在Java中查找两个数组元素的最大和
Two elements giving the maximum sum in an array means, we have to find two largest array elements which will eventually give the maximum sum possible.
In this article we will see how we can find the maximum sum of two elements in Java.
To show you some instances
Instance-1
的中文翻译为:实例-1
Suppose we have the below array
[10, 2, 3, -5, 99, 12, 0, -1]
In this array the largest element is 99 and second largest is 12.
Max sum = 99 + 12
因此,这个数组中两个元素的最大和为111。
Instance-2
Suppose we have the below array
[556, 10, 259, 874, 123, 453, -96, -54, -2369]
在这个数组中,最大的元素是874,第二大的元素是556。
Max sum = 874+556
因此,该数组中两个元素的最大和为1430。
Instance-3
Suppose we have the below array
[55, 10, 29, 74, 12, 45, 6, 5, 269]
在这个数组中,最大的元素是269,第二大的元素是74。
Max sum= 269+74
因此,该数组中两个元素的最大和为343。
Algorithm
算法-1
步骤 1 − 使用 for 循环在数组中找到最大和第二大的元素。
步骤 2 - 找到它们的和。
Step 3 − Print the sum.
Algorithm-2
的翻译为:算法-2
Step 1 − Sort the array elements.
Step 2 −Take the last and second last element of the array.
Step 3 − Find their sum.
Step 4 − Print the sum.
Syntax
To sort the array we need to use the sort( ) method of the Arrays class of java.util package.
Following is the syntax to sort any array in ascending order using the method
<span class="typ">Arrays</span><span class="pun">.</span><span class="pln">sort</span><span class="pun">(</span><span class="pln">array_name</span><span class="pun">);</span>
Where, ‘array_name’ refers to the array that you want to sort.
多种方法
We have provided the solution in different approaches.
使用for循环找到最大的和
Find The Largest Sum Using Arrays.sort
让我们逐一查看程序及其输出。
方法一:使用for循环
In this approach, we use a for loop to iterate through the array elements to find out the largest and second largest element. These two elements will give the maximum sum.
Example
public class Main { public static void main(String[] args) { // The array elements int arr[] = { 10, 2, 3, -5, 99, 12, 0, -1 }; // Storing the first element in both variables int first = arr[0], second = arr[0]; // For loop to iterate the elements from 1 to n // to find the first largest element for (int i = 0; i < arr.length; i++) { // If array element is larger than current largest element, then swap if (arr[i] > first) first = arr[i]; } // For loop to iterate the elements from 1 to n // to find the second largest element for (int i = 0; i < arr.length; i++) { // If array element is larger than current largest element and not equals to // largest element, then swap if (arr[i] > second && arr[i] != first) second = arr[i]; } // Print the sum System.out.println("Largest sum = " + (first + second)); System.out.println("The elements are " + first + " and " + second); } }
Output
Largest sum = 111 The elements are 99 and 12
Approach-2: By Using Arrays.sort
在这种方法中,我们使用Arrays.sort()方法对数组进行排序。然后我们取最后一个和倒数第二个索引上的元素。由于数组已经排序过了,这两个元素将给出最大的和。
Example
import java.util.Arrays; public class Main { public static void main(String[] args) { // The array elements int arr[] = { 10, 2, 3, -5, 99, 12, 0, -1 }; // Sort the array using the sort method from array class Arrays.sort(arr); // Storing the last element as largest and second last element as second largest int first = arr[arr.length - 1], second = arr[arr.length - 2]; // Print the maximum sum System.out.println("Maximum sum = " + (first + second)); System.out.println("The elements are " + first + " and " + second); } }
Output
Maximum sum = 104 The elements are 99 and 12
在本文中,我们探讨了在Java中找到数组中具有最大和的两个元素的不同方法。
以上是在Java中查找两个数组元素的最大和的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)

热门话题
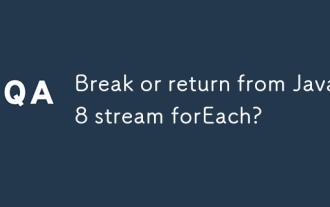
Java 8引入了Stream API,提供了一种强大且表达力丰富的处理数据集合的方式。然而,使用Stream时,一个常见问题是:如何从forEach操作中中断或返回? 传统循环允许提前中断或返回,但Stream的forEach方法并不直接支持这种方式。本文将解释原因,并探讨在Stream处理系统中实现提前终止的替代方法。 延伸阅读: Java Stream API改进 理解Stream forEach forEach方法是一个终端操作,它对Stream中的每个元素执行一个操作。它的设计意图是处
