在 PHP 中使用 Swift Mailer 发送电子邮件,需要安装 Swift Mailer、配置 SMTP 服务器、创建邮件消息、创建邮件发送器,最后发送邮件。具体步骤包括:安装 Swift Mailer;配置 SMTP 服务器;创建邮件消息;创建邮件发送器;发送邮件。
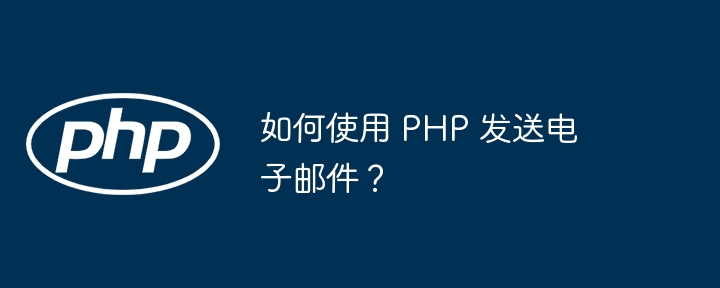
如何在 PHP 中使用 Swift Mailer 发送电子邮件
在 PHP 中发送电子邮件是一种常见的任务,可以通过使用 Swift Mailer 库轻松实现。Swift Mailer 是一个流行的 PHP 库,提供了简单易用的接口来发送电子邮件。
步骤 1:安装 Swift Mailer
1 | composer require swiftmailer/swiftmailer
|
登录后复制
步骤 2:配置 SMTP 服务器
Swift Mailer 需要一个 SMTP 服务器来发送电子邮件。以下是如何使用 Gmail SMTP 服务器进行配置:
1 2 3 | $transport = ( new \Swift_SmtpTransport( 'smtp.gmail.com' , 587))
->setUsername( 'your_gmail_address@gmail.com' )
->setPassword( 'your_gmail_password' );
|
登录后复制
步骤 3:创建邮件消息
1 2 3 4 5 | $message = ( new \Swift_Message())
->setFrom([ 'from_address@example.com' => 'From Name' ])
->setTo([ 'to_address@example.com' => 'To Name' ])
->setSubject( 'Email Subject' )
->setBody( 'Email Body' );
|
登录后复制
步骤 4:创建邮件发送器
1 | $mailer = new \Swift_Mailer( $transport );
|
登录后复制
步骤 5:发送邮件
1 | $result = $mailer ->send( $message );
|
登录后复制
实战案例:发送一封简单的电子邮件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 | use Swift_Mailer;
use Swift_Message;
use Swift_SmtpTransport;
$transport = ( new Swift_SmtpTransport( 'smtp.mailtrap.io' , 2525))
->setUsername( 'your_mailtrap_username' )
->setPassword( 'your_mailtrap_password' );
$message = ( new Swift_Message())
->setFrom([ 'from@example.com' => 'From Name' ])
->setTo([ 'to@example.com' => 'To Name' ])
->setSubject( 'Hello from PHP!' )
->setBody( 'This is a simple email sent using PHP and Swift Mailer.' );
$mailer = new Swift_Mailer( $transport );
$result = $mailer ->send( $message );
if ( $result ) {
echo 'Email sent successfully.' ;
} else {
echo 'There was an error sending the email.' ;
}
|
登录后复制
以上是如何使用 PHP 发送电子邮件?的详细内容。更多信息请关注PHP中文网其他相关文章!