Python中functools模块的常用函数解析
1.partial
首先是partial函数,它可以重新绑定函数的可选参数,生成一个callable的partial对象:
>>> int('10') # 实际上等同于int('10', base=10)和int('10', 10) 10 >>> int('10', 2) # 实际上是int('10', base=2)的缩写 2 >>> from functools import partial >>> int2 = partial(int, 2) # 这里我没写base,结果就出错了 >>> int2('10') Traceback (most recent call last): File "<stdin>", line 1, in <module> TypeError: an integer is required >>> int2 = partial(int, base=2) # 把base参数绑定在int2这个函数里 >>> int2('10') # 现在缺省参数base被设为2了 2 >>> int2('10', 3) # 没加base,结果又出错了 Traceback (most recent call last): File "<stdin>", line 1, in <module> TypeError: keyword parameter 'base' was given by position and by name >>> int2('10', base=3) 3 >>> type(int2) <type 'functools.partial'>
从中可以看出,唯一要注意的是可选参数必须写出参数名。
2.update_wrapper
接着是update_wrapper函数,它可以把被封装函数的__name__、__module__、__doc__和 __dict__都复制到封装函数去:
#-*- coding: gbk -*- def thisIsliving(fun): def living(*args, **kw): return fun(*args, **kw) + '活着就是吃嘛。' return living @thisIsliving def whatIsLiving(): "什么是活着" return '对啊,怎样才算活着呢?' print whatIsLiving() print whatIsLiving.__doc__ print from functools import update_wrapper def thisIsliving(fun): def living(*args, **kw): return fun(*args, **kw) + '活着就是吃嘛。' return update_wrapper(living, fun) @thisIsliving def whatIsLiving(): "什么是活着" return '对啊,怎样才算活着呢?' print whatIsLiving() print whatIsLiving.__doc__
结果:
对啊,怎样才算活着呢?活着就是吃嘛。 None 对啊,怎样才算活着呢?活着就是吃嘛。 什么是活着
不过也没多大用处,毕竟只是少写了4行赋值语句而已。
3.wraps
再有是wraps函数,它将update_wrapper也封装了进来:
#-*- coding: gbk -*- from functools import wraps def thisIsliving(fun): @wraps(fun) def living(*args, **kw): return fun(*args, **kw) + '活着就是吃嘛。' return living @thisIsliving def whatIsLiving(): "什么是活着" return '对啊,怎样才算活着呢?' print whatIsLiving() print whatIsLiving.__doc__
结果还是一样的:
对啊,怎样才算活着呢?活着就是吃嘛。 什么是活着
4.total_ordering
最后至于total_ordering函数则给予类丰富的排序方法,使用装饰器简化了操作。如果使用必须在类里面定义一个__lt__(),__le__(), __gt__(), 或__ge__()。应该给类添加一个__eq__() 方法。
from functools import total_ordering @total_ordering class Student(object): def __init__(self, name): self.name = name def __eq__(self, other): return self.name.lower() == other.name.lower() def __lt__(self, other): return self.name.lower() < other.name.lower() a = Student('dan') b = Student('mink') print a > b print a print sorted([b, a])
打印结果
False <__main__.Student object at 0x7f16ecb194d0> [<__main__.Student object at 0x7f16ecb194d0>, <__main__.Student object at 0x7f16ecb195d0>]
更多Python中functools模块的常用函数解析相关文章请关注PHP中文网!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)

热门话题
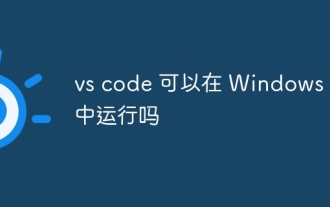
VS Code可以在Windows 8上运行,但体验可能不佳。首先确保系统已更新到最新补丁,然后下载与系统架构匹配的VS Code安装包,按照提示安装。安装后,注意某些扩展程序可能与Windows 8不兼容,需要寻找替代扩展或在虚拟机中使用更新的Windows系统。安装必要的扩展,检查是否正常工作。尽管VS Code在Windows 8上可行,但建议升级到更新的Windows系统以获得更好的开发体验和安全保障。
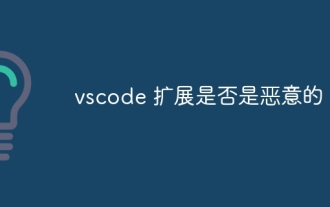
VS Code 扩展存在恶意风险,例如隐藏恶意代码、利用漏洞、伪装成合法扩展。识别恶意扩展的方法包括:检查发布者、阅读评论、检查代码、谨慎安装。安全措施还包括:安全意识、良好习惯、定期更新和杀毒软件。
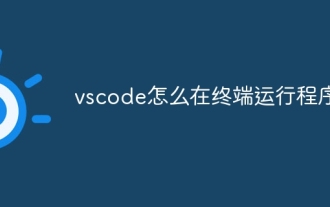
在 VS Code 中,可以通过以下步骤在终端运行程序:准备代码和打开集成终端确保代码目录与终端工作目录一致根据编程语言选择运行命令(如 Python 的 python your_file_name.py)检查是否成功运行并解决错误利用调试器提升调试效率
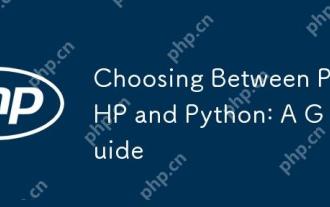
PHP适合网页开发和快速原型开发,Python适用于数据科学和机器学习。1.PHP用于动态网页开发,语法简单,适合快速开发。2.Python语法简洁,适用于多领域,库生态系统强大。
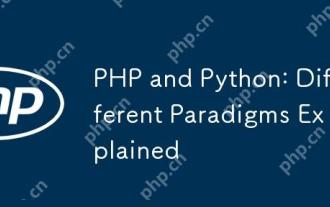
PHP主要是过程式编程,但也支持面向对象编程(OOP);Python支持多种范式,包括OOP、函数式和过程式编程。PHP适合web开发,Python适用于多种应用,如数据分析和机器学习。
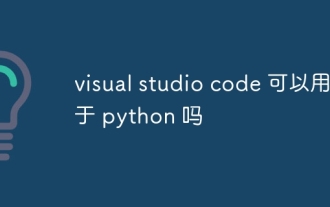
VS Code 可用于编写 Python,并提供许多功能,使其成为开发 Python 应用程序的理想工具。它允许用户:安装 Python 扩展,以获得代码补全、语法高亮和调试等功能。使用调试器逐步跟踪代码,查找和修复错误。集成 Git,进行版本控制。使用代码格式化工具,保持代码一致性。使用 Linting 工具,提前发现潜在问题。
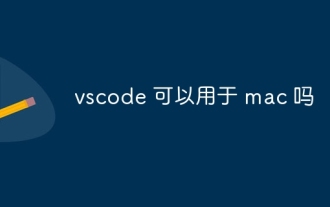
VS Code 可以在 Mac 上使用。它具有强大的扩展功能、Git 集成、终端和调试器,同时还提供了丰富的设置选项。但是,对于特别大型项目或专业性较强的开发,VS Code 可能会有性能或功能限制。
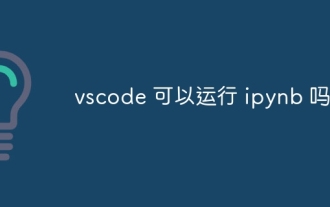
VS Code 运行 Jupyter Notebook 的关键是要确保 Python 环境正确配置,理解代码执行顺序与单元格顺序一致,并注意可能影响性能的大型文件或外部库。VS Code 提供的代码补全和调试功能可以大大提高编码效率和减少错误。
