深入研究Java线程的几种状态及其对程序执行的影响
深入研究Java线程的几种状态及其对程序执行的影响
在Java中,线程是一种轻量级的执行单位,可以在程序中独立运行并执行特定的任务。线程的状态是描述线程在执行过程中的不同阶段,了解线程的状态对于编写多线程程序以及优化程序性能非常重要。本文将深入研究Java线程的几种状态以及它们对程序执行的影响,并提供具体的代码示例。
Java线程的几种状态包括:NEW(新建)、RUNNABLE(可运行)、BLOCKED(阻塞)、WAITING(等待)、TIMED_WAITING(定时等待)和TERMINATED(终止)。
- 新建(NEW)状态:当通过创建Thread类的实例来新建一个线程时,线程处于新建状态。在新建状态中,线程尚未开始运行任何代码。下面是一个创建新线程的示例代码:
Thread thread = new Thread(() -> { System.out.println("Hello, World!"); });
- 可运行(RUNNABLE)状态:当线程调用start()方法后,线程将进入可运行状态。在这个状态下,线程将等待CPU分配时间片,以便执行run()方法中的代码。如果有多个线程处于可运行状态,操作系统会根据调度策略为这些线程分配时间片。下面是一个示例代码:
Thread thread = new Thread(() -> { System.out.println("Hello, World!"); }); thread.start();
- 阻塞(BLOCKED)状态:当线程因为某些原因不能继续执行时,线程将进入阻塞状态。常见的原因包括等待锁、等待输入输出(I/O)等。在阻塞状态中,线程暂停执行,直到满足某个条件后才能继续执行。下面是一个使用synchronized关键字来实现线程同步的示例代码:
public class MyRunnable implements Runnable { private Object lock = new Object(); public void run() { synchronized(lock) { System.out.println("In synchronized block"); // 一些代码 } } public static void main(String[] args) { MyRunnable runnable = new MyRunnable(); Thread thread1 = new Thread(runnable); Thread thread2 = new Thread(runnable); thread1.start(); thread2.start(); } }
在上述代码中,两个线程尝试同时进入synchronized块,因为锁是共享的,所以第二个线程将进入阻塞状态,直到第一个线程执行完毕释放锁。
- 等待(WAITING)状态:线程在以下情况下会进入等待状态:调用了wait()方法、调用了join()方法或者调用了LockSupport的park()方法。在等待状态中,线程不会主动执行任何代码,直到其他线程唤醒它或者等待时间到达。下面是一个使用wait()方法的示例代码:
public class MyThread extends Thread { public void run() { synchronized(this) { System.out.println("Waiting for next thread..."); try { wait(); } catch (InterruptedException e) { e.printStackTrace(); } System.out.println("Thread resumed."); } } public static void main(String[] args) { MyThread thread = new MyThread(); thread.start(); try { Thread.sleep(1000); } catch (InterruptedException e) { e.printStackTrace(); } synchronized(thread) { thread.notify(); } } }
在上述代码中,线程进入等待状态后,主线程通过notify()方法唤醒了该线程。
- 定时等待(TIMED_WAITING)状态:线程在以下情况下会进入定时等待状态:调用了sleep()方法、调用了带有超时参数的join()方法、调用了带有超时参数的wait()方法、调用了LockSupport的parkNanos()方法或者parkUntil()方法。在定时等待状态中,线程不会主动执行任何代码,等待时间到达后将被唤醒。下面是一个使用sleep()方法的示例代码:
public class MyThread extends Thread { public void run() { try { System.out.println("Thread sleeping..."); Thread.sleep(2000); System.out.println("Thread woke up."); } catch (InterruptedException e) { e.printStackTrace(); } } public static void main(String[] args) { MyThread thread = new MyThread(); thread.start(); } }
在上述代码中,线程通过调用sleep()方法进入定时等待状态,并等待2秒后被唤醒。
- 终止(TERMINATED)状态:线程执行完run()方法中的代码后,线程将进入终止状态。在终止状态中,线程将不再运行。
综上所述,线程的状态对于程序的执行有着重要的影响。了解各种状态以及其含义对于编写高效的多线程程序至关重要。
参考资料:
- Oracle官方文档 - Java线程状态:https://docs.oracle.com/javase/8/docs/api/java/lang/Thread.State.html
- Java多线程入门教程:https://www.journaldev.com/1162/java-thread-tutorial
以上是深入研究Java线程的几种状态及其对程序执行的影响的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

Video Face Swap
使用我们完全免费的人工智能换脸工具轻松在任何视频中换脸!

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)
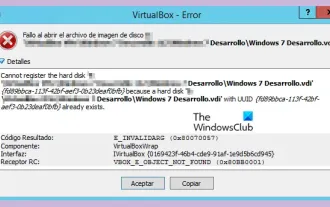
在VirtualBox中尝试打开磁盘映像时,可能会遇到错误提示,指示硬盘无法注册。这种情况通常发生在您尝试打开的VM磁盘映像文件与另一个虚拟磁盘映像文件具有相同的UUID时。在这种情况下,VirtualBox会显示错误代码VBOX_E_OBJECT_NOT_FOUND(0x80bb0001)。如果您遇到这个错误,不必担心,有一些解决方法可以尝试。首先,您可以尝试使用VirtualBox的命令行工具来更改磁盘映像文件的UUID,这样可以避免冲突。您可以运行命令`VBoxManageinternal
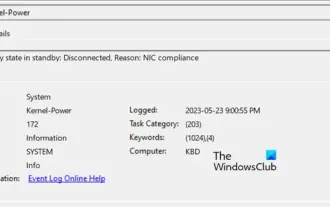
“事件日志消息中的连接状态显示为待机状态:已断开连接,原因是符合NIC标准。这意味着系统在待机模式下,网络接口卡(NIC)已断开连接。虽然这通常是网络问题,但也可能由软件和硬件冲突引起。在接下来的讨论中,我们将探讨如何解决这一问题。”待机连接断开的原因是什么?NIC合规性?如果在Windows事件查看器中发现“ConnectivityStatusinStandby:DisConnected,Reason:NICCompliance”消息,这表示您的NIC或网络接口控制器可能存在问题。这种情况通常
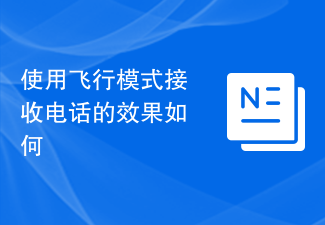
飞行模式别人打电话会怎么样手机已经成为人们生活中必不可少的工具之一,它不仅仅是通信工具,还是娱乐、学习、工作等多种功能的集合体。随着手机功能的不断升级和改进,人们对于手机的依赖性也越来越高。在飞行模式出现后,人们可以更方便地在飞行中使用手机。但是,有人担心在飞行模式下别人打电话的情况会对手机或者使用者产生什么样的影响呢?本文将从几个方面来进行分析和讨论。首先
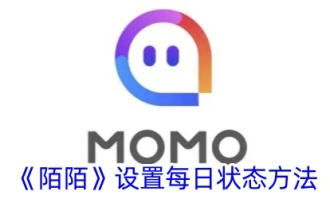
陌陌这款广为人知的社交平台,为用户的日常社交提供了丰富的功能服务。在陌陌上,用户可以轻松分享生活状态、结交朋友、进行聊天等。其中设置状态功能让用户能够向其他展示自己当前的心情和状态,进而吸引更多人的关注和交流。那么究竟该如何设置自己的陌陌状态呢,下文中就为大家带来详细的内容介绍!陌陌怎么设置状态?1、打开陌陌,点击右下角更多,找到并点击每日状态。2、选择状态。3、即可显示设置的状态。
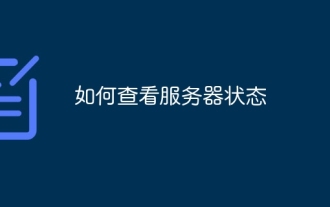
查看服务器状态的方法有使用命令行工具、图形界面工具、监控工具、日志文件和远程管理工具等。详细介绍:1、使用命令行工具,在Linux或Unix服务器上,可以使用命令行工具来查看服务器的状态;2、使用图形界面工具,对于具有图形界面的服务器操作系统,可以使用系统提供的图形界面工具来查看服务器状态;3、使用监控工具,可以使用专门的监控工具来实时监视服务器的状态等等。
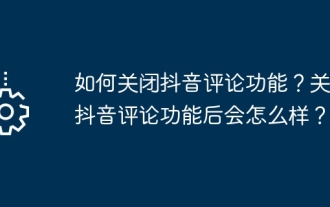
在抖音平台上,用户不仅可以分享自己的生活点滴,还可以与其他用户互动交流。有时候评论功能可能会引发一些不愉快的经历,如网络暴力、恶意评论等。那么,如何关闭抖音评论功能呢?一、如何关闭抖音评论功能?1.登录抖音APP,进入个人主页。2.点击右下角的“我”,进入设置菜单。3.在设置菜单中,找到“隐私设置”。4.点击“隐私设置”,进入隐私设置界面。5.在隐私设置界面,找到“评论设置”。6.点击“评论设置”,进入评论设置界面。7.在评论设置界面,找到“关闭评论”选项。8.点击“关闭评论”选项,确认关闭评论
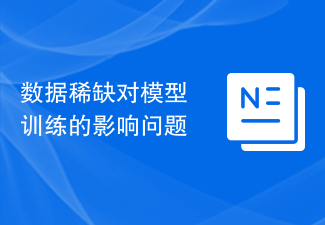
数据稀缺对模型训练的影响问题,需要具体代码示例在机器学习和人工智能领域,数据是训练模型的核心要素之一。然而,现实中我们经常面临的一个问题是数据稀缺。数据稀缺指的是训练数据的量不足或标注数据的缺乏,这种情况下会对模型训练产生一定的影响。数据稀缺的问题主要体现在以下几个方面:过拟合:当训练数据量不够时,模型很容易出现过拟合的现象。过拟合是指模型过度适应训练数据,
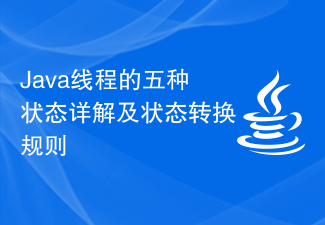
深入了解Java线程的五种状态及其转换规则一、线程的五种状态介绍在Java中,线程的生命周期可以分为五个不同的状态,包括新建状态(NEW)、就绪状态(RUNNABLE)、运行状态(RUNNING)、阻塞状态(BLOCKED)和终止状态(TERMINATED)。新建状态(NEW):当线程对象创建后,它就处于新建状态。此时,线程对象已经分配了足够的资源来执行任务
