Java程序用于检查TPP学生是否有资格参加面试
请考虑下表了解不同公司的资格标准 -
CGPA | 的中文翻译为:绩点平均成绩 |
符合条件的公司 |
---|---|---|
大于或等于8 |
谷歌、微软、亚马逊、戴尔、英特尔、Wipro |
|
大于或等于7 |
教程点、accenture、Infosys、Emicon、Rellins |
|
大于或等于6 |
rtCamp、Cybertech、Skybags、Killer、Raymond |
|
大于或等于5 |
Patronics、鞋子、NoBrokers |
让我们进入 java 程序来检查 tpp 学生参加面试的资格。
方法1:使用if else if条件
通常,当我们必须检查多个条件时,我们会使用 if else if 语句。它遵循自上而下的方法。
语法
if(condition 1) { // code will be executed only when condition 1 is true } else if(condition 2) { // code will be executed only when condition 2 is true } else { // code will be executed when all of the above condition is false }
示例
public class Eligible { public static void main(String[] args) { int regd = 12109659; double CGPA = 8.08; if( CGPA >= 8 ) { System.out.println(regd + " is eligible for companies: Google, Microsoft, Amazon, Capgemini, Dell, Intel, Wipro"); } else if(CGPA >= 7) { System.out.println(regd + " is eligible for companies: Tutorials point, accenture, Infosys, Emicon, Rellins"); } else if(CGPA >= 6) { System.out.println(regd + " is eligible for companies: rtCamp, Cybertech, Skybags, Killer, Raymond"); } else if( CGPA >= 5 ) { System.out.println(regd + " is eligible for companies: Patronics, Bata, Nobroker"); } else { System.out.println("Improve yourself!"); } } }
输出
12109659 is eligible for companies: Google, Microsoft, Amazon, Capgemini, Dell, Intel, Wiproe
在上面的代码中,我们声明并初始化了两个名为“regd”和“CGPA”的变量。当我们运行此代码时,编译器将检查第一个 if 条件,并且对于给定的“CGPA”值,它是 true。因此,它执行了第一个 if 块内的代码。
使用Switch语句的方法
switch 语句仅适用于 int、short、byte 和 char 数据类型。它不支持十进制值。它首先评估表达式,如果有任何情况匹配,它将执行该代码块。如果没有任何情况匹配,则执行默认情况。
语法
// expression and value must be of same datatype switch(expression) { case value: // code will be executed only when the expression and case value matched break; case value: // code will be executed only when the expression and case value matched break; . . . case value n: // n is required number of value default: // If none of the case matched then it will be executed }
示例
public class Main { public static void main(String[] args){ int regd = 12109659; double CGPA = 6.55; int GPA = (int) CGPA; // typecasting double to integer type switch(GPA){ // here GPA = 6 case 10: case 9: case 8: System.out.println(regd + " is eligible for companies: Google, Microsoft, Amazon, Capgemini, Dell, Intel, Wipro"); break; case 7: System.out.println(regd + " is eligible for companies: Tutorials point, accenture, Infosys, Emicon, Rellins"); break; case 6: System.out.println(regd + " is eligible for companies: rtCamp, Cybertech, Skybags, Killer, Raymond"); break; case 5: System.out.println(regd + " is eligible for companies: Patronics, Bata, Nobroker"); break; default: System.out.println("Improve yourself!"); } } }
输出
12109659 is eligible for companies: rtCamp, Cybertech, Skybags, Killer, Raymond
在上面的代码中,我们再次采用了相同的变量。由于 switch 与 double 变量不兼容,我们将其类型转换为名为“GPA”的整数类型变量。对于给定的“GPA”值,情况 6 与表达式匹配。因此,编译器执行了 case 6 代码。
方法三:使用用户自定义方法
方法是可以多次重用以执行单个操作的代码块。它节省了我们的时间,也减少了代码的大小。
语法
accessSpecifier nonAccessModifier return_Type method_Name(Parameters){ //Body of the method }
accessSpecifier - 用于设置方法的可访问性。它可以是公共的、受保护的、默认的和私有的。
nonAccessModifier - 它显示方法的附加功能或行为,例如静态和最终。
return_Type − 方法将返回的数据类型。当方法不返回任何内容时,我们使用void关键字。
method_Name - 方法的名称。
参数 - 它包含变量名称,后跟数据类型。
示例
public class Main { public static void eligible(int regd, double CGPA){ if(CGPA >= 8){ System.out.println(regd + " is eligible for companies: Google, Microsoft, Amazon, Capgemini, Dell, Intel, Wipro"); } else if(CGPA >= 7){ System.out.println(regd + " is eligible for companies: Tutorials point, accenture, Infosys, Emicon, Rellins"); } else if(CGPA >= 6){ System.out.println(regd + " is eligible for companies: rtCamp, Cybertech, Skybags, Killer, Raymond"); } else if(CGPA >= 5){ System.out.println(regd + " is eligible for companies: Patronics, Bata, Nobroker"); } else { System.out.println("Improve yourself!"); } } public static void main(String[] args){ eligible(12109659, 7.89); } }
输出
12109659 is eligible for companies: Tutorials point, accenture, Infosys, Emicon, Rellins
上述程序的逻辑与我们在本文中讨论的第一个程序相同。主要区别在于我们创建了一个名为“eligible()”的用户定义方法,带有两个参数“regd”和“CGPA”,并且我们在主方法中使用两个参数调用了该方法。
结论
在本文中,我们讨论了三种用于检查tpp学生是否有资格参加面试的java程序方法。我们看到了使用if else if条件和switch语句的用法。我们还为给定的问题创建了一个用户定义的方法。
以上是Java程序用于检查TPP学生是否有资格参加面试的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
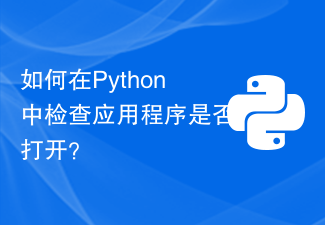
正在执行的程序称为进程。进程可以是当前操作系统上运行的应用程序,也可以是与操作系统相关的应用程序。如果一个应用程序与操作系统相关,它首先会创建一个进程来执行自己。其他应用程序依赖操作系统服务来执行。大多数应用程序是操作系统服务以及维护操作系统、软件和硬件的后台应用程序。在python中,我们有不同的方法来检查应用程序是否打开。让我们一一详细了解它们。使用psutil.process_iter()函数psutil是python中的一个模块,它为用户提供一个接口来检索正在运行的进程和系统利用率的信息
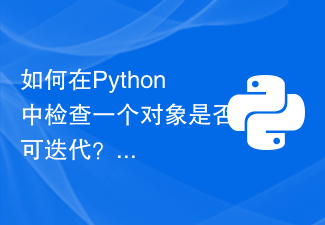
可迭代对象是可以使用循环或可迭代函数迭代其所有元素的对象。列表、字符串、字典、元组等都称为可迭代对象。在Python语言中,有多种方法可以检查对象是否可迭代。让我们一一看看。使用循环在Python中,我们有两种循环技术,一种是使用“for”循环,另一种是使用“while”循环。使用这两个循环中的任何一个,我们可以检查给定的对象是否可迭代。示例在这个例子中,我们将尝试使用“for”循环迭代一个对象并检查它是否被迭代。以下是代码。l=["apple",22,"orang
![拼写检查在团队中不起作用[修复]](https://img.php.cn/upload/article/000/887/227/170968741326618.jpg?x-oss-process=image/resize,m_fill,h_207,w_330)
我们已经开始注意到,有时拼写检查停止工作的团队。拼写检查是有效沟通的基本工具,任何对它的打击都会对工作流程造成相当大的破坏。在本文中,我们将探讨拼写检查可能无法按预期运行的常见原因,以及如何将其恢复到以前的状态。所以,如果拼写检查在团队中不起作用,请遵循本文中提到的解决方案。为什么Microsoft拼写检查不起作用?Microsoft拼写检查无法正常工作可能有多种原因。这些原因包括不兼容的语言设置、拼写检查功能被禁用、MSTeam或MSOffice安装损坏等。另外,过时的MSTeams和MSOf
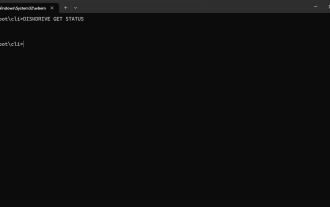
Windows11中如何检查SSD运行状况?对于其快速的读取、写入和访问速度,SSD正在迅速取代HDD,但即使它们更可靠,您仍然需要在Windows11中检查SSD的运行状况。怎么去操作呢?本篇教程小编就来为大家分享一下方法吧。方法一:使用WMIC1、使用按键组合Win+R,键入wmic,然后按或单击“确定”。Enter2、现在,键入或粘贴以下命令以检查SSD运行状况:diskdrivegetstatus如果您收到“状态:正常”消息,则您的SSD驱动器运行正
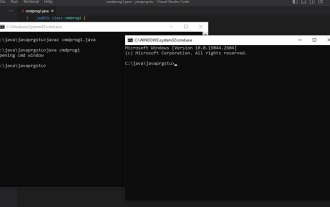
本文使用多种方法通过 Java 代码选择在打开的命令窗口中插入的命令。命令窗口是使用“cmd”打开的。这里,使用 Java 代码指定执行相同操作的方法。首先使用 Java 程序打开命令窗口。
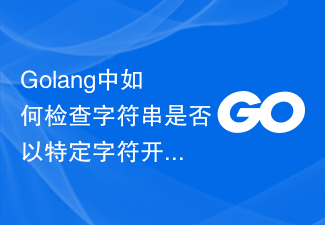
Golang中如何检查字符串是否以特定字符开头?在使用Golang编程时,经常会遇到需要检查一个字符串是否以特定字符开头的情况。针对这一需求,我们可以使用Golang中的strings包提供的函数来实现。接下来将详细介绍如何使用Golang检查字符串是否以特定字符开头,并附上具体的代码示例。在Golang中,我们可以使用strings包中的HasPrefix
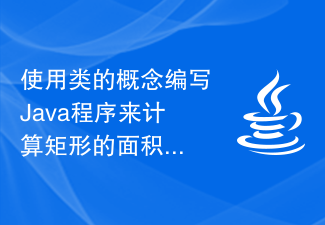
Java语言是当今世界上最常用的面向对象编程语言之一。类的概念是面向对象语言中最重要的特性之一。一个类就像一个对象的蓝图。例如,当我们想要建造一座房子时,我们首先创建一份房子的蓝图,换句话说,我们创建一个显示我们将如何建造房子的计划。根据这个计划,我们可以建造许多房子。同样地,使用类,我们可以创建许多对象。类是创建许多对象的蓝图,其中对象是真实世界的实体,如汽车、自行车、笔等。一个类具有所有对象的特征,而对象具有这些特征的值。在本文中,我们将使用类的概念编写一个Java程序,以找到矩形的周长和面
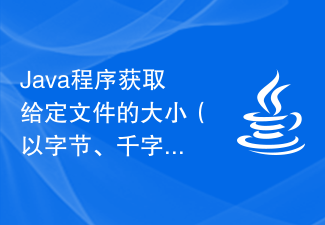
文件的大小是特定文件在特定存储设备(例如硬盘驱动器)上占用的存储空间量。文件的大小以字节为单位来衡量。在本节中,我们将讨论如何实现一个java程序来获取给定文件的大小(以字节、千字节和兆字节为单位)。字节是数字信息的最小单位。一个字节等于八位。1千字节(KB)=1,024字节1兆字节(MB)=1,024KB千兆字节(GB)=1,024MB和1太字节(TB)=1,024GB。文件的大小通常取决于文件的类型及其包含的数据量。以文本文档为例,文件的大小可能只有几千字节,而高分辨率图像或视频文件的大小可
