PHP 兑现队列类 仅供参考
PHP 实现队列类 仅供参考
<?php /** * PHP Class for queue * @author yangqijun@live.cn * @copyright DataFrog Beijingbei Ltd. 2011-07-25 */ class Queue { public $length=12; public $queue = array(); // if String like this "22,23,24" convert to array to do queue public $delimiter=','; function __construct($queue=array()) { $this->queue=$queue; } /** * @desc start queue * @param String $param new queue element */ public function run($param) { if(!is_array($this->queue)){ $this->strToQue(); } $currentlength=$this->countqueue(); //Count the queue length if($currentlength<$this->length&&$this->length>0) { $this->queAdd($param); }else if($this->length=0) { $this->queue[]=$param; } else { $this->queRemove(); $this->queAdd($param); } return $this->queue; } /** * String like this "22,23,24" convert to array to do queue * @param String $string * @param String $delimiter */ public function strToQue (){ if (empty($this->queue)) { $this->queue=array(); } else { $this->queue=explode($this->delimiter,$this->queue); } } /** * insert $node into queue * @param string $node */ private function queAdd($node){ array_push($this->queue,$node); $this->countqueue(); } private function queRemove(){ $node = array_shift($this->queue); $this->countqueue(); return $node; } private function countqueue(){ $currentlength= count($this->queue); return $currentlength; } function __destruct() { unset($this->queue); } } //example $str='' ; //array(); $obj=new Queue ($str); $obj->length=8; // 队列元素长度 $obj->delimiter='|'; //如果队列是字符串,则元素直接的分隔符为| $a=$obj->run('91'); //要添加到队列中的元素 $a=$obj->run('92'); $a=$obj->run('93'); $a=$obj->run('94'); print_r($a); ?>

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
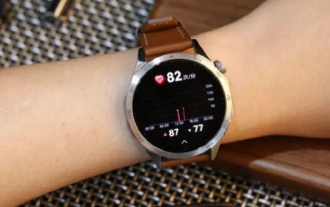
许多用户在选择智能手表的时候都会选择的华为的品牌,其中华为GT3pro和GT4都是非常热门的选择,不少用户都很好奇华为GT3pro和GT4有什么区别,下面就就给大家介绍一下二者。华为GT3pro和GT4有什么区别一、外观GT4:46mm和41mm,材质是玻璃表镜+不锈钢机身+高分纤维后壳。GT3pro:46.6mm和42.9mm,材质是蓝宝石玻璃表镜+钛金属机身/陶瓷机身+陶瓷后壳二、健康GT4:采用最新的华为Truseen5.5+算法,结果会更加的精准。GT3pro:多了ECG心电图和血管及安
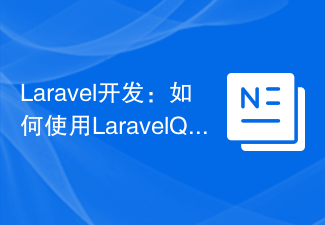
随着应用程序变得越来越复杂,处理和管理大量数据和流程是一个挑战。为了处理这种情况,Laravel为用户提供了一个非常强大的工具,即Laravel队列(Queue)。它允许开发人员在后台运行诸如发送电子邮件,生成PDF,处理图像剪裁等任务,而不会对用户界面产生任何影响。在这篇文章中,我们将深入研究如何使用Laravel队列。什么是LaravelQueue队列

function是函数的意思,是一段具有特定功能的可重复使用的代码块,是程序的基本组成单元之一,可以接受输入参数,执行特定的操作,并返回结果,其目的是封装一段可重复使用的代码,提高代码的可重用性和可维护性。
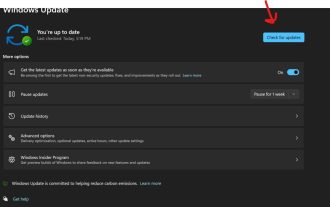
为什么截图工具在Windows11上不起作用了解问题的根本原因有助于找到正确的解决方案。以下是截图工具可能无法正常工作的主要原因:对焦助手已打开:这可以防止截图工具打开。应用程序损坏:如果截图工具在启动时崩溃,则可能已损坏。过时的图形驱动程序:不兼容的驱动程序可能会干扰截图工具。来自其他应用程序的干扰:其他正在运行的应用程序可能与截图工具冲突。证书已过期:升级过程中的错误可能会导致此issu简单的解决方案这些适合大多数用户,不需要任何特殊的技术知识。1.更新窗口和Microsoft应用商店应用程
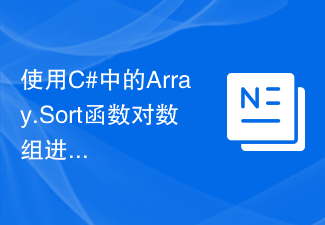
标题:C#中使用Array.Sort函数对数组进行排序的示例正文:在C#中,数组是一种常用的数据结构,经常需要对数组进行排序操作。C#提供了Array类,其中有Sort方法可以方便地对数组进行排序。本文将演示如何使用C#中的Array.Sort函数对数组进行排序,并提供具体的代码示例。首先,我们需要了解一下Array.Sort函数的基本用法。Array.So
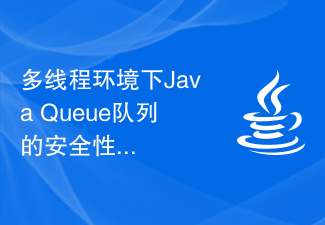
JavaQueue队列在多线程环境下的安全性问题与解决方案引言:在多线程编程中,程序中的共享资源可能面临竞争条件,这可能导致数据的不一致性或者错误。在Java中,Queue队列是一种常用的数据结构,在多个线程同时操作队列的情况下,就存在安全性问题。本文将讨论JavaQueue队列在多线程环境下的安全性问题,并介绍几种解决方案,重点以代码示例的方式解释。一
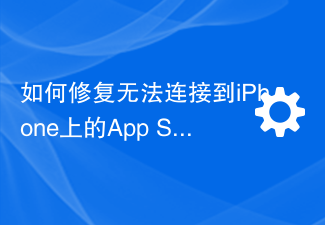
第1部分:初始故障排除步骤检查苹果的系统状态:在深入研究复杂的解决方案之前,让我们从基础知识开始。问题可能不在于您的设备;苹果的服务器可能会关闭。访问Apple的系统状态页面,查看AppStore是否正常工作。如果有问题,您所能做的就是等待Apple修复它。检查您的互联网连接:确保您拥有稳定的互联网连接,因为“无法连接到AppStore”问题有时可归因于连接不良。尝试在Wi-Fi和移动数据之间切换或重置网络设置(“常规”>“重置”>“重置网络设置”>设置)。更新您的iOS版本:
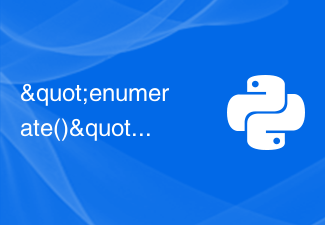
在本文中,我们将了解enumerate()函数以及Python中“enumerate()”函数的用途。什么是enumerate()函数?Python的enumerate()函数接受数据集合作为参数并返回一个枚举对象。枚举对象以键值对的形式返回。key是每个item对应的索引,value是items。语法enumerate(iterable,start)参数iterable-传入的数据集合可以作为枚举对象返回,称为iterablestart-顾名思义,枚举对象的起始索引由start定义。如果我们忽
