Python开发【Django】:组合搜索、JSONP、XSS过滤
1、简单实现
关联文件:
from django.conf.urls import url from . import views urlpatterns = [ url(r'^index.html/$',views.index), url(r'^article/(?P<article_type>\d+)-(?P<category>\d+).html/$',views.article) ] url.py
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Title</title> <style> .condition a{ display:inline-block; padding: 3px 5px; border: 1px solid black; } .condition a.active{ background-color: brown; } </style> </head> <body> <h2>过滤条件</h2> <div> {% if kwargs.article_type == 0 %} <a href="/article/0-{{ kwargs.category }}.html">全部</a> {% else %} <a href="/article/0-{{ kwargs.category }}.html">全部</a> {% endif %} {% for row in article_type %} {% if row.id == kwargs.article_type %} <a href="/article/{{ row.id }}-{{ kwargs.category }}.html">{{ row.caption }}</a> {% else %} <a href="/article/{{ row.id }}-{{ kwargs.category }}.html">{{ row.caption }}</a> {% endif %} {% endfor %} </div> <div> {% if kwargs.category == 0 %} <a href="/article/{{ kwargs.article_type }}-0.html">全部</a> {% else %} <a href="/article/{{ kwargs.article_type }}-0.html">全部</a> {% endif %} {% for row in category %} {% if row.id == kwargs.category %} <a href="/article/{{ kwargs.article_type }}-{{ row.id }}.html">{{ row.caption }}</a> {% else %} <a href="/article/{{ kwargs.article_type }}-{{ row.id }}.html">{{ row.caption }}</a> {% endif %} {% endfor %} </div> <h2>查询结果</h2> <ul> {% for row in articles %} <li>{{ row.id }}-{{ row.title }}------[{{ row.article_type.caption }}]-[{{ row.category.caption }}]</li> {% endfor %} </ul> </body> </html> article.html
数据库结构:
from django.db import models # Create your models here. class Categoery(models.Model): caption = models.CharField(max_length=16) class ArticleType(models.Model): caption = models.CharField(max_length=16) class Article(models.Model): title = models.CharField(max_length=32) content = models.CharField(max_length=255) category = models.ForeignKey(Categoery) article_type = models.ForeignKey(ArticleType)
处理文件:
from . import models def article(request,*args,**kwargs): search_dict = {} for key,value in kwargs.items(): kwargs[key] = int(value) # 把字符类型转化为int类型 方便前端做if a == b 这样的比较 if value !='0': search_dict[key] = value articles = models.Article.objects.filter(**search_dict) # 字典为空时表示搜索所有 article_type = models.ArticleType.objects.all() category = models.Categoery.objects.all() return render(request,'article.html',{'articles':articles, 'article_type':article_type, 'category':category , 'kwargs':kwargs})
注:实现此功能并不难,最重要的是理清里面的思路;首先先要确定url访问路径格式http://127.0.0.1:8000/article/0-0.html ,第一个0表示article_type字段,第二个0表示category字段,如果为零时,表示搜索此字段全部信息,确认好这个,是成功的第一步,处理文件上有检索的处理;第二个关键点是生成字典search_dict进行相关的搜索,如果是0表示搜索全部;第三个关键点,也是很巧妙的一个方式,把参数kwargs再次传到前端,简直神来之笔!
2、另一种尝试(加载内存调优)
由于ArticleType类型是博客定死的数据,后期不会做变动,可以把数据加载到内存当中,加快查询速度
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Title</title> <style> .condition a{ display:inline-block; padding: 3px 5px; border: 1px solid black; } .condition a.active{ background-color: brown; } </style> </head> <body> <h2>过滤条件</h2> <div> {% if kwargs.article_type_id == 0 %} <a href="/article/0-{{ kwargs.category_id }}.html">全部</a> {% else %} <a href="/article/0-{{ kwargs.category_id }}.html">全部</a> {% endif %} {% for row in article_type%} {% if row.0 == kwargs.article_type_id %} <a href="/article/{{ row.0 }}-{{ kwargs.category_id }}.html">{{ row.1 }}</a> {% else %} <a href="/article/{{ row.0 }}-{{ kwargs.category_id }}.html">{{ row.1 }}</a> {% endif %} {% endfor %} </div> <div> {% if kwargs.category_id == 0 %} <a href="/article/{{ kwargs.article_type_id }}-0.html">全部</a> {% else %} <a href="/article/{{ kwargs.article_type_id }}-0.html">全部</a> {% endif %} {% for row in category %} {% if row.id == kwargs.category_id %} <a href="/article/{{ kwargs.article_type_id }}-{{ row.id }}.html">{{ row.caption }}</a> {% else %} <a href="/article/{{ kwargs.article_type_id }}-{{ row.id }}.html">{{ row.caption }}</a> {% endif %} {% endfor %} </div> <h2>查询结果</h2> <ul> {% for row in articles %} <li>{{ row.id }}-{{ row.title }}------[{{ row.article_type }}]-[{{ row.category.caption }}]</li> {% endfor %} </ul> </body> </html> article.html
from django.shortcuts import render from django.shortcuts import HttpResponse # Create your views here. def index(request): return HttpResponse('Ok') from . import models def article(request,*args,**kwargs): search_dict = {} for key,value in kwargs.items(): kwargs[key] = int(value) # 把字符类型转化为int类型 方便前端做if a == b 这样的比较 if value !='0': search_dict[key] = value print(kwargs) articles = models.Article.objects.filter(**search_dict) # 字典为空时表示搜索所有 article_type = models.Article.type_choice print(article_type) category = models.Categoery.objects.all() return render(request,'article.html',{'articles':articles, 'article_type':article_type, 'category':category , 'kwargs':kwargs}) 处理文件.py
数据库文件:
from django.db import models # Create your models here. class Categoery(models.Model): caption = models.CharField(max_length=16) # class ArticleType(models.Model): # caption = models.CharField(max_length=16) class Article(models.Model): title = models.CharField(max_length=32) content = models.CharField(max_length=255) category = models.ForeignKey(Categoery) # article_type = models.ForeignKey(ArticleType) type_choice = [ (1,'Python'), (2,'Linux'), (3,'大数据'), (4,'架构'), ] article_type_id = models.IntegerField(choices=type_choice)
3、利用simple_tag把代码优化
关联文件:
from django.db import models # Create your models here. class Categoery(models.Model): caption = models.CharField(max_length=16) class ArticleType(models.Model): caption = models.CharField(max_length=16) class Article(models.Model): title = models.CharField(max_length=32) content = models.CharField(max_length=255) category = models.ForeignKey(Categoery) article_type = models.ForeignKey(ArticleType) # type_choice = [ # (1,'Python'), # (2,'Linux'), # (3,'大数据'), # (4,'架构'), # ] # article_type_id = models.IntegerField(choices=type_choice) 数据库文件.py
from django.shortcuts import render from django.shortcuts import HttpResponse # Create your views here. def index(request): return HttpResponse('Ok') from . import models def article(request, *args, **kwargs): search_dict = {} for key, value in kwargs.items(): kwargs[key] = int(value) # 把字符类型转化为int类型 方便前端做if a == b 这样的比较 if value != '0': search_dict[key] = value articles = models.Article.objects.filter(**search_dict) # 字典为空时表示搜索所有 article_type = models.ArticleType.objects.all() print(article_type) category = models.Categoery.objects.all() return render(request, 'article.html', {'articles': articles, 'article_type': article_type, 'category': category, 'kwargs': kwargs}) 处理文件.py
{% load filter %} <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Title</title> <style> .condition a{ display:inline-block; padding: 3px 5px; border: 1px solid black; } .condition a.active{ background-color: brown; } </style> </head> <body> <h2>过滤条件</h2> <div> {% filter_all kwargs 'article_type'%} {% filter_single article_type kwargs 'article_type'%} </div> <div> {% filter_all kwargs 'category'%} {% filter_single category kwargs 'category'%} </div> <h2>查询结果</h2> <ul> {% for row in articles %} <li>{{ row.id }}-{{ row.title }}------[{{ row.article_type.caption }}]-[{{ row.category.caption }}]</li> {% endfor %} </ul> </body> </html> article.html
创建templatetags目录,在目录下创建filter.py文件:
from django import template from django.utils.safestring import mark_safe register = template.Library() @register.simple_tag def filter_all(kwargs,type_str): print(type_str) if type_str == 'article_type': if kwargs['article_type'] == 0: tmp = '<a href = "/article/0-%s.html" class ="active" > 全部 </a>'%(kwargs['category']) else: tmp = '<a href = "/article/0-%s.html"> 全部 </a>'%(kwargs['category']) elif type_str == 'category': if kwargs['category'] == 0: tmp = '<a href = "/article/%s-0.html" class ="active" > 全部 </a>' % (kwargs['article_type']) else: tmp = '<a href = "/article/%s-0.html"> 全部 </a>' % (kwargs['article_type']) return mark_safe(tmp) @register.simple_tag() def filter_single(type_obj,kwargs,type_str): print(type_str) tmp = '' if type_str == 'article_type': for row in type_obj: if row.id == kwargs['article_type']: tag = '<a class="active" href="/article/%s-%s.html">%s</a>\n'%(row.id,kwargs['category'],row.caption) else: tag = '<a href="/article/%s-%s.html">%s</a>\n' % (row.id, kwargs['category'],row.caption) tmp +=tag elif type_str == 'category': for row in type_obj: if row.id == kwargs['category']: tag = '<a class="active" href="/article/%s-%s.html">%s</a>\n' % (kwargs['article_type'],row.id, row.caption) else: tag = '<a href="/article/%s-%s.html">%s</a>\n' % (kwargs['article_type'], row.id, row.caption) tmp += tag return mark_safe(tmp)
HTML文件主体内容:
{% load filter %} <body> <h2>过滤条件</h2> <div class="condition"> {% filter_all kwargs 'article_type'%} {% filter_single article_type kwargs 'article_type'%} </div> <div class="condition"> {% filter_all kwargs 'category'%} {% filter_single category kwargs 'category'%} </div> <h2>查询结果</h2> <ul> {% for row in articles %} <li>{{ row.id }}-{{ row.title }}------[{{ row.article_type.caption }}]-[{{ row.category.caption }}]</li> {% endfor %} </ul> </body>
JSONP
JSONP(JSON with Padding)是JSON的一种“使用模式”,可用于解决主流浏览器的跨域数据访问的问题。由于同源策略,一般来说位于 server1.example.com 的网页无法与不是 server1.example.com的服务器沟通,而 HTML 的

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)

热门话题
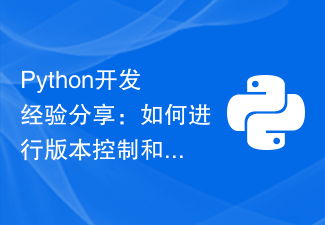
Python开发经验分享:如何进行版本控制和发布管理引言:在Python开发过程中,版本控制和发布管理是非常重要的环节。通过版本控制,我们可以轻松地追踪代码的更改、协同开发、解决冲突等;而发布管理则能够帮助我们组织代码的部署、测试和发布过程,确保代码的质量和稳定性。本文将从版本控制和发布管理两个方面,分享一些Python开发中的经验和实践。一、版本控制版本控

Python作为一种高级编程语言,具有易学易用和开发效率高等优点,在开发人员中越来越受欢迎。但是,由于其垃圾回收机制的实现方式,Python在处理大量内存时,容易出现内存泄漏问题。本文将从常见内存泄漏问题、引起问题的原因以及避免内存泄漏的方法三个方面来介绍Python开发过程中需要注意的事项。一、常见内存泄漏问题内存泄漏是指程序在运行中分配的内存空间无法释放
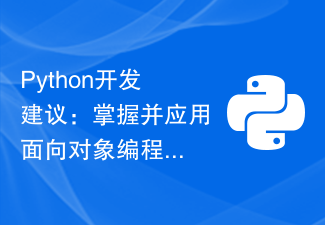
Python是一门强大而灵活的编程语言,广泛应用于各种领域的软件开发。在Python开发过程中,掌握并应用面向对象编程(Object-OrientedProgramming,OOP)的原则是非常重要的。本文将介绍一些关键的Python开发建议,帮助开发者更好地掌握和应用面向对象编程的原则。首先,面向对象编程的核心思想是将问题划分为一系列的对象,并通过对象之
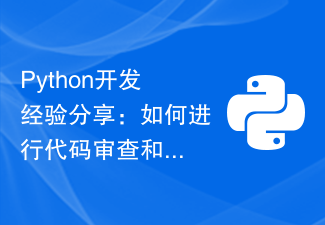
Python开发经验分享:如何进行代码审查和质量保证导言:在软件开发过程中,代码审查和质量保证是至关重要的环节。良好的代码审查可以提高代码质量、减少错误和缺陷,提高程序的可维护性和可扩展性。本文将从以下几个方面分享Python开发中如何进行代码审查和质量保证的经验。一、制定代码审查规范代码审查是一种系统性的活动,需要对代码进行全面的检查和评估。为了规范代码审
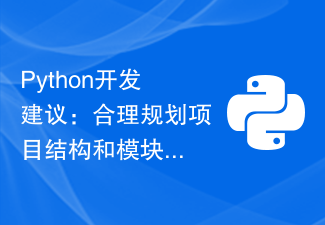
Python开发是一种简单而又强大的编程语言,常被用于开发各种类型的应用程序。然而,对于初学者来说,可能会在项目结构和模块划分方面遇到一些挑战。一个良好的项目结构和模块划分不仅有助于提高代码的可维护性和可扩展性,还能提升团队开发的效率。在本文中,我们将分享一些建议,帮助您合理规划Python项目的结构和模块划分。首先,一个好的项目结构应当能够清晰地展示项目的
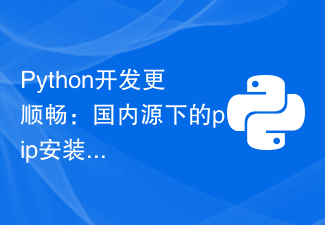
pip国内源安装教程:让你的Python开发更顺畅,需要具体代码示例在Python开发中,使用pip来管理第三方库是非常常见的。然而,由于众所周知的原因,有时候直接使用官方的pip源会遇到下载速度慢、无法连接等问题。为了解决这个问题,国内出现了一些优秀的pip国内源,如阿里云、腾讯云、豆瓣等。使用这些国内源,可以极大地提高下载速度,提升Python开发的效率
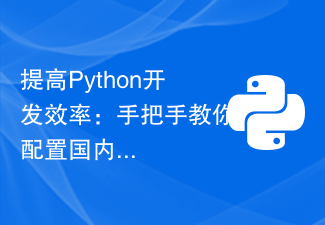
从零开始,一步步教你配置pip国内源,让你的Python开发更高效在Python的开发过程中,我们经常会用到pip来管理第三方库。然而,由于国内的网络环境问题,使用默认的pip源往往会导致下载速度缓慢、甚至无法连接的情况。为了让我们的Python开发更加高效,配置一个国内源是非常有必要的。那么,现在就让我们一步步来配置pip国内源吧!首先,我们需要找到pip
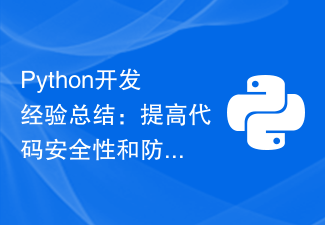
Python开发经验总结:提高代码安全性和防御性的方法随着互联网的发展,代码的安全性和防御性越来越受到关注。特别是Python作为一门广泛使用的动态语言,也面临着各种潜在的风险。本文将总结一些提高Python代码安全性和防御性的方法,希望对Python开发者有所帮助。合理使用输入验证在开发过程中,用户的输入可能包含恶意代码。为了避免这种情况发生,开发者应该对
