如何使用Java开发一个基于Spring Cloud Alibaba的微服务架构
如何使用Java开发一个基于Spring Cloud Alibaba的微服务架构
微服务架构已经成为了现代软件开发的主流架构之一,它将一个复杂的系统拆分成多个小型的、独立的服务,每个服务都可以独立部署、扩展和管理。而Spring Cloud Alibaba则是基于Spring Cloud的开源项目,为开发者提供了一套快速构建微服务架构的工具和组件。
本文将介绍如何使用Java开发一个基于Spring Cloud Alibaba的微服务架构,并提供具体的代码示例。我们将使用以下几个组件来构建我们的微服务架构:
- Spring Boot:Spring Boot是一个快速构建应用的框架,它提供了诸如自动配置、通用启动、监控、性能测试等一系列功能。我们将使用Spring Boot作为我们的微服务框架。
- Spring Cloud Alibaba:Spring Cloud Alibaba是阿里巴巴基于Spring Cloud开发的一套微服务框架。它提供了服务注册与发现、配置管理、负载均衡、熔断器等一系列功能。
- Nacos:Nacos是一个用于动态服务发现、配置管理的平台。我们将使用Nacos作为我们的注册中心,用于注册、管理和发现我们的微服务。
- Sentinel:Sentinel是一个高性能的并发流量控制框架,用于保护微服务的稳定性。我们将使用Sentinel作为我们的熔断器,以防止服务出现长时间不可用的情况。
- Feign:Feign是一个声明式的HTTP客户端,它可以帮助我们快速构建和调用其他微服务的接口。
下面是具体的代码示例,以展示如何使用Java开发一个基于Spring Cloud Alibaba的微服务架构:
首先,我们需要创建一个Spring Boot项目,并添加相关的依赖。在项目的pom.xml文件中添加以下依赖:
<!-- Spring Boot --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter</artifactId> </dependency> <!-- Spring Cloud Alibaba --> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-alibaba-nacos-discovery</artifactId> </dependency> <!-- Spring Cloud Alibaba Sentinel --> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-alibaba-sentinel</artifactId> </dependency> <!-- Feign --> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-feign</artifactId> </dependency>
接下来,我们需要在启动类上添加相关的注解,以启用Spring Cloud Alibaba的功能:
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.cloud.client.discovery.EnableDiscoveryClient; import org.springframework.cloud.openfeign.EnableFeignClients; @SpringBootApplication @EnableDiscoveryClient @EnableFeignClients public class MicroserviceApplication { public static void main(String[] args) { SpringApplication.run(MicroserviceApplication.class, args); } }
然后,我们需要创建一个微服务,并使用RestController注解来标识该服务是一个RESTful服务:
import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; @RestController @RequestMapping("/api") public class HelloController { @GetMapping("/hello") public String hello() { return "Hello World!"; } }
接下来,我们需要创建一个Feign客户端来调用其他微服务的接口。在接口上使用FeignClient注解,并指定要调用的微服务名称和接口路径:
import org.springframework.cloud.openfeign.FeignClient; import org.springframework.web.bind.annotation.GetMapping; @FeignClient(name = "other-service") public interface OtherServiceClient { @GetMapping("/api/hello") String hello(); }
最后,我们可以在我们的微服务中调用其他微服务的接口。在我们的控制器中注入Feign客户端,并调用其接口方法:
import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; @RestController @RequestMapping("/api") public class HelloController { private final OtherServiceClient otherServiceClient; @Autowired public HelloController(OtherServiceClient otherServiceClient) { this.otherServiceClient = otherServiceClient; } @GetMapping("/hello") public String hello() { String response = otherServiceClient.hello(); return "Hello World! " + response; } }
以上就是使用Java开发一个基于Spring Cloud Alibaba的微服务架构的具体代码示例。通过使用Spring Boot、Spring Cloud Alibaba等组件,我们可以方便地构建和管理一个复杂的微服务架构,并实现高性能、高可用的服务。希望本文对您有所帮助!
以上是如何使用Java开发一个基于Spring Cloud Alibaba的微服务架构的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)

热门话题
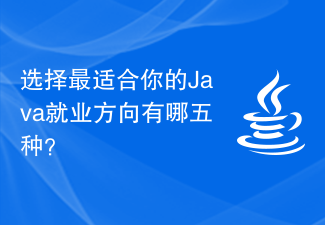
从事Java行业的五个就业方向,你适合哪一个?Java作为一种广泛应用于软件开发领域的编程语言,一直以来都备受青睐。由于其强大的跨平台性和丰富的开发框架,Java开发人员在各行各业中都有着广泛的就业机会。在Java行业中,有五个主要的就业方向,包括JavaWeb开发、移动应用开发、大数据开发、嵌入式开发和云计算开发。每个方向都有其特点和优势,下面将对这五个方
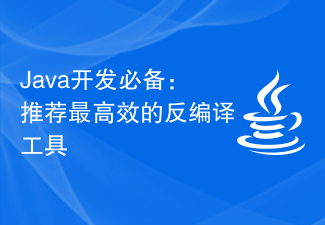
Java开发者必备:推荐最好用的反编译工具,需要具体代码示例引言:在Java开发过程中,我们经常会遇到需要对已有的Java类进行反编译的情况。反编译可以帮助我们了解和学习别人的代码,或者进行修复和优化。本文将推荐几款最好用的Java反编译工具,以及提供一些具体的代码示例,以帮助读者更好地学习和使用这些工具。一、JD-GUIJD-GUI是一款非常受欢迎的开源
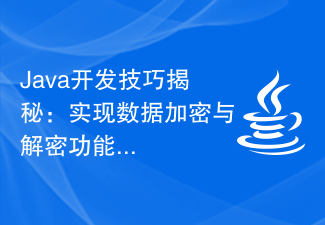
Java开发技巧揭秘:实现数据加密与解密功能在当前信息化时代,数据安全成为一个非常重要的问题。为了保护敏感数据的安全性,很多应用程序都会使用加密算法来对数据进行加密。而Java作为一种非常流行的编程语言,也提供了丰富的加密技术和工具库。本文将揭秘一些Java开发中实现数据加密和解密功能的技巧,帮助开发者更好地保护数据安全。一、数据加密算法的选择Java支持多
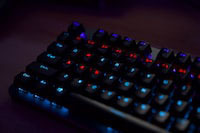
PHP微服务架构已成为构建复杂应用程序和实现高伸缩性和可用性的流行方式。但是,采用微服务也带来了独特的挑战和机遇。本文将深入探究php微服务架构的这些方面,帮助开发人员在探索未知领域时做出明智的决策。挑战分布式系统复杂性:微服务架构将应用程序分解为松散耦合的服务,这增加了分布式系统固有的复杂性。例如,服务之间通信、故障处理和网络延迟都成为需要考虑的因素。服务治理:管理大量微服务需要一种机制来发现、注册、路由和管理这些服务。这涉及到构建和维护一个服务治理框架,这可能会很耗费资源。故障处理:在微服务
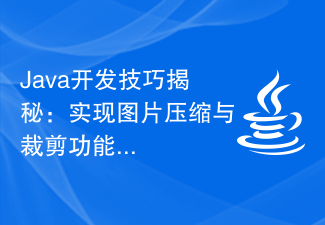
Java作为一种广泛应用于软件开发领域的编程语言,其丰富的库和强大的功能可用于开发各种应用程序。在Web和移动应用开发中,图片压缩和裁剪是常见的需求。在本文中,将揭秘一些Java开发技巧,帮助开发者实现图片压缩和裁剪的功能。首先,让我们讨论图片压缩的实现。在Web应用中,经常需要通过网络传输图片。如果图片过大,将会导致加载时间过长和占用更多的带宽。因此,我们

Java作为一种非常流行的编程语言,一直备受大家的青睐。在我刚开始学习Java开发的过程中,曾经碰到过一个问题——如何构建一个消息订阅系统。在这篇文章中,我将分享我从零开始构建消息订阅系统的经验,希望对其他Java初学者有所帮助。第一步:选择合适的消息队列要构建一个消息订阅系统,首先需要选择一个合适的消息队列。目前市面上比较流行的消息队列有ActiveMQ、
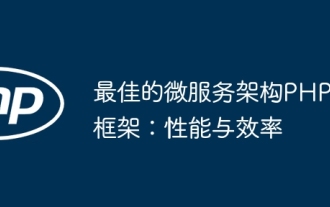
最佳PHP微服务框架:Symfony:灵活性、性能和可扩展性,提供组件套件用于构建微服务。Laravel:专注效率和可测试性,提供干净的API接口,支持无状态服务。Slim:极简主义,速度快,提供简单的路由系统和可选的中体建器,适用于构建高性能API。

深入理解Java开发中的文件压缩与解压缩技术随着互联网的高速发展和信息技术的日新月异,大量的数据交换和传输已经成为当今社会的常态。为了高效地存储和传输数据,文件压缩与解压缩技术应运而生。在Java开发中,文件压缩与解压缩是一个必备的技能,本文将深入探讨这一技术的原理和使用方法。一、文件压缩与解压缩的原理在计算机中,文件压缩就是将一个或多个文件通过使用特定的算
