PHP实现适用于自定义的验证码类,php验证码
PHP实现适用于自定义的验证码类,php验证码
本文实例为大家分享了PHP验证码类,利用对象来实现的验证码类,供大家参考,具体内容如下
<?php /* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ Class Image{ private $img; public $width = 85; public $height = 25; public $code; public $code_len = 4; public $code_str = "329832983DSDSKDSLKQWEWQ2lkfDSFSDjfdsfdsjwlkfj93290KFDSKJFDSOIDSLK"; public $bg_color = '#DCDCDC'; public $font_size = 16; public $font = 'font.ttf'; public $font_color = '#000000'; //创建验证码饿字符创 public function create_code(){ $code = ''; for( $i=0;$i<$this->code_len;$i++ ){ $code .= $this->code_str[mt_rand(0, strlen($this->code_str)-1)]; } return $this->code = $code; } //输出图像 public function getImage(){ $w = $this->width; $h = $this->height; $bg_color = $this->bg_color; $img = imagecreatetruecolor($w, $h); $bg_color = imagecolorallocate($img, hexdec(substr($bg_color, 1,2)), hexdec(substr($bg_color, 3,2)), hexdec(substr($bg_color, 5,2))); imagefill($img, 0, 0, $bg_color); $this->img = $img; $this->create_font(); $this->create_pix(); $this->show_code(); } //写入验证码 public function create_font(){ $this->create_code(); $color = $this->font_color; $font_color = imagecolorallocate($this->img, hexdec(substr($color,1,2)), hexdec(substr($color, 3,2)), hexdec(substr($color,5,2))); $x = $this->width/$this->code_len; for( $i=0;$i<$this->code_len;$i++ ){ $txt_color = imagecolorallocate($this->img, mt_rand(0,100), mt_rand(0, 150), mt_rand(0, 200)); imagettftext($this->img, $this->font_size, mt_rand(-30, 30), $x*$i+mt_rand(3, 6), mt_rand($this->height/1.2, $this->height), $txt_color, $this->font , $this->code[$i]); //imagestring($this->img, $this->font_size, $x*$i+mt_rand(3, 6),mt_rand(0, $this->height/4) , $this->code[$i], $font_color); } $this->font_color = $font_color; } //画干扰线 public function create_pix(){ $pix_color= $this->font_color; for($i=0;$i<100;$i++){ imagesetpixel($this->img, mt_rand(0, $this->width),mt_rand(0, $this->height), $pix_color); } for($j=0;$j<4;$j++){ imagesetthickness($this->img, mt_rand(1, 2)); imageline($this->img, mt_rand(0, $this->width), mt_rand(0, $this->height), mt_rand(0, $this->width), mt_rand(0, $this->height), $pix_color); } } //得到验证码 public function getCode(){ return strtoupper($this->code); } //输出验证码 private function show_code(){ header("Content-type:image/png"); imagepng($this->img); imagedestroy($this->img); } }
效果图:
精彩专题分享:ASP.NET验证码大全 PHP验证码大全 java验证码大全
以上就是使用对象编写的验证码类的全部内容,希望对大家学习PHP程序设计有所帮助。

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
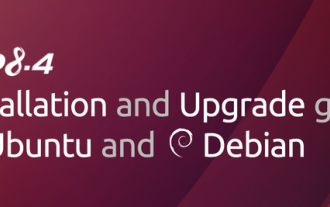
PHP 8.4 带来了多项新功能、安全性改进和性能改进,同时弃用和删除了大量功能。 本指南介绍了如何在 Ubuntu、Debian 或其衍生版本上安装 PHP 8.4 或升级到 PHP 8.4
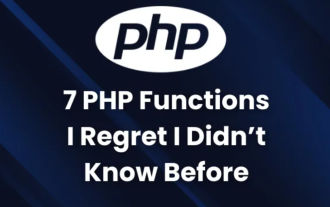
如果您是一位经验丰富的 PHP 开发人员,您可能会感觉您已经在那里并且已经完成了。您已经开发了大量的应用程序,调试了数百万行代码,并调整了一堆脚本来实现操作
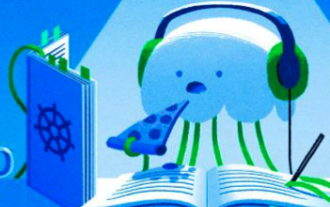
Visual Studio Code,也称为 VS Code,是一个免费的源代码编辑器 - 或集成开发环境 (IDE) - 可用于所有主要操作系统。 VS Code 拥有针对多种编程语言的大量扩展,可以轻松编写
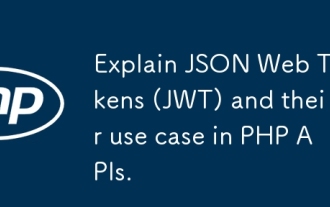
JWT是一种基于JSON的开放标准,用于在各方之间安全地传输信息,主要用于身份验证和信息交换。1.JWT由Header、Payload和Signature三部分组成。2.JWT的工作原理包括生成JWT、验证JWT和解析Payload三个步骤。3.在PHP中使用JWT进行身份验证时,可以生成和验证JWT,并在高级用法中包含用户角色和权限信息。4.常见错误包括签名验证失败、令牌过期和Payload过大,调试技巧包括使用调试工具和日志记录。5.性能优化和最佳实践包括使用合适的签名算法、合理设置有效期、
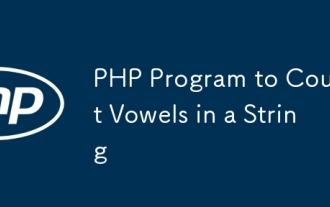
字符串是由字符组成的序列,包括字母、数字和符号。本教程将学习如何使用不同的方法在PHP中计算给定字符串中元音的数量。英语中的元音是a、e、i、o、u,它们可以是大写或小写。 什么是元音? 元音是代表特定语音的字母字符。英语中共有五个元音,包括大写和小写: a, e, i, o, u 示例 1 输入:字符串 = "Tutorialspoint" 输出:6 解释 字符串 "Tutorialspoint" 中的元音是 u、o、i、a、o、i。总共有 6 个元
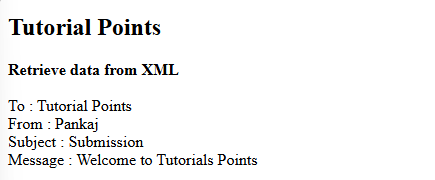
本教程演示了如何使用PHP有效地处理XML文档。 XML(可扩展的标记语言)是一种用于人类可读性和机器解析的多功能文本标记语言。它通常用于数据存储
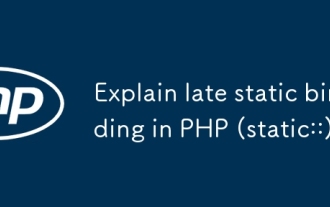
静态绑定(static::)在PHP中实现晚期静态绑定(LSB),允许在静态上下文中引用调用类而非定义类。1)解析过程在运行时进行,2)在继承关系中向上查找调用类,3)可能带来性能开销。

PHP的魔法方法有哪些?PHP的魔法方法包括:1.\_\_construct,用于初始化对象;2.\_\_destruct,用于清理资源;3.\_\_call,处理不存在的方法调用;4.\_\_get,实现动态属性访问;5.\_\_set,实现动态属性设置。这些方法在特定情况下自动调用,提升代码的灵活性和效率。
