Json操作日期格式
这次给大家带来Json操作日期格式,Json操作日期格式的注意事项有哪些,下面就是实战案例,一起来看一下。
开发中有时候需要从服务器端返回json格式的数据,在后台代码中如果有DateTime类型的数据使用系统自带的工具类序列化后将得到一个很长的数字表示日期数据,如下所示:
//设置服务器响应的结果为纯文本格式 context.Response.ContentType = "text/plain"; //学生对象集合 List<Student> students = new List<Student> { new Student(){Name ="Tom", Birthday =Convert.ToDateTime("2014-01-31 12:12:12")}, new Student(){Name ="Rose", Birthday =Convert.ToDateTime("2014-01-10 11:12:12")}, new Student(){Name ="Mark", Birthday =Convert.ToDateTime("2014-01-09 10:12:12")} }; //javascript序列化器 JavaScriptSerializer jss=new JavaScriptSerializer(); //序列化学生集合对象得到json字符 string studentsJson=jss.Serialize(students); //将字符串响应到客户端 context.Response.Write(studentsJson); context.Response.End();
运行结果是:
其中Tom所对应生日“2014-01-31”变成了1391141532000,这其实是1970 年 1 月 1 日至今的毫秒数;1391141532000/1000/60/60/24/365=44.11年,44+1970=2014年,按这种方法可以得出年月日时分秒和毫秒。这种格式是一种可行的表示形式但不是普通人可以看懂的友好格式,怎么让这个格式变化?
解决办法:
方法1:在服务器端将日期格式使用Select方法或LINQ表达式转换后发到客户端:
using System; using System.Collections.Generic; using System.Web; using System.Web.Script.Serialization; namespace JsonDate1 { using System.Linq; /// <summary> /// 学生类,测试用 /// </summary> public class Student { /// <summary> /// 姓名 /// </summary> public String Name { get; set; } /// <summary> /// 生日 /// </summary> public DateTime Birthday { get; set; } } /// <summary> /// 返回学生集合的json字符 /// </summary> public class GetJson : IHttpHandler { public void ProcessRequest(HttpContext context) { //设置服务器响应的结果为纯文本格式 context.Response.ContentType = "text/plain"; //学生对象集合 List<Student> students = new List<Student> { new Student(){Name ="Tom",Birthday =Convert.ToDateTime("2014-01-31 12:12:12")}, new Student(){Name ="Rose",Birthday =Convert.ToDateTime("2014-01-10 11:12:12")}, new Student(){Name ="Mark",Birthday =Convert.ToDateTime("2014-01-09 10:12:12")} }; //使用Select方法重新投影对象集合将Birthday属性转换成一个新的属性 //注意属性变化后要重新命名,并立即执行 var studentSet = students.Select ( p => new { p.Name, Birthday = p.Birthday.ToString("yyyy-mm-dd") } ).ToList(); //javascript序列化器 JavaScriptSerializer jss = new JavaScriptSerializer(); //序列化学生集合对象得到json字符 string studentsJson = jss.Serialize(studentSet); //将字符串响应到客户端 context.Response.Write(studentsJson); context.Response.End(); } public bool IsReusable { get { return false; } } } }
Select方法重新投影对象集合将Birthday属性转换成一个新的属性,注意属性变化后要重新命名,属性名可以相同;这里可以使用select方法也可以使用LINQ查询表达式,也可以选择别的方式达到相同的目的;这种办法可以将集合中客户端不用的属性剔除,达到简单优化性能的目的。
运行结果:
这时候的日期格式就已经变成友好格式了,不过在javascript中这只是一个字符串。
方法二:
在javascript中将"Birthday":"\/Date(1391141532000)\/"中的字符串转换成javascript中的日期对象,可以将Birthday这个Key所对应的Value中的非数字字符以替换的方式删除,到到一个数字1391141532000,然后实例化一个Date对象,将1391141532000毫秒作为参数,得到一个javascript中的日期对象,代码如下:
<!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <title>json日期格式处理</title> <script src="Scripts/jquery-1.10.2.min.js" type="text/javascript"></script> <script type="text/javascript"> $(function() { $.getJSON("getJson.ashx", function (students) { $.each(students, function (index, obj) { $("<li/>").html(obj.Name).appendTo("#ulStudents"); //使用正则表达式将生日属性中的非数字(\D)删除 //并把得到的毫秒数转换成数字类型 var birthdayMilliseconds = parseInt(obj.Birthday.replace(/\D/igm, "")); //实例化一个新的日期格式,使用1970 年 1 月 1 日至今的毫秒数为参数 var birthday = new Date(birthdayMilliseconds); $("<li/>").html(birthday.toLocaleString()).appendTo("#ulStudents"); ; }); }); }); </script> </head> <body> <h2>json日期格式处理</h2> <ul id="ulStudents"> </ul> </body> </html>
运行结果:
上的使用正则/\D/igm达到替换所有非数字的目的,\D表示非数字,igm是参数,分别表示忽视(ignore)大小写;多次、全局(global)替换;多行替换(multi-line);有一些时候还会出现+86的情况,只需要变换正则同样可以达到目的。另外如果项目中反复出现这种需要处理日期格式的问题,可以扩展一个javascript方法,代码如下:
$(function () { $.getJSON("getJson.ashx", function (students) { $.each(students, function (index, obj) { $("<li/>").html(obj.Name).appendTo("#ulStudents"); //使用正则表达式将生日属性中的非数字(\D)删除 //并把得到的毫秒数转换成数字类型 var birthdayMilliseconds = parseInt(obj.Birthday.replace(/\D/igm, "")); //实例化一个新的日期格式,使用1970 年 1 月 1 日至今的毫秒数为参数 var birthday = new Date(birthdayMilliseconds); $("<li/>").html(birthday.toLocaleString()).appendTo("#ulStudents"); $("<li/>").html(obj.Birthday.toDate()).appendTo("#ulStudents"); }); }); }); //在String对象中扩展一个toDate方法,可以根据要求完善 String.prototype.toDate = function () { var dateMilliseconds; if (isNaN(this)) { //使用正则表达式将日期属性中的非数字(\D)删除 dateMilliseconds =this.replace(/\D/igm, ""); } else { dateMilliseconds=this; } //实例化一个新的日期格式,使用1970 年 1 月 1 日至今的毫秒数为参数 return new Date(parseInt(dateMilliseconds)); };
上面扩展的方法toDate不一定合理,也不够强大,可以根据需要修改。
方法三:
可以选择一些第三方的json工具类,其中不乏有一些已经对日期格式问题已处理好了的,常见的json序列化与反序列化工具库有:
1.fastJSON.
2.JSON_checker.
3.Jayrock.
4.Json.NET - LINQ to JSON.
5.LitJSON.
6.JSON for .NET.
7.JsonFx.
8.JSONSharp.
9.JsonExSerializer.
10.fluent-json
11.Manatee Json
这里以litjson为序列化与反序列化json的工具类作示例,代码如下:
using System; using System.Collections.Generic; using System.Web; using LitJson; namespace JsonDate2 { using System.Linq; /// <summary> /// 学生类,测试用 /// </summary> public class Student { /// <summary> /// 姓名 /// </summary> public String Name { get; set; } /// <summary> /// 生日 /// </summary> public DateTime Birthday { get; set; } } /// <summary> /// 返回学生集合的json字符 /// </summary> public class GetJson : IHttpHandler { public void ProcessRequest(HttpContext context) { //设置服务器响应的结果为纯文本格式 context.Response.ContentType = "text/plain"; //学生对象集合 List<Student> students = new List<Student> { new Student(){Name ="Tom",Birthday =Convert.ToDateTime("2014-01-31 12:12:12")}, new Student(){Name ="Rose",Birthday =Convert.ToDateTime("2014-01-10 11:12:12")}, new Student(){Name ="Mark",Birthday =Convert.ToDateTime("2014-01-09 10:12:12")} }; //序列化学生集合对象得到json字符 string studentsJson = JsonMapper.ToJson(students); //将字符串响应到客户端 context.Response.Write(studentsJson); context.Response.End(); } public bool IsReusable { get { return false; } } } }
运行结果如下:
这时候的日期格式就基本正确了,只要在javascript中直接实例化日期就好了,
var date = new Date("01/31/2014 12:12:12"); alert(date.toLocaleString());
客户端的代码如下:
$(function () { $.getJSON("GetJson2.ashx", function (students) { $.each(students, function (index, obj) { $("<li/>").html(obj.Name).appendTo("#ulStudents"); var birthday = new Date(obj.Birthday); $("<li/>").html(birthday.toLocaleString()).appendTo("#ulStudents"); }); }); }); var date = new Date("01/31/2014 12:12:12"); alert(date.toLocaleString());
方法四:
这点文字发到博客上有网友提出了他们宝贵的意见,我并没有考虑在MVC中的情况,其实MVC中也可以使用handler,所以区别不是很大了,但MVC中有专门针对服务器响应为JSON的Action,代码如下:
using System; using System.Web.Mvc; namespace JSONDateMVC.Controllers { public class HomeController : Controller { public JsonResult GetJson1() { //序列化当前日期与时间对象,并允许客户端Get请求 return Json(DateTime.Now, JsonRequestBehavior.AllowGet); } } }
运行结果:
下载一个内容为Application/json的文件,文件名为GetJson1,内容是"\/Date(1391418272884)\/"
从上面的情况看来MVC中序列化时并未对日期格式特别处理,我们可以反编译看源码:
Return调用的Json方法:
protected internal JsonResult Json(object data, JsonRequestBehavior behavior) { return this.Json(data, null, null, behavior); } this.Json方法 protected internal virtual JsonResult Json(object data, string contentType, Encoding contentEncoding, JsonRequestBehavior behavior) { return new JsonResult { Data = data, ContentType = contentType, ContentEncoding = contentEncoding, JsonRequestBehavior = behavior }; }
JsonResult类ActionResult类的子类,ExecuteResult方法:
从上面的代码中不难看出微软的JsonResult类仍然是使用了JavaScriptSerializer,所以返回的结果与方法一未处理时是一样的,要解决这个问题我们可以派生出一个新的类,重写ExecuteResult方法,使用Json.net来完成序列化工作,JsonResultPro.cs文件的代码如下:
namespace JSONDateMVC.Common { using System; using System.Web; using System.Web.Mvc; using Newtonsoft.Json; using Newtonsoft.Json.Converters; public class JsonResultPro : JsonResult { public JsonResultPro(){} public JsonResultPro(object data, JsonRequestBehavior behavior) { base.Data = data; base.JsonRequestBehavior = behavior; this.DateTimeFormat = "yyyy-MM-dd hh:mm:ss"; } public JsonResultPro(object data, String dateTimeFormat) { base.Data = data; base.JsonRequestBehavior = JsonRequestBehavior.AllowGet; this.DateTimeFormat = dateTimeFormat; } /// <summary> /// 日期格式 /// </summary> public string DateTimeFormat{ get; set; } public override void ExecuteResult(ControllerContext context) { if (context == null) { throw new ArgumentNullException("context"); } if ((this.JsonRequestBehavior == JsonRequestBehavior.DenyGet) && string.Equals(context.HttpContext.Request.HttpMethod, "GET", StringComparison.OrdinalIgnoreCase)) { throw new InvalidOperationException("MvcResources.JsonRequest_GetNotAllowed"); } HttpResponseBase base2 = context.HttpContext.Response; if (!string.IsNullOrEmpty(this.ContentType)) { base2.ContentType = this.ContentType; } else { base2.ContentType = "application/json"; } if (this.ContentEncoding != null) { base2.ContentEncoding = this.ContentEncoding; } if (this.Data != null) { //转换System.DateTime的日期格式到 ISO 8601日期格式 //ISO 8601 (如2008-04-12T12:53Z) IsoDateTimeConverter isoDateTimeConverter=new IsoDateTimeConverter(); //设置日期格式 isoDateTimeConverter.DateTimeFormat = DateTimeFormat; //序列化 String jsonResult = JsonConvert.SerializeObject(this.Data,isoDateTimeConverter); //相应结果 base2.Write(jsonResult); } } } }
使用上面的JsonResultPro Action类型的代码如下:
public JsonResultPro GetJson2() { //序列化当前日期与时间对象,并允许客户端Get请求,注意H是大写 return new JsonResultPro(DateTime.Now,"yyyy-MM-dd HH:mm"); }
相信看了本文案例你已经掌握了方法,更多精彩请关注php中文网其它相关文章!
推荐阅读:
以上是Json操作日期格式的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
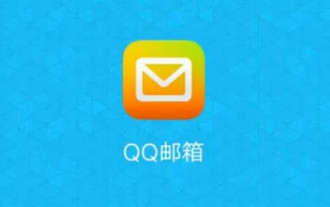
QQ邮箱:QQ号@qq.com,英文QQ邮箱:英文或数字@qq.com,foxmail邮箱账号:自己设置账号@foxmail.com,手机邮箱账号:手机号@qq.com。教程适用型号:iPhone13系统:IOS15.3版本:QQ邮箱6.3.3解析1QQ邮箱有四种格式,常用的QQ邮箱:QQ号@qq.com,英文QQ邮箱:英文或数字@qq.com,foxmail邮箱账号:自己设置账号@foxmail.com,手机邮箱账号:手机号@qq.com。补充:qq邮箱是什么1最早的QQ邮箱还只是QQ用户之间
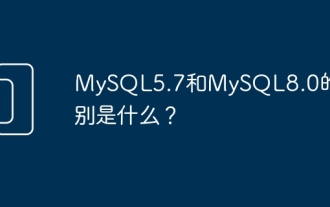
MySQL5.7和MySQL8.0是两个不同的MySQL数据库版本,它们之间有以下一些主要区别:性能改进:MySQL8.0相对于MySQL5.7有一些性能改进。其中包括更好的查询优化器、更高效的查询执行计划生成、更好的索引算法和并行查询等。这些改进可以提高查询性能和整体系统性能。JSON支持:MySQL8.0引入了对JSON数据类型的原生支持,包括JSON数据的存储、查询和索引。这使得在MySQL中处理和操作JSON数据变得更加方便和高效。事务特性:MySQL8.0引入了一些新的事务特性,如原子
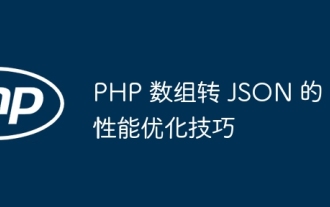
PHP数组转JSON的性能优化方法包括:使用JSON扩展和json_encode()函数;添加JSON_UNESCAPED_UNICODE选项以避免字符转义;使用缓冲区提高循环编码性能;缓存JSON编码结果;考虑使用第三方JSON编码库。
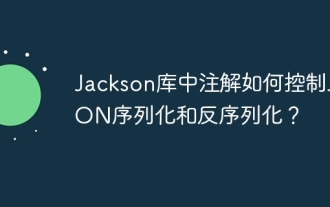
Jackson库中的注解可控制JSON序列化和反序列化:序列化:@JsonIgnore:忽略属性@JsonProperty:指定名称@JsonGetter:使用获取方法@JsonSetter:使用设置方法反序列化:@JsonIgnoreProperties:忽略属性@JsonProperty:指定名称@JsonCreator:使用构造函数@JsonDeserialize:自定义逻辑
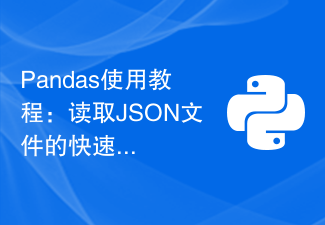
快速入门:Pandas读取JSON文件的方法,需要具体代码示例引言:在数据分析和数据科学领域,Pandas是一个重要的Python库之一。它提供了丰富的功能和灵活的数据结构,能够方便地对各种数据进行处理和分析。在实际应用中,我们经常会遇到需要读取JSON文件的情况。本文将介绍如何使用Pandas来读取JSON文件,并附上具体的代码示例。一、Pandas的安装
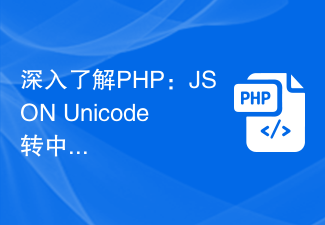
深入了解PHP:JSONUnicode转中文的实现方法在开发中,我们经常会遇到需要处理JSON数据的情况,而JSON中的Unicode编码在一些场景下会给我们带来一些问题,特别是当需要将Unicode编码转换为中文字符时。在PHP中,有一些方法可以帮助我们实现这个转换过程,下面将介绍一种常用的方法,并提供具体的代码示例。首先,让我们先了解一下JSON中Un
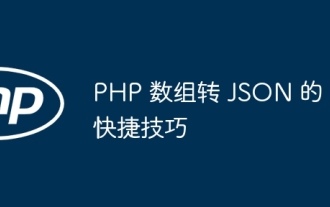
PHP数组可通过json_encode()函数转换为JSON字符串(例如:$json=json_encode($array);),反之亦可用json_decode()函数从JSON转换为数组($array=json_decode($json);)。其他技巧还包括:避免深度转换、指定自定义选项以及使用第三方库。
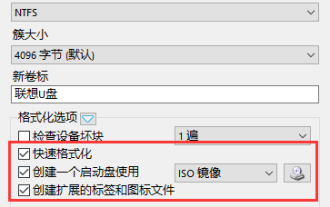
随着win10系统的不断更新,其原版安装镜像也越来越大,给喜欢使用U盘进行UEFI启动方式安装的同学带来了困扰,大家都知道,使用EFI方式安装系统,直接将微软原版镜像解压缩后,复制到fat32或fat16格式的U盘根目录,然后直接设置主板U盘启动就可以安装了,但是最近有很多小伙伴反映事与愿违,U盘使用fat格式有个限制,那就是单个文件最大不能超过4G,不然就会出现无法写入的情况,但是随着win10镜像的增大,其安装包里的install.wim文件也越来越大,已经超过了4G,这就导致了无法直接使用
