; import javax.ws.rs.client.WebTarget; import javax.ws.rs.core.MediaType; import javax.ws.rs.core.Response; import com.fasterxml.jackson.databind.ObjectMapper; //Jackson library public class ReadPostResponseBody { public static void main(String[] args) { Client client = ClientBuilder.newClient(); WebTarget target = client.target("http://your-api-endpoint.com/your-resource"); // Create a sample request entity (replace with your actual data) String requestBody = "{\"key1\":\"value1\", \"key2\":\"value2\"}"; Response response = target.request(MediaType.APPLICATION_JSON).post(Entity.json(requestBody)); if (response.getStatus() == 200) { //Check for successful response try { ObjectMapper objectMapper = new ObjectMapper(); YourResponseObject responseObject = objectMapper.readValue(response.readEntity(String.class), YourResponseObject.class); //Process responseObject System.out.println("Response: " + responseObject); } catch (Exception e) { e.printStackTrace(); } } else { System.err.println("Request failed with status code: " + response.getStatus()); } response.close(); client.close(); } //Define your response object structure public static class YourResponseObject { // Your class fields here } }
登录后复制登录后复制>
以上是从邮政请求中读取jax-rs客户端中的响应主体的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
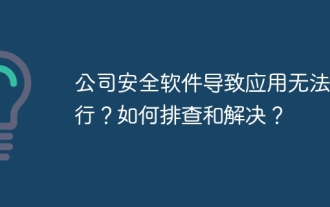
公司安全软件导致部分应用无法正常运行的排查与解决方法许多公司为了保障内部网络安全,会部署安全软件。...
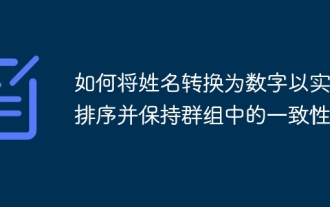
将姓名转换为数字以实现排序的解决方案在许多应用场景中,用户可能需要在群组中进行排序,尤其是在一个用...
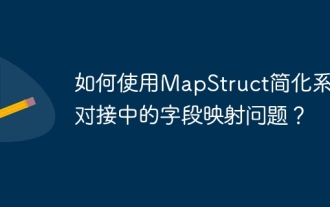
系统对接中的字段映射处理在进行系统对接时,常常会遇到一个棘手的问题:如何将A系统的接口字段有效地映�...
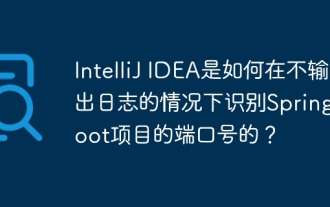
在使用IntelliJIDEAUltimate版本启动Spring...
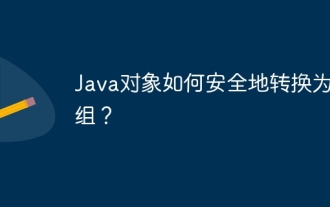
Java对象与数组的转换:深入探讨强制类型转换的风险与正确方法很多Java初学者会遇到将一个对象转换成数组的�...
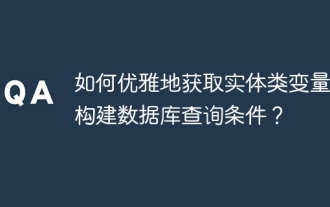
在使用MyBatis-Plus或其他ORM框架进行数据库操作时,经常需要根据实体类的属性名构造查询条件。如果每次都手动...
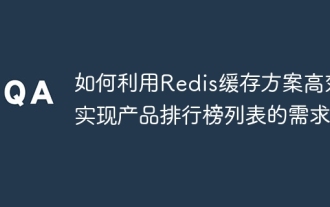
Redis缓存方案如何实现产品排行榜列表的需求?在开发过程中,我们常常需要处理排行榜的需求,例如展示一个�...
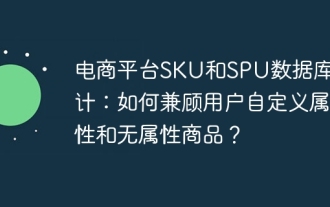
电商平台SKU和SPU表设计详解本文将探讨电商平台中SKU和SPU的数据库设计问题,特别是如何处理用户自定义销售属...
