C++中数据结构问题和解决方案的讨论
C++中数据结构问题和解决方案的讨论
数据结构是计算机科学中非常重要的概念之一,它是存储和组织数据的方式和方法。在C++编程中,我们经常会遇到各种各样的数据结构问题,比如如何高效地存储和操作数据,如何实现各种常见数据结构等等。本文将探讨C++中一些常见的数据结构问题,并提供解决方案的示例代码。
- 数组与动态数组
在C++中,数组是最简单的数据结构之一。它可以一次性存储多个具有相同数据类型的元素。然而,数组的大小在编译时必须确定,无法动态地进行调整。为了解决这个问题,我们可以使用动态数组,即动态分配内存来实现数组的灵活性。
#include <iostream> using namespace std; int main() { int size; cout << "请输入数组的大小:"; cin >> size; int *arr = new int[size]; // 动态分配内存 for (int i = 0; i < size; i++) { cout << "请输入第 " << i + 1 << " 个元素:"; cin >> arr[i]; } // 对数组进行操作... delete[] arr; // 释放内存 return 0; }
- 链表
链表是另一种常见的数据结构,与数组相比,它具有动态性,可以在运行时进行插入、删除等操作。在C++中,我们可以使用指针来实现链表。
#include <iostream> using namespace std; struct Node { int data; Node *next; }; int main() { Node *head = NULL; Node *current = NULL; int size; cout << "请输入链表的长度:"; cin >> size; for (int i = 0; i < size; i++) { int val; cout << "请输入第 " << i + 1 << " 个节点的值:"; cin >> val; Node *newNode = new Node; newNode->data = val; newNode->next = NULL; if (head == NULL) { head = newNode; current = head; } else { current->next = newNode; current = current->next; } } // 遍历链表并打印每个节点的值 Node *temp = head; while (temp != NULL) { cout << temp->data << " "; temp = temp->next; } // 对链表进行操作... // 释放内存 temp = head; while (temp != NULL) { Node *delNode = temp; temp = temp->next; delete delNode; } return 0; }
- 栈和队列
栈和队列是两种经常用到的数据结构。栈具有先进后出(LIFO)的特点,队列具有先进先出(FIFO)的特点。
#include <iostream> #include <stack> #include <queue> using namespace std; int main() { // 使用栈 stack<int> myStack; myStack.push(1); myStack.push(2); myStack.push(3); while (!myStack.empty()) { cout << myStack.top() << " "; myStack.pop(); } cout << endl; // 使用队列 queue<int> myQueue; myQueue.push(1); myQueue.push(2); myQueue.push(3); while (!myQueue.empty()) { cout << myQueue.front() << " "; myQueue.pop(); } cout << endl; return 0; }
- 哈希表
哈希表是一种高效的数据结构,它以键值对的形式存储数据。在C++中,我们可以使用std::unordered_map
来实现哈希表。
#include <iostream> #include <unordered_map> using namespace std; int main() { unordered_map<string, int> myMap; myMap["Alice"] = 24; myMap["Bob"] = 30; myMap["Charlie"] = 18; cout << "Bob 的年龄是:" << myMap["Bob"] << endl; return 0; }
在C++编程中,掌握数据结构的实现和应用非常重要。本文基于C++语言,讨论了一些常见的数据结构问题,并提供了相应的解决方案和示例代码。希望读者能够通过本文的学习和实践,更加熟练地运用和理解数据结构在C++编程中的应用。
以上是C++中数据结构问题和解决方案的讨论的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
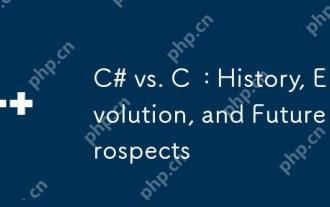
C#和C 的历史与演变各有特色,未来前景也不同。1.C 由BjarneStroustrup在1983年发明,旨在将面向对象编程引入C语言,其演变历程包括多次标准化,如C 11引入auto关键字和lambda表达式,C 20引入概念和协程,未来将专注于性能和系统级编程。2.C#由微软在2000年发布,结合C 和Java的优点,其演变注重简洁性和生产力,如C#2.0引入泛型,C#5.0引入异步编程,未来将专注于开发者的生产力和云计算。
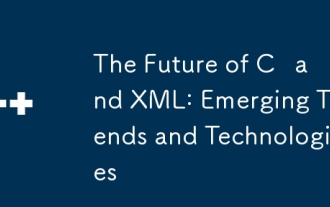
C 和XML的未来发展趋势分别为:1)C 将通过C 20和C 23标准引入模块、概念和协程等新特性,提升编程效率和安全性;2)XML将继续在数据交换和配置文件中占据重要地位,但会面临JSON和YAML的挑战,并朝着更简洁和易解析的方向发展,如XMLSchema1.1和XPath3.1的改进。
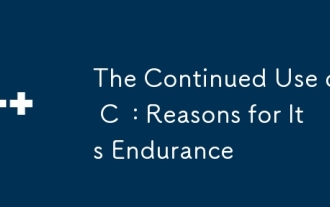
C 持续使用的理由包括其高性能、广泛应用和不断演进的特性。1)高效性能:通过直接操作内存和硬件,C 在系统编程和高性能计算中表现出色。2)广泛应用:在游戏开发、嵌入式系统等领域大放异彩。3)不断演进:自1983年发布以来,C 持续增加新特性,保持其竞争力。
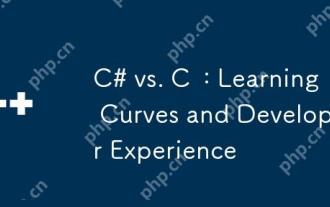
C#和C 的学习曲线和开发者体验有显着差异。 1)C#的学习曲线较平缓,适合快速开发和企业级应用。 2)C 的学习曲线较陡峭,适用于高性能和低级控制的场景。
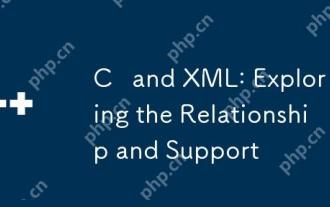
C 通过第三方库(如TinyXML、Pugixml、Xerces-C )与XML交互。1)使用库解析XML文件,将其转换为C 可处理的数据结构。2)生成XML时,将C 数据结构转换为XML格式。3)在实际应用中,XML常用于配置文件和数据交换,提升开发效率。
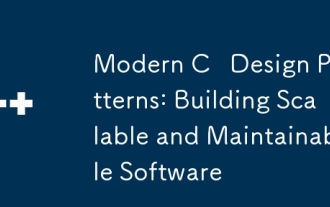
现代C 设计模式利用C 11及以后的新特性实现,帮助构建更灵活、高效的软件。1)使用lambda表达式和std::function简化观察者模式。2)通过移动语义和完美转发优化性能。3)智能指针确保类型安全和资源管理。
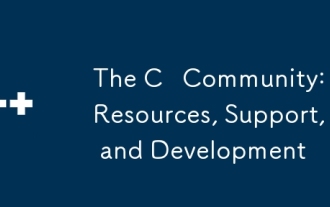
C 学习者和开发者可以从StackOverflow、Reddit的r/cpp社区、Coursera和edX的课程、GitHub上的开源项目、专业咨询服务以及CppCon等会议中获得资源和支持。1.StackOverflow提供技术问题的解答;2.Reddit的r/cpp社区分享最新资讯;3.Coursera和edX提供正式的C 课程;4.GitHub上的开源项目如LLVM和Boost提升技能;5.专业咨询服务如JetBrains和Perforce提供技术支持;6.CppCon等会议有助于职业
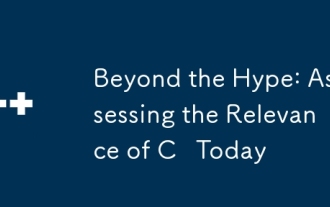
C 在现代编程中仍然具有重要相关性。1)高性能和硬件直接操作能力使其在游戏开发、嵌入式系统和高性能计算等领域占据首选地位。2)丰富的编程范式和现代特性如智能指针和模板编程增强了其灵活性和效率,尽管学习曲线陡峭,但其强大功能使其在今天的编程生态中依然重要。
