<?php namespace Auryn; class CachingReflector implements Reflector { const CACHE_KEY_CLASSES = 'auryn.refls.classes.'; const CACHE_KEY_CTORS = 'auryn.refls.ctors.'; const CACHE_KEY_CTOR_PARAMS = 'auryn.refls.ctor-params.'; const CACHE_KEY_FUNCS = 'auryn.refls.funcs.'; const CACHE_KEY_METHODS = 'auryn.refls.methods.'; private $reflector; private $cache; public function __construct(Reflector $reflector = null, ReflectionCache $cache = null) { $this->reflector = $reflector ?: new StandardReflector; $this->cache = $cache ?: new ReflectionCacheArray; } public function getClass($class) { $cacheKey = self::CACHE_KEY_CLASSES . strtolower($class); if (!$reflectionClass = $this->cache->fetch($cacheKey)) { $reflectionClass = new \ReflectionClass($class); $this->cache->store($cacheKey, $reflectionClass); } return $reflectionClass; }
Our idea is that when the application uses a Foo class, it will create the Foo class and call the methods of the Foo class. If this method requires a Bar class, it will create the Bar class and call the Bar class methods. This method requires a Bim class, it will create the Bim class, and then do other work. The idea of using dependency injection is that the application uses the Foo class, the Foo class needs the Bar class, and the Bar class needs the Bim class, then first create the Bim class, then create the Bar class and inject Bim, then create the Foo class, and inject the Bar class , then call the Foo method, Foo calls the Bar method, and then does other work. This is the Inversion of Control pattern. Control of dependencies is reversed to the beginning of the call chain. This way you have complete control over dependencies and control the behavior of your program by adjusting different injected objects. For example, the Foo class uses memcache, and you can use redis instead without modifying the Foo class code.
The idea behind using a dependency injection container is that if the application needs to get the Foo class, it gets the Foo class from the container, the container creates the Bim class, then creates the Bar class and injects Bim, then creates the Foo class, and injects it into the Bim class. Bar injection, the application calls the Foo method, Foo calls the Bar method, and then does other work. In short, the container is responsible for instantiation, injecting dependencies, processing dependencies, etc.
All resources on this site are contributed by netizens or reprinted by major download sites. Please check the integrity of the software yourself! All resources on this site are for learning reference only. Please do not use them for commercial purposes. Otherwise, you will be responsible for all consequences! If there is any infringement, please contact us to delete it. Contact information: admin@php.cn
Related Article
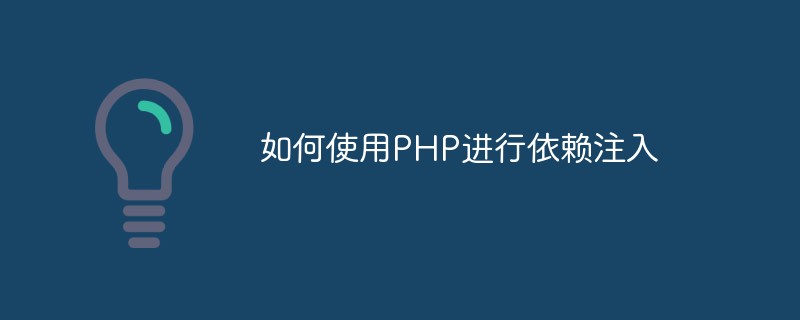
06 Jun 2023
With the development of software engineering, more and more developers focus on writing applications that are easy to maintain and scalable. Among them, dependency injection (DependencyInjection) is a very important pattern, which can achieve testability, organizeability and scalability in applications. As a well-known programming language, PHP also has its own dependency injection container and related libraries. Developers can use these tools to implement dependency injection. This article will introduce how to use PHP for dependency injection. What
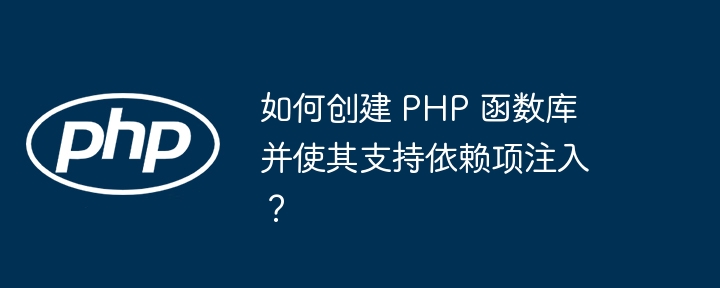
26 Apr 2024
How to create a PHP function library that supports dependency injection (DI): Create a Composer package as a function library. Implement function library functions, such as implementing a greeting function in a file. Install the PhpDI container and create a container configuration, adding the function library class to the container as a factory definition. Use libraries in code and inject dependencies, such as using containers to obtain instances of library classes. In practical applications, such as saving user data to the database, injecting database connections to improve flexibility.
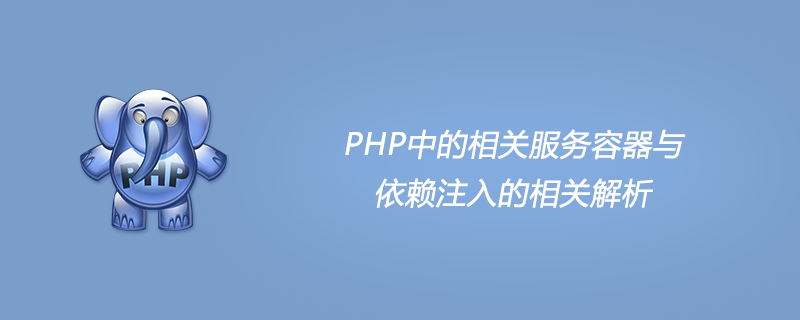
18 Jun 2019
Dependency injection: When class A needs to depend on class B, that is to say, when an object of class B needs to be instantiated in class A for use, if the functions in class B change, it will also cause the use of class B in class A. It must also be modified accordingly, resulting in high coupling between class A and class B.
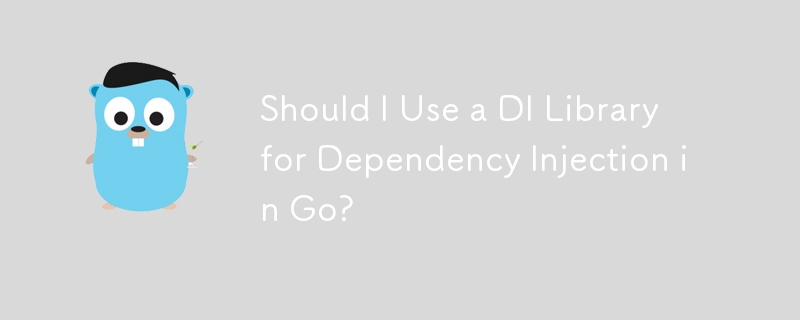
18 Dec 2024
Dependency Injection in Go: Exploring Alternative PatternsIn the code provided, the wiring of components in the main function manually passes a...
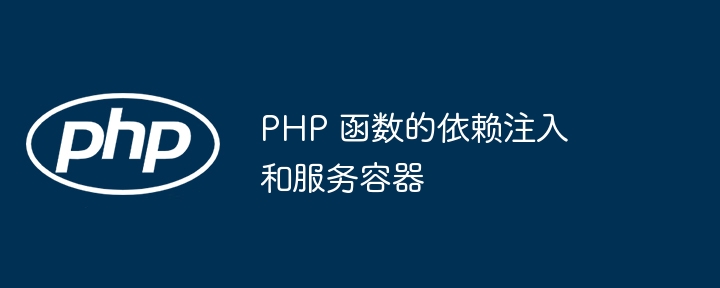
27 Apr 2024
Answer: Dependency injection and service containers in PHP help to flexibly manage dependencies and improve code testability. Dependency injection: Pass dependencies through the container to avoid direct creation within the function, improving flexibility. Service container: stores dependency instances for easy access in the program, further enhancing loose coupling. Practical case: The sample application demonstrates the practical application of dependency injection and service containers, injecting dependencies into the controller, reflecting the advantages of loose coupling.
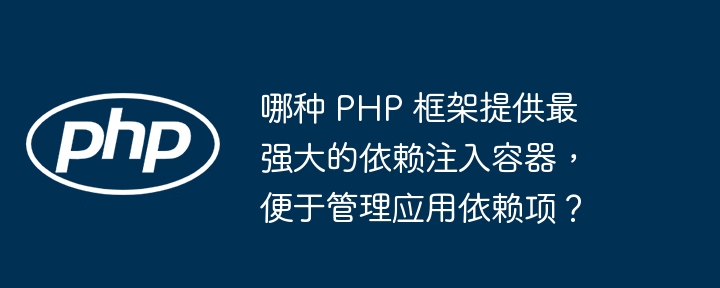
01 Jun 2024
The Laravel framework has a powerful dependency injection (DI) container that brings the following advantages: Loose coupling, improved testability and reusability Simplified dependency management, easier to change and maintain Better organization, grouping components according to type Laravel The DI container (called a "service container") provides powerful features such as automatic binding, type hints, and contracts. By injecting service instances in controllers and binding services in service providers, dependencies can be easily managed, improving code readability and maintainability.


Hot Tools
A collection of 50 excellent classic PHP algorithms
Classic PHP algorithm, learn excellent ideas and expand your thinking
Small PHP library for optimizing images
Small PHP library for optimizing images
PHP function class for winning probability algorithm
PHP function class for winning probability algorithm
