如何用PHP实现模拟退火算法
如何用PHP实现模拟退火算法
简介:
模拟退火算法(Simulated Annealing)是一种常用的全局优化算法,它通过模拟物质退火过程中的行为来寻找问题的最优解。它可以克服局部最优解的问题,可以应用于很多优化问题,如旅行商问题、背包问题等。本文将介绍如何用PHP实现模拟退火算法,并给出代码示例。
算法步骤:
- 初始化参数 - 设置初始温度、终止温度、降温速率、当前状态等。
- 生成邻域解 - 根据当前状态生成一个邻域解。
- 计算函数值 - 计算邻域解的函数值。
- 判断是否接受邻域解 - 通过计算接受概率来判断是否接受邻域解。
- 更新当前状态 - 根据接受与否来更新当前状态。
- 降温 - 更新温度值,减小温度。
- 迭代 - 重复以上步骤,直到满足终止条件。
示例代码:
<?php function simulatedAnnealing($initState, $initTemp, $finalTemp, $coolRate) { $currentTemp = $initTemp; $currentState = $initState; $bestState = $initState; $currentEnergy = calculateEnergy($currentState); $bestEnergy = $currentEnergy; while ($currentTemp > $finalTemp) { $newState = generateNeighbor($currentState); $newEnergy = calculateEnergy($newState); $energyDifference = $newEnergy - $currentEnergy; if ($energyDifference < 0) { $currentState = $newState; $currentEnergy = $newEnergy; if ($newEnergy < $bestEnergy) { $bestState = $newState; $bestEnergy = $newEnergy; } } else { $random = mt_rand() / mt_getrandmax(); $acceptProbability = exp(-$energyDifference / $currentTemp); if ($random < $acceptProbability) { $currentState = $newState; $currentEnergy = $newEnergy; } } $currentTemp *= $coolRate; } return $bestState; } function calculateEnergy($state) { // 计算函数值,根据具体问题进行定义 // 这里以一个简单的函数为例 $x = $state; $energy = pow($x, 2) - 10 * cos(2 * M_PI * $x); return $energy; } function generateNeighbor($state) { // 生成邻域解,根据具体问题进行定义 // 这里以一个简单的生成随机数的方式为例 $neighbor = $state + (mt_rand() / mt_getrandmax()) * 2 - 1; return $neighbor; } // 示例调用 $initState = 0; $initTemp = 100; $finalTemp = 0.1; $coolRate = 0.9; $bestState = simulatedAnnealing($initState, $initTemp, $finalTemp, $coolRate); echo "Best state: " . $bestState . " "; echo "Best energy: " . calculateEnergy($bestState) . " "; ?>
本例中,模拟退火算法用于求解一个简单的函数的最小值。通过调用simulatedAnnealing
函数传入初始状态、初始温度、终止温度和降温速率等参数,即可得到最优解。
总结:
本文介绍了如何用PHP实现模拟退火算法,并给出了一个简单的函数优化问题的代码示例。通过该示例,可以理解和掌握模拟退火算法的基本原理和实现过程。在实际应用中,可以根据具体问题进行相应的函数值计算和邻域解生成。希望本文能对想要了解和应用模拟退火算法的读者提供帮助。
以上是如何用PHP实现模拟退火算法的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)

热门话题
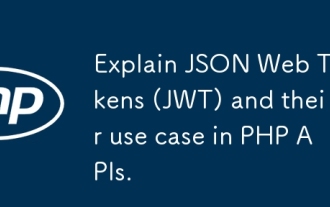
JWT是一种基于JSON的开放标准,用于在各方之间安全地传输信息,主要用于身份验证和信息交换。1.JWT由Header、Payload和Signature三部分组成。2.JWT的工作原理包括生成JWT、验证JWT和解析Payload三个步骤。3.在PHP中使用JWT进行身份验证时,可以生成和验证JWT,并在高级用法中包含用户角色和权限信息。4.常见错误包括签名验证失败、令牌过期和Payload过大,调试技巧包括使用调试工具和日志记录。5.性能优化和最佳实践包括使用合适的签名算法、合理设置有效期、
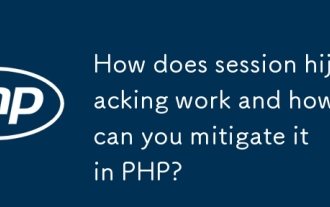
会话劫持可以通过以下步骤实现:1.获取会话ID,2.使用会话ID,3.保持会话活跃。在PHP中防范会话劫持的方法包括:1.使用session_regenerate_id()函数重新生成会话ID,2.通过数据库存储会话数据,3.确保所有会话数据通过HTTPS传输。
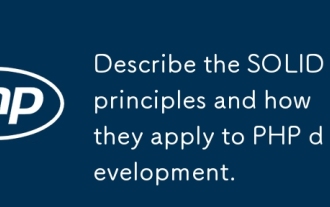
SOLID原则在PHP开发中的应用包括:1.单一职责原则(SRP):每个类只负责一个功能。2.开闭原则(OCP):通过扩展而非修改实现变化。3.里氏替换原则(LSP):子类可替换基类而不影响程序正确性。4.接口隔离原则(ISP):使用细粒度接口避免依赖不使用的方法。5.依赖倒置原则(DIP):高低层次模块都依赖于抽象,通过依赖注入实现。
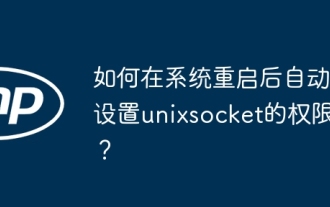
如何在系统重启后自动设置unixsocket的权限每次系统重启后,我们都需要执行以下命令来修改unixsocket的权限:sudo...
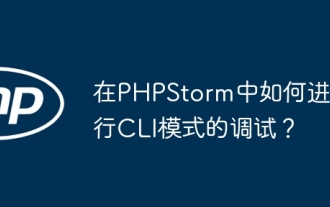
在PHPStorm中如何进行CLI模式的调试?在使用PHPStorm进行开发时,有时我们需要在命令行界面(CLI)模式下调试PHP�...
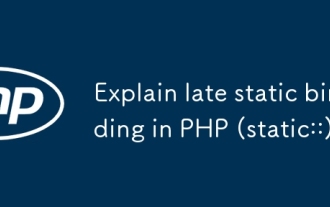
静态绑定(static::)在PHP中实现晚期静态绑定(LSB),允许在静态上下文中引用调用类而非定义类。1)解析过程在运行时进行,2)在继承关系中向上查找调用类,3)可能带来性能开销。
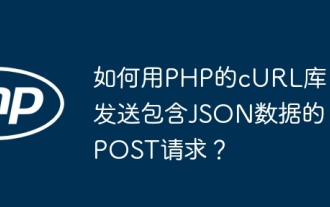
使用PHP的cURL库发送JSON数据在PHP开发中,经常需要与外部API进行交互,其中一种常见的方式是使用cURL库发送POST�...
