如何使用PHP和SOAP实现Web服务的身份验证和授权
如何使用PHP和SOAP实现Web服务的身份验证和授权
在当前互联网环境下,很多网站都提供了Web服务供其他应用程序调用。为了保证只有合法的用户能够访问这些Web服务,身份验证和授权的功能变得尤为重要。本文将介绍如何使用PHP和SOAP实现Web服务的身份验证和授权,并通过代码示例加深理解。
一、什么是SOAP?
SOAP全称为"Simple Object Access Protocol",是一种用于交换结构化信息的轻量级协议。它基于XML,可用于在分布式环境中进行应用程序间通信。在Web服务中,SOAP是常用的协议。
二、身份验证和授权
身份验证是确保用户是合法用户的过程,通常需要用户提供用户名和密码。而授权则是基于用户身份验证的结果,判断该用户是否有权访问某些资源。
三、使用SOAP实现Web服务的身份验证和授权
- 首先,我们需要创建一个SOAP服务器。
<?php // 创建SOAP服务器 $server = new SoapServer("service.wsdl"); // 注册Web服务方法 $server->addFunction("authenticate"); $server->addFunction("authorize"); // 处理请求 $server->handle(); ?>
- 接下来,我们需要编写身份验证和授权的函数。
<?php // 身份验证函数 function authenticate($username, $password) { // 身份验证逻辑,比如检查用户名和密码是否正确 if ($username === "admin" && $password === "123456") { return true; } else { return false; } } // 授权函数 function authorize($username, $serviceName) { // 授权逻辑,比如检查用户是否有权限访问某个Web服务 if ($username === "admin" && $serviceName === "service1") { return true; } else { return false; } } ?>
- 在SOAP服务器中注册这些函数。
<?php // 创建SOAP服务器 $server = new SoapServer("service.wsdl"); // 注册Web服务方法 $server->addFunction("authenticate"); $server->addFunction("authorize"); // 处理请求 $server->handle(); ?>
- 最后,我们需要定义Web服务的WSDL文件。
<?xml version="1.0"?> <definitions name="authenticationService" targetNamespace="http://example.com/authentication" xmlns:tns="http://example.com/authentication" xmlns:soap="http://schemas.xmlsoap.org/wsdl/soap/" xmlns="http://schemas.xmlsoap.org/wsdl/"> <!-- 身份验证方法 --> <portType name="authenticationPortType"> <operation name="authenticate"> <input message="tns:authenticateRequest"/> <output message="tns:authenticateResponse"/> </operation> </portType> <!-- 授权方法 --> <portType name="authorizationPortType"> <operation name="authorize"> <input message="tns:authorizeRequest"/> <output message="tns:authorizeResponse"/> </operation> </portType> <!-- 输入和输出消息定义 --> <message name="authenticateRequest"> <part name="username" type="xsd:string"/> <part name="password" type="xsd:string"/> </message> <message name="authenticateResponse"> <part name="result" type="xsd:boolean"/> </message> <message name="authorizeRequest"> <part name="serviceName" type="xsd:string"/> </message> <message name="authorizeResponse"> <part name="result" type="xsd:boolean"/> </message> <!-- SOAP绑定 --> <binding name="authenticationBinding" type="tns:authenticationPortType"> <soap:binding style="rpc" transport="http://schemas.xmlsoap.org/soap/http"/> <operation name="authenticate"> <soap:operation soapAction="authenticate"/> <input> <soap:body use="encoded" namespace="urn:examples" encodingStyle="http://schemas.xmlsoap.org/soap/encoding/"/> </input> <output> <soap:body use="encoded" namespace="urn:examples" encodingStyle="http://schemas.xmlsoap.org/soap/encoding/"/> </output> </operation> </binding> <binding name="authorizationBinding" type="tns:authorizationPortType"> <soap:binding style="rpc" transport="http://schemas.xmlsoap.org/soap/http"/> <operation name="authorize"> <soap:operation soapAction="authorize"/> <input> <soap:body use="encoded" namespace="urn:examples" encodingStyle="http://schemas.xmlsoap.org/soap/encoding/"/> </input> <output> <soap:body use="encoded" namespace="urn:examples" encodingStyle="http://schemas.xmlsoap.org/soap/encoding/"/> </output> </operation> </binding> <!-- 服务定义 --> <service name="authenticationService"> <port name="authenticationPort" binding="tns:authenticationBinding"> <soap:address location="http://example.com/authentication"/> </port> <port name="authorizationPort" binding="tns:authorizationBinding"> <soap:address location="http://example.com/authorization"/> </port> </service> </definitions>
通过以上步骤,我们就实现了基于PHP和SOAP的Web服务的身份验证和授权功能。在客户端调用这些Web服务时,只需要构造合适的SOAP请求,并处理返回的SOAP响应即可。
总结:身份验证和授权是任何一个Web服务都需要考虑的重要方面。使用PHP和SOAP实现身份验证和授权可以帮助我们保护Web服务,只让合法的用户能够访问。本文介绍了如何使用PHP和SOAP实现Web服务的身份验证和授权,并提供了相应的代码示例。希望读者能够通过此文理解并应用于实际项目中。
以上是如何使用PHP和SOAP实现Web服务的身份验证和授权的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
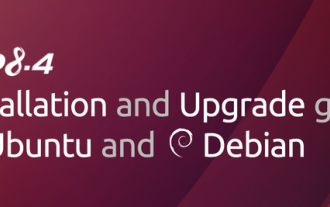
PHP 8.4 带来了多项新功能、安全性改进和性能改进,同时弃用和删除了大量功能。 本指南介绍了如何在 Ubuntu、Debian 或其衍生版本上安装 PHP 8.4 或升级到 PHP 8.4
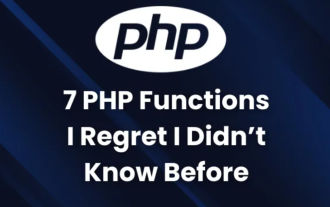
如果您是一位经验丰富的 PHP 开发人员,您可能会感觉您已经在那里并且已经完成了。您已经开发了大量的应用程序,调试了数百万行代码,并调整了一堆脚本来实现操作
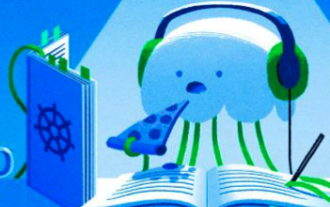
Visual Studio Code,也称为 VS Code,是一个免费的源代码编辑器 - 或集成开发环境 (IDE) - 可用于所有主要操作系统。 VS Code 拥有针对多种编程语言的大量扩展,可以轻松编写
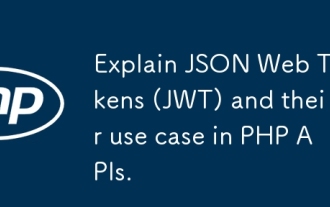
JWT是一种基于JSON的开放标准,用于在各方之间安全地传输信息,主要用于身份验证和信息交换。1.JWT由Header、Payload和Signature三部分组成。2.JWT的工作原理包括生成JWT、验证JWT和解析Payload三个步骤。3.在PHP中使用JWT进行身份验证时,可以生成和验证JWT,并在高级用法中包含用户角色和权限信息。4.常见错误包括签名验证失败、令牌过期和Payload过大,调试技巧包括使用调试工具和日志记录。5.性能优化和最佳实践包括使用合适的签名算法、合理设置有效期、
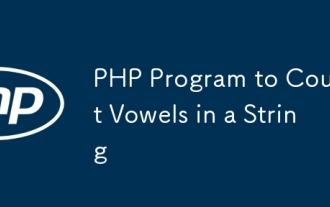
字符串是由字符组成的序列,包括字母、数字和符号。本教程将学习如何使用不同的方法在PHP中计算给定字符串中元音的数量。英语中的元音是a、e、i、o、u,它们可以是大写或小写。 什么是元音? 元音是代表特定语音的字母字符。英语中共有五个元音,包括大写和小写: a, e, i, o, u 示例 1 输入:字符串 = "Tutorialspoint" 输出:6 解释 字符串 "Tutorialspoint" 中的元音是 u、o、i、a、o、i。总共有 6 个元
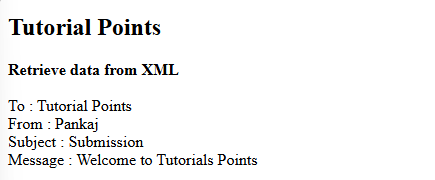
本教程演示了如何使用PHP有效地处理XML文档。 XML(可扩展的标记语言)是一种用于人类可读性和机器解析的多功能文本标记语言。它通常用于数据存储
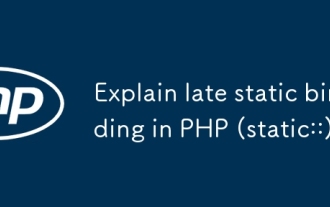
静态绑定(static::)在PHP中实现晚期静态绑定(LSB),允许在静态上下文中引用调用类而非定义类。1)解析过程在运行时进行,2)在继承关系中向上查找调用类,3)可能带来性能开销。

PHP的魔法方法有哪些?PHP的魔法方法包括:1.\_\_construct,用于初始化对象;2.\_\_destruct,用于清理资源;3.\_\_call,处理不存在的方法调用;4.\_\_get,实现动态属性访问;5.\_\_set,实现动态属性设置。这些方法在特定情况下自动调用,提升代码的灵活性和效率。
