Java函数中递归调用有哪些替代方案?
用迭代替代 Java 函数中的递归调用
在 Java 中,递归是一个强大的工具,用于解决各种问题。但是,在某些情况下,使用迭代可能是一个更好的选择,因为它更有效且不易出现堆栈溢出。
以下是迭代的优点:
- 效率更高,因为它不需要为每个递归调用创建新的栈帧。
- 不容易发生堆栈溢出,因为堆栈空间使用受限制。
替代递归调用的迭代方法:
Java 中有几种方法可以将递归函数转换为迭代函数。
1. 使用栈
使用栈是将递归函数转换为迭代函数最简单的方法。栈是一种后入先出 (LIFO) 数据结构,类似于函数调用栈。
public int factorial(int n) { Stack<Integer> stack = new Stack<>(); stack.push(n); while (!stack.isEmpty()) { int curr = stack.pop(); if (curr == 1) { return 1; } stack.push(curr - 1); stack.push(curr); } }
2. 使用队列
也可以使用队列将递归函数转换为迭代函数。队列是一种先进先出 (FIFO) 数据结构,类似于消息队列。
public int factorial(int n) { Queue<Integer> queue = new LinkedList<>(); queue.offer(n); while (!queue.isEmpty()) { int curr = queue.poll(); if (curr == 1) { return 1; } queue.offer(curr - 1); queue.offer(curr); } }
3. 手动模拟函数调用栈
也可以手动模拟函数调用栈来实现迭代。这涉及显式维护一个栈帧数组,并通过数组索引跟踪当前栈帧。
public int factorial(int n) { int[] stack = new int[100]; int top = -1; stack[++top] = 1; stack[++top] = n; while (top > 0) { int curr = stack[top--]; if (curr == 1) { return stack[top--]; } stack[++top] = curr - 1; stack[++top] = curr; } }
实战案例:斐波那契数列
让我们以斐波那契数列为例,说明如何使用迭代替代递归。
// 递归 public int fib(int n) { if (n <= 1) { return n; } return fib(n - 1) + fib(n - 2); } // 迭代(使用队列) public int fib(int n) { Queue<Integer> queue = new LinkedList<>(); queue.offer(0); queue.offer(1); while (n-- > 1) { int a = queue.poll(); int b = queue.poll(); queue.offer(a + b); } return queue.poll(); }
通过使用迭代,我们避免了递归调用的开销,提高了效率。
以上是Java函数中递归调用有哪些替代方案?的详细内容。更多信息请关注PHP中文网其他相关文章!

热AI工具

Undresser.AI Undress
人工智能驱动的应用程序,用于创建逼真的裸体照片

AI Clothes Remover
用于从照片中去除衣服的在线人工智能工具。

Undress AI Tool
免费脱衣服图片

Clothoff.io
AI脱衣机

AI Hentai Generator
免费生成ai无尽的。

热门文章

热工具

记事本++7.3.1
好用且免费的代码编辑器

SublimeText3汉化版
中文版,非常好用

禅工作室 13.0.1
功能强大的PHP集成开发环境

Dreamweaver CS6
视觉化网页开发工具

SublimeText3 Mac版
神级代码编辑软件(SublimeText3)

热门话题

C++函数的递归深度受到限制,超过该限制会导致栈溢出错误。限制值因系统和编译器而异,通常在1000到10000之间。解决方法包括:1.尾递归优化;2.尾调用;3.迭代实现。
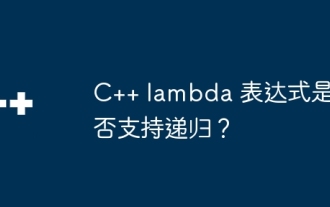
是的,C++Lambda表达式可以通过使用std::function支持递归:使用std::function捕获Lambda表达式的引用。通过捕获的引用,Lambda表达式可以递归调用自身。

递归算法通过函数自调用解决结构化的问题,优点是简洁易懂,缺点是效率较低且可能发生堆栈溢出;非递归算法通过显式管理堆栈数据结构避免递归,优点是效率更高且避免堆栈溢出,缺点是代码可能更复杂。选择递归或非递归取决于问题和实现的具体限制。
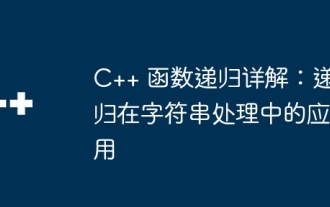
递归函数是一种在字符串处理中反复调用自身来解决问题的技术。它需要一个终止条件以防止无限递归。递归在字符串反转和回文检查等操作中被广泛使用。

Java和Haskell函数的主要区别在于:语法:Java使用return关键字返回结果,而Haskell使用赋值符号(=)。执行模型:Java采用顺序执行,而Haskell采用懒惰求值。类型系统:Java具有静态类型系统,而Haskell具有强大的灵活类型系统,可在编译时和运行时检查类型。实战性能:Haskell在处理大输入时比Java更有效,因为它使用尾递归,而Java使用递归。
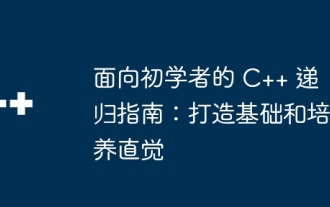
递归是一种强大的技术,它允许函数调用自身来解决问题,在C++中,递归函数由两个关键要素构成:基本情况(确定递归何时停止)和递归调用(将问题分解为更小子问题)。通过理解基础知识并练习实战示例(如阶乘计算、斐波那契数列和二叉树遍历),您可以建立递归直觉,并自信地在代码中使用它。
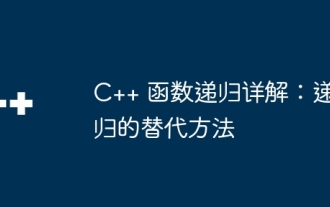
递归是一种函数调用自身的技术,但存在堆栈溢出和效率低下的缺点。替代方法包括:尾递归优化,由编译器优化递归调用为循环;迭代,使用循环而不是递归;协程,允许暂停和恢复执行,模拟递归行为。
