堆栈数据结构|后进先出 (LIFO)
- - 推送(添加元素):将元素添加到堆栈顶部。
- - pop(删除元素):从顶部删除元素。
- - isfull:检查堆栈是否已达到其限制(在本例中为 10)。
- - isempty:检查堆栈是否为空。
- - 显示:显示堆栈元素。
1.示例:
索引.html
<meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>stack | last in first out (lifo) or first in last out | - by sudhanshu gaikwad (filo)</title> <h3 id="stack-in-javascript">stack in javascript</h3> <script> let data = []; // add an element to the array function addele(ele) { if (isfull()) { console.log("array is full, element can't be added!"); } else { console.log("element added!"); data.push(ele); } } // check if the array is full function isfull() { return data.length >= 10; } // remove an element from the array function remove() { if (isempty()) { console.log("array is empty, can't remove element!"); } else { data.pop(); console.log("element removed!"); } } // check if the array is empty function isempty() { return data.length === 0; } // display the array elements function display() { console.log("updated array >> ", data); } // example usage addele(55); addele(85); addele(25); remove(); display(); // [55, 85] </script>
2.示例:
index2.html
<meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>what is stack in javascript | by sudhanshu gaikwad</title> <style> * { box-sizing: border-box; } body { font-family: "roboto condensed", sans-serif; background-color: #f4f4f4; margin: 0; padding: 0; display: flex; flex-direction: column; justify-content: center; align-items: center; height: 100vh; } .container { background-color: white; padding: 20px; border-radius: 10px; box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1); max-width: 400px; width: 100%; margin-bottom: 20px; } h3 { color: #333; text-align: center; margin-bottom: 20px; } input { padding: 10px; width: calc(100% - 20px); margin-bottom: 10px; border: 1px solid #ccc; border-radius: 5px; } button { padding: 10px; margin: 10px 0; border: none; border-radius: 5px; background-color: #292f31; color: white; cursor: pointer; width: 100%; } button:hover { background-color: #e9e9ea; color: #292f31; } .message { margin-top: 15px; color: #333; font-size: 16px; text-align: center; } .footer { text-align: center; margin-top: 20px; font-size: 14px; color: #555; } /* responsive design */ @media (max-width: 768px) { .container { padding: 15px; max-width: 90%; } button { font-size: 14px; } input { font-size: 14px; } } </style> <div class="container"> <!-- title --> <h3 id="stack-in-javascript">stack in javascript</h3> <!-- input section --> <input type="text" id="addele" placeholder="enter an element"> <!-- buttons section --> <button onclick="adddata()">add element</button> <button onclick="removeele()">remove element</button> <button onclick="display()">show array</button> <!-- message sections --> <div id="add" class="message"></div> <div id="remove" class="message"></div> <div id="display" class="message"></div> </div> <!-- footer with copyright symbol --> <div class="footer"> © 2024 sudhanshu developer | all rights reserved </div> <script> let data = []; // function to add an element to the stack function adddata() { let newele = document.getelementbyid("addele").value; if (isfull()) { document.getelementbyid("add").innerhtml = "array is full, element cannot be added!"; } else if (newele.trim() === "") { document.getelementbyid("add").innerhtml = "please enter a valid element!"; } else { data.push(newele); document.getelementbyid("add").innerhtml = `element "${newele}" added!`; document.getelementbyid("addele").value = ""; console.log("current array: ", data); display(); } } function isfull() { return data.length >= 10; } function removeele() { if (isempty()) { document.getelementbyid("remove").innerhtml = "array is empty!"; } else { let removedelement = data.pop(); document.getelementbyid("remove").innerhtml = `element "${removedelement}" removed!`; console.log("current array: ", data); display(); } } function isempty() { return data.length === 0; } function display() { let displayarea = document.getelementbyid("display"); displayarea.innerhtml = ""; if (data.length === 0) { displayarea.innerhtml = "no elements in the array!"; console.log("array is empty."); } else { for (let i = 0; i < data.length; i ) { displayarea.innerhtml = `element ${i 1}: ${data[i]}<br>`; } console.log("displaying array: ", data); } } </script>
输出:
带有用户输入的 c 语言堆栈
#include <stdio.h> #include <stdbool.h> #define max 10 int data[max]; int top = -1; // function to check if the stack is full bool isfull() { return top >= max - 1; } // function to check if the stack is empty bool isempty() { return top == -1; } // function to add an element to the stack (push operation) void addele() { int ele; if (isfull()) { printf("array is full, element can't be added!\n"); } else { printf("enter an element to add: "); scanf("%d", &ele); // read user input data[ top] = ele; // increment top and add element printf("element %d added!\n", ele); } } // function to remove an element from the stack (pop operation) void remove() { if (isempty()) { printf("array is empty, can't remove element!\n"); } else { printf("element %d removed!\n", data[top--]); // remove element and decrement top } } // function to display all elements in the stack void display() { if (isempty()) { printf("array is empty!\n"); } else { printf("updated array >> "); for (int i = 0; i <p><strong>示例输出:</strong><br> </p> <pre class="brush:php;toolbar:false">1. Add Element 2. Remove Element 3. Display Stack 4. Exit Enter your choice: 1 Enter an element to add: 55 Element 55 Added! 1. Add Element 2. Remove Element 3. Display Stack 4. Exit Enter your choice: 3 Updated Array >> 55 1. Add Element 2. Remove Element 3. Display Stack 4. Exit Enter your choice: 4 Exiting...
以上是堆栈数据结构|后进先出 (LIFO)的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
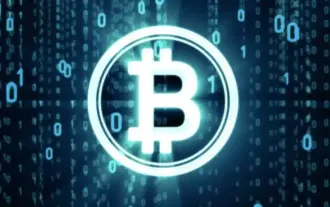
Binance、OKX、gate.io等十大数字货币交易所完善系统、高效多元化交易和严密安全措施严重推崇。
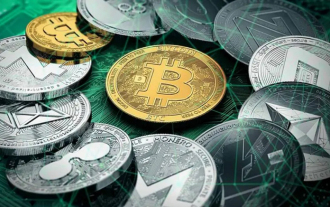
全球十大加密货币交易平台包括Binance、OKX、Gate.io、Coinbase、Kraken、Huobi Global、Bitfinex、Bittrex、KuCoin和Poloniex,均提供多种交易方式和强大的安全措施。
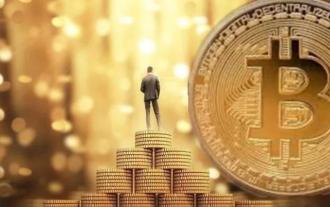
靠谱的数字货币交易平台推荐:1. OKX,2. Binance,3. Coinbase,4. Kraken,5. Huobi,6. KuCoin,7. Bitfinex,8. Gemini,9. Bitstamp,10. Poloniex,这些平台均以其安全性、用户体验和多样化的功能着称,适合不同层次的用户进行数字货币交易
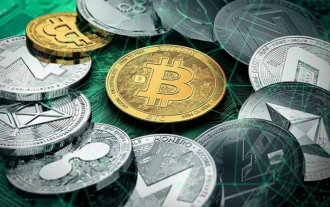
2025年全球十大加密货币交易所包括Binance、OKX、Gate.io、Coinbase、Kraken、Huobi、Bitfinex、KuCoin、Bittrex和Poloniex,均以高交易量和安全性着称。
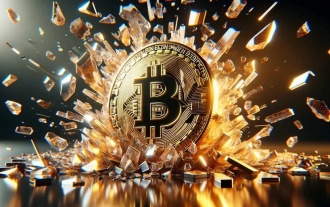
比特币的价格在20,000到30,000美元之间。1. 比特币自2009年以来价格波动剧烈,2017年达到近20,000美元,2021年达到近60,000美元。2. 价格受市场需求、供应量、宏观经济环境等因素影响。3. 通过交易所、移动应用和网站可获取实时价格。4. 比特币价格波动性大,受市场情绪和外部因素驱动。5. 与传统金融市场有一定关系,受全球股市、美元强弱等影响。6. 长期趋势看涨,但需谨慎评估风险。
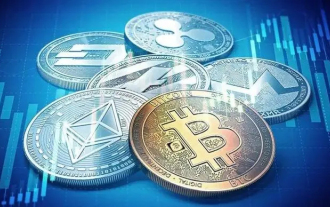
目前排名前十的虚拟币交易所:1.币安,2. OKX,3. Gate.io,4。币库,5。海妖,6。火币全球站,7.拜比特,8.库币,9.比特币,10。比特戳。

MeMebox 2.0通过创新架构和性能突破重新定义了加密资产管理。1) 它解决了资产孤岛、收益衰减和安全与便利悖论三大痛点。2) 通过智能资产枢纽、动态风险管理和收益增强引擎,提升了跨链转账速度、平均收益率和安全事件响应速度。3) 为用户提供资产可视化、策略自动化和治理一体化,实现了用户价值重构。4) 通过生态协同和合规化创新,增强了平台的整体效能。5) 未来将推出智能合约保险池、预测市场集成和AI驱动资产配置,继续引领行业发展。
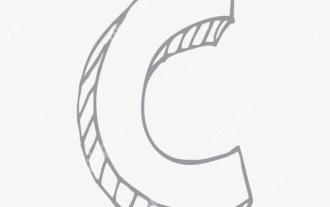
在C 中测量线程性能可以使用标准库中的计时工具、性能分析工具和自定义计时器。1.使用库测量执行时间。2.使用gprof进行性能分析,步骤包括编译时添加-pg选项、运行程序生成gmon.out文件、生成性能报告。3.使用Valgrind的Callgrind模块进行更详细的分析,步骤包括运行程序生成callgrind.out文件、使用kcachegrind查看结果。4.自定义计时器可灵活测量特定代码段的执行时间。这些方法帮助全面了解线程性能,并优化代码。
