SpringBoot+Elasticsearch如何实现数据搜索
一、简介
SpringBoot 连接 ElasticSearch,主流的方式有以下四种方式
方式一:通过Elastic Transport Client客户端连接 es 服务器,底层基于 TCP 协议通过 transport 模块和远程 ES 服务端通信,不过,从 V7.0 开始官方不建议使用,V8.0开始正式移除。
方式二:通过Elastic Java Low Level Rest Client客户端连接 es 服务器,底层基于 HTTP 协议通过 restful API 来和远程 ES 服务端通信,只提供了最简单最基本的 API,类似于上篇文章中给大家介绍的 API 操作逻辑。
方式三:通过Elastic Java High Level Rest Client客户端连接 es 服务器,底层基于Elastic Java Low Level Rest Client客户端做了一层封装,提供了更高级得 API 且和Elastic Transport Client接口及参数保持一致,官方推荐的 es 客户端。
方式四:通过JestClient客户端连接 es 服务器,这是开源社区基于 HTTP 协议开发的一款 es 客户端,官方宣称接口及代码设计比 ES 官方提供的 Rest 客户端更简洁、更合理,更好用,具有一定的 ES 服务端版本兼容性,但是更新速度不是很快,目前 ES 版本已经出到 V7.9,但是JestClient只支持 V1.0~V6.X 版 本的 ES。
还有一个需要大家注意的地方,那就是版本号的兼容!
在开发过程中,大家尤其需要关注一下客户端和服务端的版本号,要尽可能保持一致,比如服务端 es 的版本号是6.8.2,那么连接 es 的客户端版本号,最好也是6.8.2,即使因项目的原因不能保持一致,客户端的版本号必须在6.0.0 ~6.8.2,不要超过服务器的版本号,这样客户端才能保持正常工作,否则会出现很多意想不到的问题,假如客户端是7.0.4的版本号,此时的程序会各种报错,甚至没办法用!
为什么要这样做呢?主要原因就是 es 的服务端,高版本不兼容低版本;es6 和 es7 的某些 API 请求参数结构有着很大的区别,所以客户端和服务端版本号尽量保持一致。
废话也不多说了,直接上代码!
二、代码实践
本文采用的SpringBoot版本号是2.1.0.RELEASE,服务端 es 的版本号是6.8.2,客户端采用的是官方推荐的Elastic Java High Level Rest Client版本号是6.4.2,方便与SpringBoot的版本兼容。
2.1、导入依赖
<!--elasticsearch--> <dependency> <groupId>org.elasticsearch</groupId> <artifactId>elasticsearch</artifactId> <version>6.4.2</version> </dependency> <dependency> <groupId>org.elasticsearch.client</groupId> <artifactId>elasticsearch-rest-client</artifactId> <version>6.4.2</version> </dependency> <dependency> <groupId>org.elasticsearch.client</groupId> <artifactId>elasticsearch-rest-high-level-client</artifactId> <version>6.4.2</version> </dependency>
2.2、配置环境变量
在application.properties全局配置文件中,配置elasticsearch自定义环境变量。
elasticsearch.scheme=http elasticsearch.address=127.0.0.1:9200 elasticsearch.userName= elasticsearch.userPwd= elasticsearch.socketTimeout=5000 elasticsearch.connectTimeout=5000 elasticsearch.connectionRequestTimeout=5000
2.3、创建 elasticsearch 的 config 类
@Configuration public class ElasticsearchConfiguration { private static final Logger log = LoggerFactory.getLogger(ElasticsearchConfiguration.class); private static final int ADDRESS_LENGTH = 2; @Value("${elasticsearch.scheme:http}") private String scheme; @Value("${elasticsearch.address}") private String address; @Value("${elasticsearch.userName}") private String userName; @Value("${elasticsearch.userPwd}") private String userPwd; @Value("${elasticsearch.socketTimeout:5000}") private Integer socketTimeout; @Value("${elasticsearch.connectTimeout:5000}") private Integer connectTimeout; @Value("${elasticsearch.connectionRequestTimeout:5000}") private Integer connectionRequestTimeout; /** * 初始化客户端 * @return */ @Bean(name = "restHighLevelClient") public RestHighLevelClient restClientBuilder() { HttpHost[] hosts = Arrays.stream(address.split(",")) .map(this::buildHttpHost) .filter(Objects::nonNull) .toArray(HttpHost[]::new); RestClientBuilder restClientBuilder = RestClient.builder(hosts); // 异步参数配置 restClientBuilder.setHttpClientConfigCallback(httpClientBuilder -> { httpClientBuilder.setDefaultCredentialsProvider(buildCredentialsProvider()); return httpClientBuilder; }); // 异步连接延时配置 restClientBuilder.setRequestConfigCallback(requestConfigBuilder -> { requestConfigBuilder.setConnectionRequestTimeout(connectionRequestTimeout); requestConfigBuilder.setSocketTimeout(socketTimeout); requestConfigBuilder.setConnectTimeout(connectTimeout); return requestConfigBuilder; }); return new RestHighLevelClient(restClientBuilder); } /** * 根据配置创建HttpHost * @param s * @return */ private HttpHost buildHttpHost(String s) { String[] address = s.split(":"); if (address.length == ADDRESS_LENGTH) { String ip = address[0]; int port = Integer.parseInt(address[1]); return new HttpHost(ip, port, scheme); } else { return null; } } /** * 构建认证服务 * @return */ private CredentialsProvider buildCredentialsProvider(){ final CredentialsProvider credentialsProvider = new BasicCredentialsProvider(); credentialsProvider.setCredentials(AuthScope.ANY, new UsernamePasswordCredentials(userName, userPwd)); return credentialsProvider; } }
至此,客户端配置完毕,项目启动的时候,会自动注入到Spring的ioc容器里面。
2.4、索引管理
es 中最重要的就是索引库,客户端如何创建呢?请看下文!
创建索引
@RunWith(SpringJUnit4ClassRunner.class) @SpringBootTest(classes = ElasticSearchApplication.class) public class IndexJunit { @Autowired private RestHighLevelClient client; /** * 创建索引(简单模式) * @throws IOException */ @Test public void createIndex() throws IOException { CreateIndexRequest request = new CreateIndexRequest("cs_index"); CreateIndexResponse response = client.indices().create(request, RequestOptions.DEFAULT); System.out.println(response.isAcknowledged()); } /** * 创建索引(复杂模式) * 可以直接把对应的文档结构也一并初始化 * @throws IOException */ @Test public void createIndexComplete() throws IOException { CreateIndexRequest request = new CreateIndexRequest(); //索引名称 request.index("cs_index"); //索引配置 Settings settings = Settings.builder() .put("index.number_of_shards", 3) .put("index.number_of_replicas", 1) .build(); request.settings(settings); //映射结构字段 Map<String, Object> properties = new HashMap(); properties.put("id", ImmutableBiMap.of("type", "text")); properties.put("name", ImmutableBiMap.of("type", "text")); properties.put("sex", ImmutableBiMap.of("type", "text")); properties.put("age", ImmutableBiMap.of("type", "long")); properties.put("city", ImmutableBiMap.of("type", "text")); properties.put("createTime", ImmutableBiMap.of("type", "long")); Map<String, Object> mapping = new HashMap<>(); mapping.put("properties", properties); //添加一个默认类型 System.out.println(JSON.toJSONString(request)); request.mapping("_doc",mapping); CreateIndexResponse response = client.indices().create(request, RequestOptions.DEFAULT); System.out.println(response.isAcknowledged()); } }
删除索引
@RunWith(SpringJUnit4ClassRunner.class) @SpringBootTest(classes = ElasticSearchApplication.class) public class IndexJunit { @Autowired private RestHighLevelClient client; /** * 删除索引 * @throws IOException */ @Test public void deleteIndex() throws IOException { DeleteIndexRequest request = new DeleteIndexRequest("cs_index1"); AcknowledgedResponse response = client.indices().delete(request, RequestOptions.DEFAULT); System.out.println(response.isAcknowledged()); } }
查询索引
@RunWith(SpringJUnit4ClassRunner.class) @SpringBootTest(classes = ElasticSearchApplication.class) public class IndexJunit { @Autowired private RestHighLevelClient client; /** * 查询索引 * @throws IOException */ @Test public void getIndex() throws IOException { // 创建请求 GetIndexRequest request = new GetIndexRequest(); request.indices("cs_index"); // 执行请求,获取响应 GetIndexResponse response = client.indices().get(request, RequestOptions.DEFAULT); System.out.println(response.toString()); } }
查询索引是否存在
@RunWith(SpringJUnit4ClassRunner.class) @SpringBootTest(classes = ElasticSearchApplication.class) public class IndexJunit { @Autowired private RestHighLevelClient client; /** * 检查索引是否存在 * @throws IOException */ @Test public void exists() throws IOException { // 创建请求 GetIndexRequest request = new GetIndexRequest(); request.indices("cs_index"); // 执行请求,获取响应 boolean response = client.indices().exists(request, RequestOptions.DEFAULT); System.out.println(response); } }
查询所有的索引名称
@RunWith(SpringJUnit4ClassRunner.class) @SpringBootTest(classes = ElasticSearchApplication.class) public class IndexJunit { @Autowired private RestHighLevelClient client; /** * 查询所有的索引名称 * @throws IOException */ @Test public void getAllIndices() throws IOException { GetAliasesRequest request = new GetAliasesRequest(); GetAliasesResponse response = client.indices().getAlias(request,RequestOptions.DEFAULT); Map<String, Set<AliasMetaData>> map = response.getAliases(); Set<String> indices = map.keySet(); for (String key : indices) { System.out.println(key); } } }
查询索引映射字段
@RunWith(SpringJUnit4ClassRunner.class) @SpringBootTest(classes = ElasticSearchApplication.class) public class IndexJunit { @Autowired private RestHighLevelClient client; /** * 查询索引映射字段 * @throws IOException */ @Test public void getMapping() throws IOException { GetMappingsRequest request = new GetMappingsRequest(); request.indices("cs_index"); request.types("_doc"); GetMappingsResponse response = client.indices().getMapping(request, RequestOptions.DEFAULT); System.out.println(response.toString()); } }
添加索引映射字段
@RunWith(SpringJUnit4ClassRunner.class) @SpringBootTest(classes = ElasticSearchApplication.class) public class IndexJunit { @Autowired private RestHighLevelClient client; /** * 添加索引映射字段 * @throws IOException */ @Test public void addMapping() throws IOException { PutMappingRequest request = new PutMappingRequest(); request.indices("cs_index"); request.type("_doc"); //添加字段 Map<String, Object> properties = new HashMap(); properties.put("accountName", ImmutableBiMap.of("type", "keyword")); Map<String, Object> mapping = new HashMap<>(); mapping.put("properties", properties); request.source(mapping); PutMappingResponse response = client.indices().putMapping(request, RequestOptions.DEFAULT); System.out.println(response.isAcknowledged()); } }
2.5、文档管理
所谓文档,就是向索引里面添加数据,方便进行数据查询,详细操作内容,请看下文!
添加文档
ublic class UserDocument { private String id; private String name; private String sex; private Integer age; private String city; private Date createTime; //省略get、set... }
@RunWith(SpringJUnit4ClassRunner.class) @SpringBootTest(classes = ElasticSearchApplication.class) public class DocJunit { @Autowired private RestHighLevelClient client; /** * 添加文档 * @throws IOException */ @Test public void addDocument() throws IOException { // 创建对象 UserDocument user = new UserDocument(); user.setId("1"); user.setName("里斯"); user.setCity("武汉"); user.setSex("男"); user.setAge(20); user.setCreateTime(new Date()); // 创建索引,即获取索引 IndexRequest request = new IndexRequest(); // 外层参数 request.id("1"); request.index("cs_index"); request.type("_doc"); request.timeout(TimeValue.timeValueSeconds(1)); // 存入对象 request.source(JSON.toJSONString(user), XContentType.JSON); // 发送请求 System.out.println(request.toString()); IndexResponse response = client.index(request, RequestOptions.DEFAULT); System.out.println(response.toString()); } }
更新文档
@RunWith(SpringJUnit4ClassRunner.class) @SpringBootTest(classes = ElasticSearchApplication.class) public class DocJunit { @Autowired private RestHighLevelClient client; /** * 更新文档(按需修改) * @throws IOException */ @Test public void updateDocument() throws IOException { // 创建对象 UserDocument user = new UserDocument(); user.setId("2"); user.setName("程咬金"); user.setCreateTime(new Date()); // 创建索引,即获取索引 UpdateRequest request = new UpdateRequest(); // 外层参数 request.id("2"); request.index("cs_index"); request.type("_doc"); request.timeout(TimeValue.timeValueSeconds(1)); // 存入对象 request.doc(JSON.toJSONString(user), XContentType.JSON); // 发送请求 System.out.println(request.toString()); UpdateResponse response = client.update(request, RequestOptions.DEFAULT); System.out.println(response.toString()); } }
删除文档
@RunWith(SpringJUnit4ClassRunner.class) @SpringBootTest(classes = ElasticSearchApplication.class) public class DocJunit { @Autowired private RestHighLevelClient client; /** * 删除文档 * @throws IOException */ @Test public void deleteDocument() throws IOException { // 创建索引,即获取索引 DeleteRequest request = new DeleteRequest(); // 外层参数 request.id("1"); request.index("cs_index"); request.type("_doc"); request.timeout(TimeValue.timeValueSeconds(1)); // 发送请求 System.out.println(request.toString()); DeleteResponse response = client.delete(request, RequestOptions.DEFAULT); System.out.println(response.toString()); } }
查询文档是不是存在
@RunWith(SpringJUnit4ClassRunner.class) @SpringBootTest(classes = ElasticSearchApplication.class) public class DocJunit { @Autowired private RestHighLevelClient client; /** * 查询文档是不是存在 * @throws IOException */ @Test public void exists() throws IOException { // 创建索引,即获取索引 GetRequest request = new GetRequest(); // 外层参数 request.id("3"); request.index("cs_index"); request.type("_doc"); // 发送请求 System.out.println(request.toString()); boolean response = client.exists(request, RequestOptions.DEFAULT); System.out.println(response); } }
通过 ID 查询指定文档
@RunWith(SpringJUnit4ClassRunner.class) @SpringBootTest(classes = ElasticSearchApplication.class) public class DocJunit { @Autowired private RestHighLevelClient client; /** * 通过ID,查询指定文档 * @throws IOException */ @Test public void getById() throws IOException { // 创建索引,即获取索引 GetRequest request = new GetRequest(); // 外层参数 request.id("1"); request.index("cs_index"); request.type("_doc"); // 发送请求 System.out.println(request.toString()); GetResponse response = client.get(request, RequestOptions.DEFAULT); System.out.println(response.toString()); } }
批量添加文档
@RunWith(SpringJUnit4ClassRunner.class) @SpringBootTest(classes = ElasticSearchApplication.class) public class DocJunit { @Autowired private RestHighLevelClient client; /** * 批量添加文档 * @throws IOException */ @Test public void batchAddDocument() throws IOException { // 批量请求 BulkRequest bulkRequest = new BulkRequest(); bulkRequest.timeout(TimeValue.timeValueSeconds(10)); // 创建对象 List<UserDocument> userArrayList = new ArrayList<>(); userArrayList.add(new UserDocument("张三", "男", 30, "武汉")); userArrayList.add(new UserDocument("里斯", "女", 31, "北京")); userArrayList.add(new UserDocument("王五", "男", 32, "武汉")); userArrayList.add(new UserDocument("赵六", "女", 33, "长沙")); userArrayList.add(new UserDocument("七七", "男", 34, "武汉")); // 添加请求 for (int i = 0; i < userArrayList.size(); i++) { userArrayList.get(i).setId(String.valueOf(i)); IndexRequest indexRequest = new IndexRequest(); // 外层参数 indexRequest.id(String.valueOf(i)); indexRequest.index("cs_index"); indexRequest.type("_doc"); indexRequest.timeout(TimeValue.timeValueSeconds(1)); indexRequest.source(JSON.toJSONString(userArrayList.get(i)), XContentType.JSON); bulkRequest.add(indexRequest); } // 执行请求 BulkResponse response = client.bulk(bulkRequest, RequestOptions.DEFAULT); System.out.println(response.status()); } }
以上是SpringBoot+Elasticsearch如何实现数据搜索的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
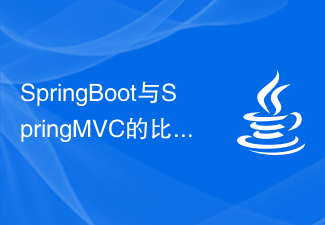
SpringBoot和SpringMVC都是Java开发中常用的框架,但它们之间有一些明显的差异。本文将探究这两个框架的特点和用途,并对它们的差异进行比较。首先,我们来了解一下SpringBoot。SpringBoot是由Pivotal团队开发的,它旨在简化基于Spring框架的应用程序的创建和部署。它提供了一种快速、轻量级的方式来构建独立的、可执行

本文来写个详细的例子来说下dubbo+nacos+Spring Boot开发实战。本文不会讲述太多的理论的知识,会写一个最简单的例子来说明dubbo如何与nacos整合,快速搭建开发环境。
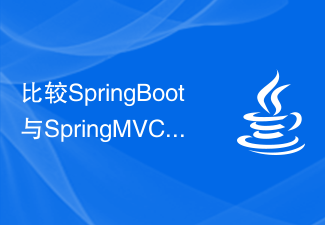
SpringBoot与SpringMVC的不同之处在哪里?SpringBoot和SpringMVC是两个非常流行的Java开发框架,用于构建Web应用程序。尽管它们经常分别被使用,但它们之间的不同之处也是很明显的。首先,SpringBoot可以被看作是一个Spring框架的扩展或者增强版。它旨在简化Spring应用程序的初始化和配置过程,以帮助开发人
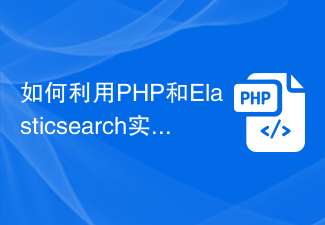
如何利用PHP和Elasticsearch实现高亮搜索结果引言:在现代的互联网世界中,搜索引擎已成为人们获取信息的主要途径。为了提高搜索结果的可读性和用户体验,高亮显示搜索关键字已成为一种常见的需求。本文将介绍如何使用PHP和Elasticsearch来实现高亮搜索结果。一、准备工作在开始之前,我们需要确保已正确安装和配置PHP和Elasticsearch。
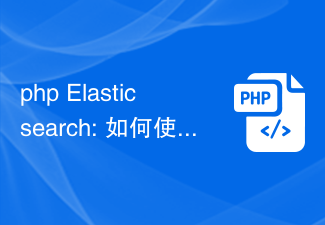
PHPElasticsearch:如何使用动态映射来实现灵活的搜索功能?引言:在开发现代化的应用程序时,搜索功能是一个不可或缺的部分。Elasticsearch是一个强大的搜索和分析引擎,提供了丰富的功能和灵活的数据建模方式。在本文中,我们将重点介绍如何使用动态映射来实现灵活的搜索功能。一、动态映射简介在Elasticsearch中,映射(mapp
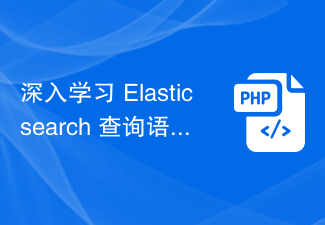
深入学习Elasticsearch查询语法与实战引言:Elasticsearch是一款基于Lucene的开源搜索引擎,主要用于分布式搜索与分析,广泛应用于大规模数据的全文搜索、日志分析、推荐系统等场景。在使用Elasticsearch进行数据查询时,灵活运用查询语法是提高查询效率的关键。本文将深入探讨Elasticsearch查询语法,并结合实际案例给出
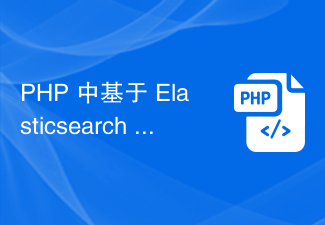
PHP中基于Elasticsearch的日志分析与异常监控概要:本文将介绍如何使用Elasticsearch数据库来进行日志分析和异常监控。通过简洁的PHP代码示例,展示了如何连接Elasticsearch数据库、将日志数据写入数据库,并使用Elasticsearch的强大查询功能来分析和监控日志中的异常情况。介绍:日志分析和异常监控是
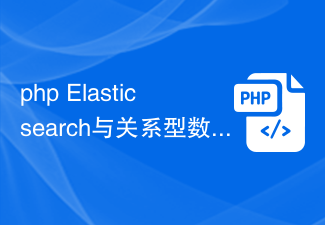
PHPElasticsearch与关系型数据库的集成实践指南引言:随着互联网和大数据时代的到来,数据的存储和处理方式也在不断发展。传统的关系型数据库在面对海量数据、高并发读写、全文搜索等场景时逐渐显示出了一些不足。而Elasticsearch作为一款实时分布式搜索和分析引擎,通过其高性能的全文搜索、实时分析和数据可视化等功能,逐渐受到了业界的关注和使用。然
