在Java中处理反序列化JSON时生成的错误的方法是什么?
当在反序列化过程中遇到可能可恢复的问题时,可以注册DeserializationProblemHandler 类来进行调用。我们可以通过实现DeserializationProblemHandler 类的handleUnknownProperty()方法来处理在反序列化JSON时生成的错误。
语法
public boolean handleUnknownProperty(DeserializationContext ctxt, JsonParser p, JsonDeserializer<!--?--> deserializer, Object beanOrClass, String propertyName) throws IOException
Example
import java.io.*; import com.fasterxml.jackson.core.*; import com.fasterxml.jackson.databind.*; import com.fasterxml.jackson.databind.deser.*; public class DeserializationErrorTest { public static void main(String[] args) throws JsonMappingException, JsonGenerationException, IOException { String jsonString = "{\"id\":\"101\", \"name\":\"Ravi Chandra\", \"address\":\"Pune\", \"salary\":\"40000\" }"; <strong>ObjectMapper </strong>objectMapper = new ObjectMapper(); DeserializationProblemHandler deserializationProblemHandler = new UnMarshallingErrorHandler(); objectMapper.addHandler(deserializationProblemHandler); Customer customer = objectMapper.readValue(jsonString, Customer.class); System.out.println(customer); } } // UnMarshallingErrorHandler class<strong> </strong>class UnMarshallingErrorHandler extends DeserializationProblemHandler { @Override public boolean handleUnknownProperty(DeserializationContext ctxt, JsonParser jp, JsonDeserializer deserializer, Object beanOrClass, String propertyName) throws IOException, JsonProcessingException { boolean result = false; super.handleUnknownProperty(ctxt, jp, deserializer, beanOrClass, propertyName); System.out.println("Property with name '" + propertyName + "' doesn't exist in Class of type '" + beanOrClass.getClass().getName() + "'"); return true; // returns true to inform the deserialization process that we can handle the error and it can continue deserializing and returns false, if we want to stop the deserialization immediately. } } // Customer class class Customer { private int id; private String name; private String address; public int getId() { return id; } public void setId(int id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getAddress() { return address; } public void setAddress(String address) { this.address = address; } @Override public String toString() { return "Customer [id=" + id + ", name=" + name + ", address=" + address + "]"; } }
当返回true时的输出
Property with name 'salary' doesn't exist in Class of type 'Customer' Customer [id=101, name=Ravi Chandra, address=Pune]
当返回false时的输出
Property with name 'salary' doesn't exist in Class of type 'Customer' Exception in thread "main" com.fasterxml.jackson.databind.exc.UnrecognizedPropertyException: Unrecognized field "salary" (class Customer), not marked as ignorable (3 known properties: "id", "address", "name"]) at [Source: (String)"{"id":"101", "name":"Ravi Chandra", "address":"Pune", "salary":"40000" }"; line: 1, column: 65] (through reference chain: Customer["salary"]) at com.fasterxml.jackson.databind.exc.UnrecognizedPropertyException.from(UnrecognizedPropertyException.java:61) at com.fasterxml.jackson.databind.DeserializationContext.handleUnknownProperty(DeserializationContext.java:840) at com.fasterxml.jackson.databind.deser.std.StdDeserializer.handleUnknownProperty(StdDeserializer.java:1179) at com.fasterxml.jackson.databind.deser.BeanDeserializerBase.handleUnknownProperty(BeanDeserializerBase.java:1592) at com.fasterxml.jackson.databind.deser.BeanDeserializerBase.handleUnknownVanilla(BeanDeserializerBase.java:1570) at com.fasterxml.jackson.databind.deser.BeanDeserializer.vanillaDeserialize(BeanDeserializer.java:294) at com.fasterxml.jackson.databind.deser.BeanDeserializer.deserialize(BeanDeserializer.java:151) at com.fasterxml.jackson.databind.ObjectMapper._readMapAndClose(ObjectMapper.java:4202) at com.fasterxml.jackson.databind.ObjectMapper.readValue(ObjectMapper.java:3205) at com.fasterxml.jackson.databind.ObjectMapper.readValue(ObjectMapper.java:3173) at DeserializationErrorTest.main(DeserializationErrorTest.java:12)<strong> </strong>
以上是在Java中处理反序列化JSON时生成的错误的方法是什么?的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
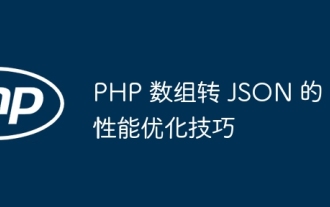
PHP数组转JSON的性能优化方法包括:使用JSON扩展和json_encode()函数;添加JSON_UNESCAPED_UNICODE选项以避免字符转义;使用缓冲区提高循环编码性能;缓存JSON编码结果;考虑使用第三方JSON编码库。
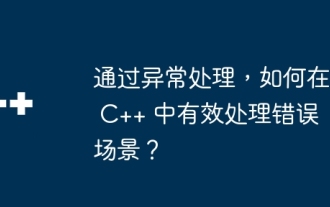
在C++中,异常处理通过try-catch块优雅地处理错误,常见的异常类型包括运行时错误、逻辑错误和超出界限错误。以文件打开错误处理为例,当程序打开文件失败时,它会抛出异常,并通过catch块打印错误消息和返回错误代码,从而在不终止程序的情况下处理错误。异常处理提供错误处理集中化、错误传递和代码健壮性等优势。
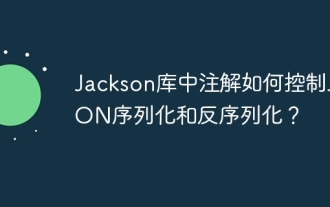
Jackson库中的注解可控制JSON序列化和反序列化:序列化:@JsonIgnore:忽略属性@JsonProperty:指定名称@JsonGetter:使用获取方法@JsonSetter:使用设置方法反序列化:@JsonIgnoreProperties:忽略属性@JsonProperty:指定名称@JsonCreator:使用构造函数@JsonDeserialize:自定义逻辑
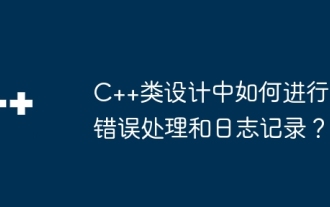
C++类设计中的错误处理和日志记录包括:异常处理:捕获并处理异常,使用自定义异常类提供特定错误信息。错误码:使用整数或枚举表示错误条件,在返回值中返回。断言:验证预置和后置条件,不成立时引发异常。C++库日志:使用std::cerr和std::clog进行基本日志记录。外部日志库:集成第三方库以获得高级功能,如级别过滤和日志文件旋转。自定义日志类:创建自己的日志类,抽象底层机制,提供通用接口记录不同级别信息。
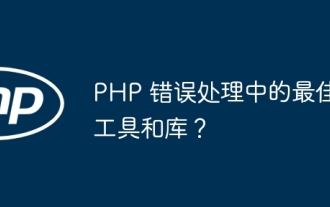
PHP中最佳的错误处理工具和库包括:内置方法:set_error_handler()和error_get_last()第三方工具包:Whoops(调试和错误格式化)第三方服务:Sentry(错误报告和监控)第三方库:PHP-error-handler(自定义错误日志记录和堆栈跟踪)和Monolog(错误日志记录处理器)
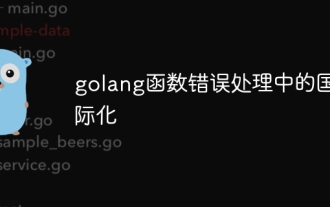
GoLang函数可以通过errors包中的Wrapf和Errorf函数进行错误国际化,从而创建本地化的错误消息,并附加到其他错误中,形成更高级别的错误。通过使用Wrapf函数,可以国际化低级错误,并追加自定义消息,例如"打开文件%s出错"。
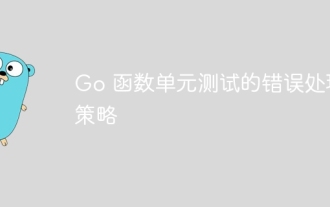
在Go函数单元测试中,错误处理有两种主要策略:1.将错误表示为error类型的具体值,用于断言预期值;2.使用通道向测试函数传递错误,适用于测试并发代码。实战案例中,使用错误值策略确保函数对负数输入返回0。
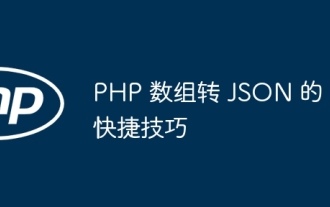
PHP数组可通过json_encode()函数转换为JSON字符串(例如:$json=json_encode($array);),反之亦可用json_decode()函数从JSON转换为数组($array=json_decode($json);)。其他技巧还包括:避免深度转换、指定自定义选项以及使用第三方库。
