PHP状态模式
####状态设计模式* 状态模式的作用是允许对象在状态改变时改变其行为* 对象中频繁的状态非常依赖于条件语句,就自身来说条件语句并没有什么问题,不过,如果选项太多,以至于程序出现混乱,或者增加或改变选项需要太多的是时间。```<?php/** * Created by PhpStorm. * User: gewenrui * Date: 16/3/12 * Time: 下午3:57 */header("Content-type: text/html; charset=utf-8");class Context{ private $offState; private $onState; private $brighterState; private $brightestState; private $currentState; public function __construct() { $this->offState = new OffState($this); $this->onState = new Onstate($this); $this->brighterState = new BrighterState($this); $this->brightestState = new BrightestState($this); $this->currentState = $this->offState; } public function turnOnLight(){ $this->currentState->turnLightOn(); } public function turnOffLight(){ $this->currentState->turnLightOff(); } public function turnBrighter(){ $this->currentState->turnBrighter(); } public function turnBrightest(){ $this->currentState->turnBrightest(); } public function setState(IState $state){ $this->currentState = $state; } public function getOnState(){ return $this->onState; } public function getOffState(){ return $this->offState; } public function getBrighterState(){ return $this->brighterState; } public function getBrightestState(){ return $this->brightestState; }}interface IState{ public function turnLightOn(); public function turnLightOff(); public function turnBrighter(); public function turnBrightest();}class Onstate implements IState{ private $context; public function __construct(Context $contextNew) { $this->context = $contextNew; } public function turnLightOff() { echo "Lights off"."</br>"; $this->context->setState($this->context->getOffState()); } public function turnLightOn() { echo "Light is already on there is no action"."</br>"; } public function turnBrighter() { echo "turn 明亮"."</br>"; } public function turnBrightest() { echo "turn 最亮"."</br>"; }}class OffState implements IState{ private $context; public function __construct(Context $contextNew) { $this->context = $contextNew; } public function turnLightOn() { echo "Lights On Now i can see"."</br>"; $this->context->setState($this->context->getOnState()); } public function turnLightOff() { echo "Light is already off"."</br>"; } public function turnBrightest() { echo "turn 最亮"."</br>"; } public function turnBrighter() { echo "turn 较亮"."</br>"; }}class BrighterState implements IState{ private $context; public function __construct(Context $contextNow) { $this->context = $contextNow; } public function turnBrighter() { echo "变暗了"; } public function turnBrightest() { $this->context->setState($this->context->getBrighterState()); } public function turnLightOff() { echo "关灯了"; } public function turnLightOn() { echo "灯是亮的"; }}class BrightestState implements IState{ private $context; public function __construct(Context $contextNow) { $this->context = $contextNow; } public function turnLightOff() { $this->context->setState($this->context->getOffState()); } public function turnBrightest() { echo "this is brightest"."</br>"; } public function turnBrighter() { echo "this is a little 暗淡"."</br>"; } public function turnLightOn() { echo "there is no use"."</br>"; }}class Client{ private $context; public function __construct() { $this->context = new Context(); $this->context->turnOnLight(); $this->context->turnOnLight(); $this->context->turnOffLight(); $this->context->turnOffLight(); $this->context->turnOnLight(); $this->context->turnBrighter(); $this->context->turnBrightest(); $this->context->turnOffLight(); }}$data = new Client();```

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)

热门话题
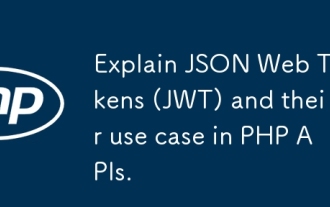
JWT是一种基于JSON的开放标准,用于在各方之间安全地传输信息,主要用于身份验证和信息交换。1.JWT由Header、Payload和Signature三部分组成。2.JWT的工作原理包括生成JWT、验证JWT和解析Payload三个步骤。3.在PHP中使用JWT进行身份验证时,可以生成和验证JWT,并在高级用法中包含用户角色和权限信息。4.常见错误包括签名验证失败、令牌过期和Payload过大,调试技巧包括使用调试工具和日志记录。5.性能优化和最佳实践包括使用合适的签名算法、合理设置有效期、
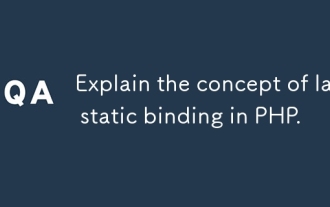
文章讨论了PHP 5.3中引入的PHP中的晚期静态结合(LSB),从而允许静态方法的运行时分辨率调用以获得更灵活的继承。 LSB的实用应用和潜在的触摸
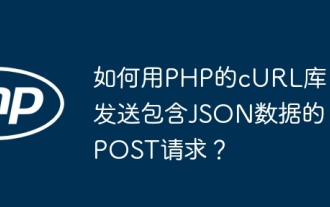
使用PHP的cURL库发送JSON数据在PHP开发中,经常需要与外部API进行交互,其中一种常见的方式是使用cURL库发送POST�...
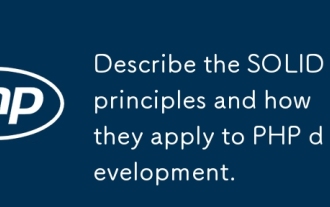
SOLID原则在PHP开发中的应用包括:1.单一职责原则(SRP):每个类只负责一个功能。2.开闭原则(OCP):通过扩展而非修改实现变化。3.里氏替换原则(LSP):子类可替换基类而不影响程序正确性。4.接口隔离原则(ISP):使用细粒度接口避免依赖不使用的方法。5.依赖倒置原则(DIP):高低层次模块都依赖于抽象,通过依赖注入实现。
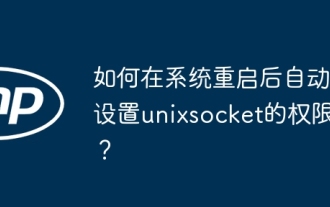
如何在系统重启后自动设置unixsocket的权限每次系统重启后,我们都需要执行以下命令来修改unixsocket的权限:sudo...
