本篇文章带大家一起了解一下Nodejs内置模块的基本用法。有一定的参考价值,有需要的朋友可以参考一下,希望对大家有所帮助。
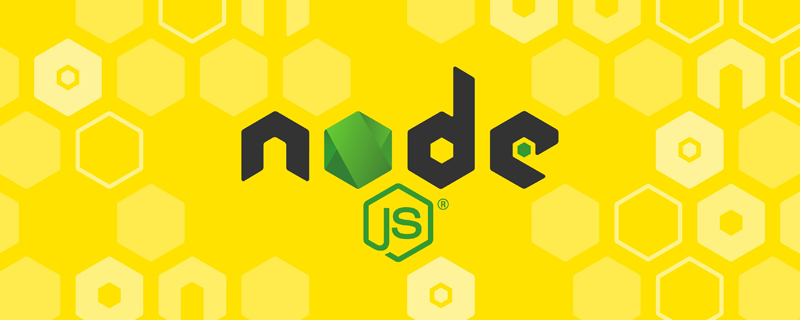
相关推荐:《nodejs 教程》
内置模块的基本使用
1 2 3 4 5 6 7 8 9 | const fs = require ( "fs" );
fs.unlink( "01-内置模块fs的使用/tmp/hello.txt" , (err) => {
if (err) throw err;
console.log( "已成功删除 /tmp/hello" );
});
|
登录后复制
fs模块的读文件
1 | fs.readFile(path[, options], callback)
|
登录后复制
第一个参数:文件的路径
第二个参数:可选参数,读取文件的编码格式
第三个参数:回调函数
1 2 3 4 5 6 | fs.readFile( "01-内置模块fs的使用/etc/passwd.txt" , "utf-8" , (err, data) => {
console.log(data);
});
|
登录后复制
Npdemon全局模块
作用:终端下自动执行代码
安装 npm i nodemon -g
nodemon
文件名即可
自动监视文件的修改,自动重新运行
vscode快捷键
ctrl+d 选择相同的下一个
ctrl+左右 按单词跳转光标
ctrl+enter 光标另起一行
同步异步
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 | console.log( "哈哈" );
for ( var i = 0; i < 10; i++) {
console.log(i);
}
console.log( "呵呵" ); ->
哈哈
0
1
2
3
4
5
6
7
8
9
10
呵呵
|
登录后复制
1 2 3 4 5 6 7 8 9 | console.log( "哈哈" );
setTimeout(() => {
console.log( "啦啦啦" );
}, 1000);
console.log( "呵呵" ); ->
哈哈
呵呵
啦啦啦
|
登录后复制
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 | console.log( "哈哈" );
const fs = require ( "fs" );
fs.readFile( "01-内置模块fs的使用/etc/望庐山瀑布.txt" , "utf-8" , (err, data) => {
if (err === null) {
console.log(data);
} else {
console.log(err);
}
});
console.log( "呵呵" ); ->
哈哈
呵呵
望庐山瀑布
唐·李白
日照香炉生紫烟,
遥看瀑布挂前川。
飞流直下三千尺,
疑是银河落九天。
|
登录后复制
1 2 3 4 5 6 7 8 9 | console.log( "哈哈" );
const fs = require ( "fs" );
let data = fs.readFileSync( "01-内置模块fs的使用/etc/passwd.txt" , "utf-8" );
console.log(data);
console.log( "呵呵" ); ->
哈哈
这是一个寂寞的天,下着有些伤心的雨!
呵呵
|
登录后复制
同步异步面试题
1 2 3 4 5 6 7 8 9 | var t = true;
while (t) {
window.setTimeout( function () {
t = false;
}, 1000);
}
alert( "end" ); -> 死循环
|
登录后复制
和路径相关的两个变量
- __dirname: 获现的是当前这个文件所在的整个文件夹的绝对路径
- __filename: 拿到的是当前这个文件的绝对路径
1 2 3 4 | console.log(__dirname);
console.log(__filename);
|
登录后复制
使用拼接的绝对路径来读取文件
1 2 3 4 5 6 7 8 9 | const fs = require ( "fs" );
const fullPath = __dirname + "\\etc\\1.txt" ;
fs.readFile(fullPath, "utf-8" , (err, data) => {
if (err === null) {
console.log(data);
} else {
console.log(err);
}
});
|
登录后复制
path.join([…paths])
path.join()
方法会将所有给定的 path
片段连接到一起(使用平台特定的分隔符作为定界符),然后规范化生成的路径。
长度为零的 path
片段会被忽略。 如果连接后的路径字符串为长度为零的字符串,则返回 '.'
,表示当前工作目录。
1 2 3 4 5 6 | const path = require ( "path" );
const fullPath = path.join(__dirname, "etc" , "1.txt" );
console.log(fullPath);
|
登录后复制
使用path模块拼接而成的绝对路径来读取文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | const fs = require ( "fs" );
const path = require ( "path" );
const fullPath = path.join(__dirname, "etc" , "1.txt" );
fs.readFile(fullPath, "utf-8" , (err, data) => {
if (err === null) {
console.log(data);
} else {
console.log(err);
}
});
|
登录后复制
使用内置模块http来创建一个服务器

1 2 3 4 5 6 7 8 9 10 11 12 13 14 | const http = require ( "http" );
const server = http.createServer(( require , response) => {
response. end ( "hello world!" );
});
server.listen(8087, () => {
console.log( "服务器开启了:8087" );
});
|
登录后复制
request.setHeader(name, value)
为请求头对象设置单个请求头的值。 如果此请求头已存在于待发送的请求头中,则其值将被替换。 这里可以使用字符串数组来发送具有相同名称的多个请求头。 非字符串值将被原样保存。 因此 request.getHeader()
可能会返回非字符串值。 但是非字符串值将转换为字符串以进行网络传输。
1 | request.setHeader( 'Content-Type' , 'application/json' );
|
登录后复制
或:
1 | request.setHeader( 'Cookie' , [ 'type=ninja' , 'language=javascript' ]);
|
登录后复制
如果想要返回去的中文不乱码,那就要设置响应头.
1 | response.setHeader( "Content-Type" , "text/html;charset=utf-8" );
|
登录后复制
更多编程相关知识,请访问:编程视频!!
以上是浅谈Nodejs中内置模块的基本用法的详细内容。更多信息请关注PHP中文网其他相关文章!