<?php class emp { var $name; var $address; var $dept; function assign_info($n,$a,$d) { $this->name=$n; $this->state=$a; $this->dept=$d; } function display_info() { echo("<p>Employee Name : $this->name"); echo("<p>State : $this->state"); echo("<p>Department : $this->dept"); } } $empobj = new emp; $empobj->assign_info("kaka lname","California","Accounts"); echo("<center><h2>Displaying Employee Information</h2></center>"); echo("<font size=4>"); $empobj->display_info(); echo("</font>"); ?>
This is a php class to define and use. Friends who need it can download it and use it.
All resources on this site are contributed by netizens or reprinted by major download sites. Please check the integrity of the software yourself! All resources on this site are for learning reference only. Please do not use them for commercial purposes. Otherwise, you will be responsible for all consequences! If there is any infringement, please contact us to delete it. Contact information: admin@php.cn
Related Article
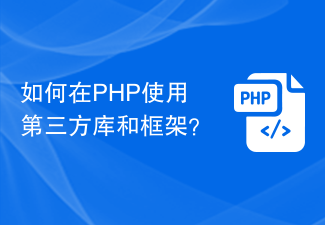
29 Jun 2023
How to use third-party libraries and frameworks in PHP? With the development of the Internet, developers often use third-party libraries and frameworks when writing PHP code. These libraries and frameworks can help developers improve code scalability and maintainability and save development time. This article will introduce how to use third-party libraries and frameworks in PHP. First, what are third-party libraries and frameworks? Third-party libraries are collections of reusable codes developed by other people or organizations that provide some common functions, such as database operations, file uploads, email sending, etc. The framework is
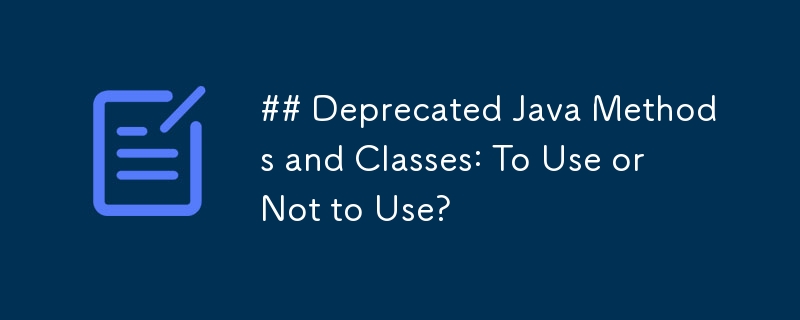
01 Nov 2024
Deprecated Java Methods and Classes: Usage GuidelinesDeveloping web applications in Eclipse often involves updating libraries and encountering...

05 May 2023
1. Comparison of objects Before comparing two objects, we first need to determine what to compare based on. There are so many member variables in the object, and direct comparison is impossible. 1.1Comparable is a parameter of the interface, and fills in the object to be compared. There is only one compareTo abstract method in this interface. The structure is as follows: After implementing this interface in a class, you can compare the sizes between classes. 1.2 Comparator This interface has an abstract method compare, which is also used to implement objects. To compare sizes, the structure of the method is as follows: Different from the Comparable interface, the Comparator interface can be used as the sort method in the Arrays class.
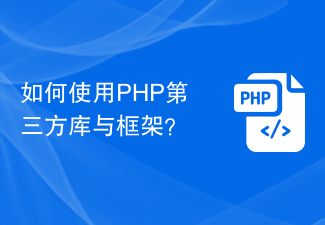
30 Jun 2023
As a popular server-side scripting language, PHP has a wide range of application scenarios and a powerful ecosystem. When we write PHP code, we often encounter situations where we need to use third-party libraries and frameworks to speed up development and improve efficiency. This article will introduce how PHP uses third-party libraries and frameworks. First, we need to clarify what third-party libraries and frameworks are. A third-party library is a collection of PHP code written and maintained by other developers to implement some common functions or solve specific problems. The framework is a higher level of abstraction,
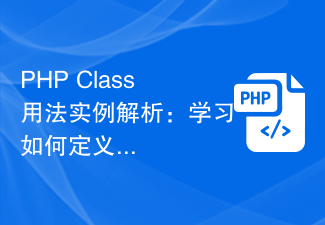
11 Mar 2024
In PHP programming, class is a very important concept. It can help us better organize and manage code, and improve code reusability and maintainability. This article will explain how to define and use PHP classes through example analysis, and provide specific code examples to help readers better understand. 1. Class definition In PHP, use the keyword class to define a class. The sample code is as follows: classPerson{public$name;
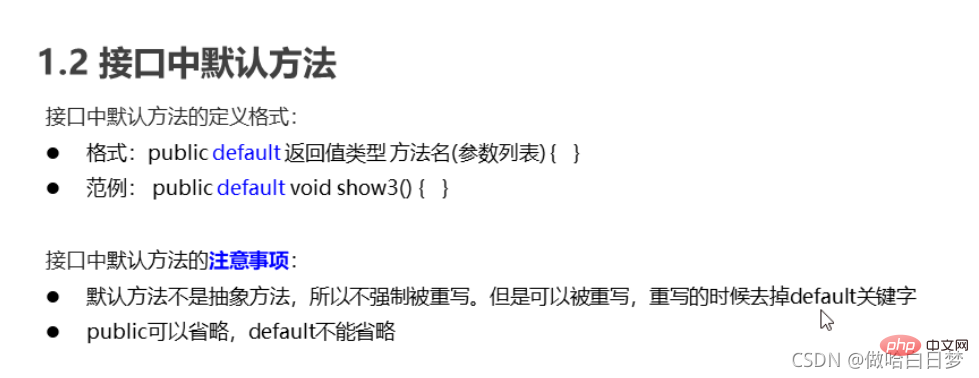
03 May 2023
1. Abstract class 1. What is an abstract class? For example, in life, we all classify dogs and cats into the same category of animals, but when we only talk about animals, we don’t know whether it is a cat, a dog, or something else. So animals are so-called abstract classes, and cats and dogs are concrete classes. Therefore, in Java, a method without a method body should be defined as an abstract class, and if a class has an abstract method, it must be an abstract class. 2. Characteristics of abstract classes: Abstract classes and abstract methods must be modified with the abstract keyword. An abstract class does not necessarily have abstract methods, but a class with abstract methods must be an abstract class. Abstract classes cannot be instantiated. If they need to be instantiated, refer to the polymorphic form and instantiate through subclasses. If a subclass inherits an abstract class, you need to rewrite all the functions in the abstract class.


Hot Tools

PHP library for dependency injection containers
PHP library for dependency injection containers
A collection of 50 excellent classic PHP algorithms
Classic PHP algorithm, learn excellent ideas and expand your thinking
Small PHP library for optimizing images
Small PHP library for optimizing images
